Maximizing Efficiency: The Power of Unix Utilities
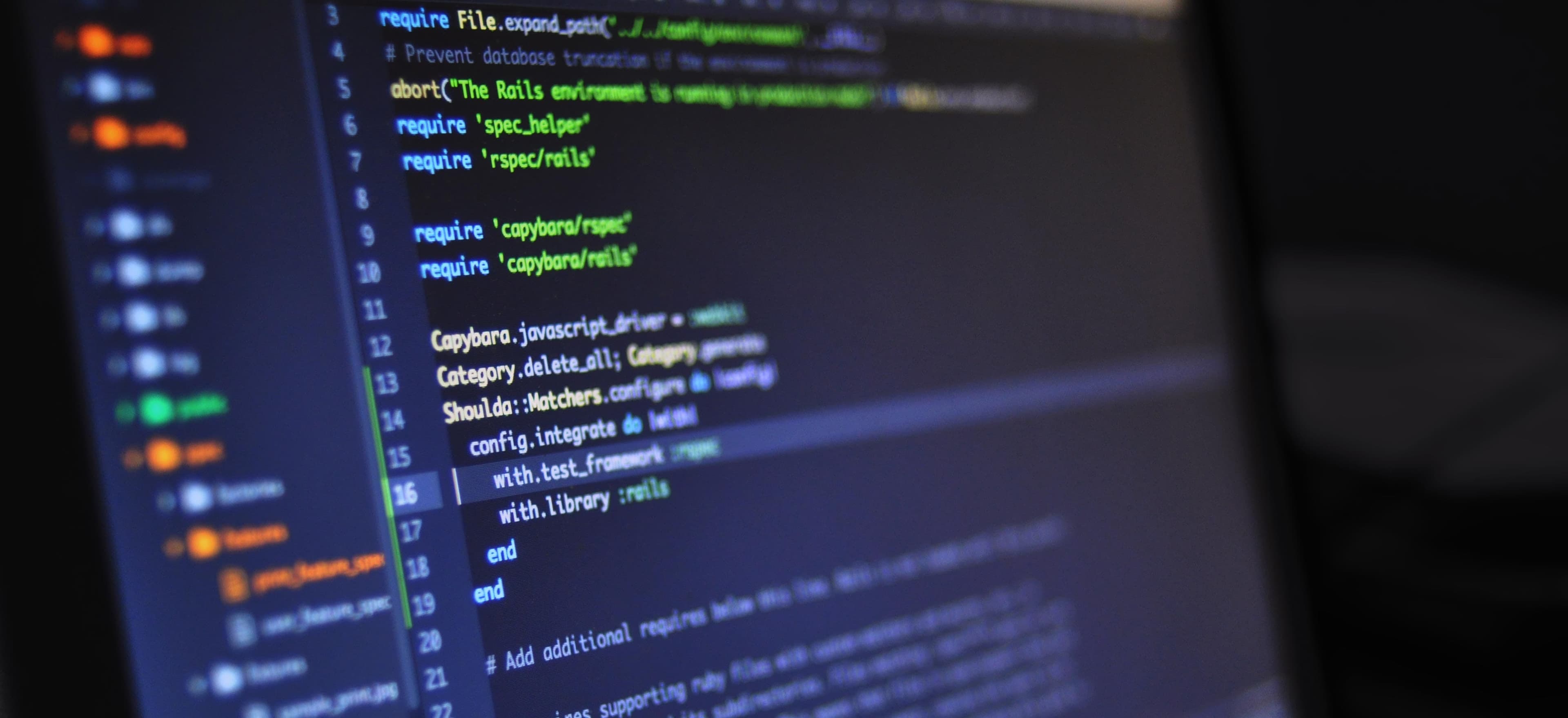
- Published on
Maximizing Efficiency: The Power of Unix Utilities
In the world of software development, efficiency is key. Whether you are developing a small personal project or working on a large-scale enterprise application, the ability to streamline your workflow is invaluable. One of the most powerful tools in a developer's arsenal is the wide array of Unix utilities available at their disposal. In this article, we'll explore some of the most essential Unix utilities and learn how they can be used to maximize efficiency in Java development.
What are Unix Utilities?
Unix utilities are a set of small, specialized command-line programs that perform specific tasks. These utilities are designed to work together seamlessly, allowing developers to combine them in powerful and creative ways. From simple text processing to complex system administration, Unix utilities offer a versatile and efficient solution to a wide range of tasks.
Using Unix Utilities in Java Development
While Java provides a rich set of libraries and tools for software development, there are times when Unix utilities can offer a more efficient and elegant solution to certain tasks. Let's explore a few scenarios where Unix utilities can be particularly useful in Java development.
Text Processing with grep
and sed
One of the most common tasks in software development is searching and manipulating text. Unix utilities like grep
and sed
are excellent tools for this purpose. For example, let's say you have a large log file and you need to find all the lines containing a specific error message. Instead of writing a custom Java program to parse the file, you can use grep
to quickly filter the relevant lines:
grep "error message" logfile.txt
Similarly, if you need to replace a particular string in multiple files, sed
can come to the rescue:
sed -i 's/old_string/new_string/g' file1.txt file2.txt
Streamlining File Operations with find
and xargs
File operations are a fundamental aspect of software development. Unix utilities like find
and xargs
can simplify and automate various file-related tasks. For instance, if you need to find all Java source files in a directory and run a specific command on each file, you can combine find
and xargs
to achieve this with a single line:
find . -name "*.java" | xargs javac
This command finds all Java files in the current directory and compiles each of them using javac
, saving you from writing a complex shell script or manually compiling each file.
Analyzing Performance with top
and ps
Monitoring performance is crucial for identifying potential bottlenecks in a Java application. Unix utilities such as top
and ps
provide insight into system resource usage. For instance, you can use top
to display real-time information about CPU and memory usage:
top
Similarly, ps
can be used to list all Java processes running on the system, along with their details:
ps aux | grep java
By leveraging these utilities, you can quickly identify resource-intensive processes and optimize your Java application's performance.
Integrating Unix Utilities into Java Programs
While Unix utilities are powerful on their own, they can be even more impactful when integrated into Java programs. Below is an example of a Java program that utilizes the grep
utility to search for a specific pattern in a file:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class GrepExample {
public static void main(String[] args) {
try {
Process process = Runtime.getRuntime().exec("grep error message logfile.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, we use Java's Runtime
class to execute the grep
command and capture its output. This demonstrates how Unix utilities can be seamlessly integrated into Java programs to enhance their functionality.
The Closing Argument
Unix utilities offer a powerful and efficient toolkit for Java developers. By harnessing the capabilities of these command-line programs, developers can streamline their workflows, perform complex operations with ease, and gain valuable insights into system performance. Whether used directly from the command line or integrated into Java programs, Unix utilities are an indispensable asset for maximizing efficiency in Java development.
In conclusion, mastering Unix utilities can significantly enhance a developer's productivity and empower them to tackle challenging tasks with elegance and ease.
By incorporating Unix utilities into your Java development workflow, you can unlock a new level of efficiency and effectiveness, ultimately leading to more robust and maintainable software products.
Embrace the power of Unix utilities and elevate your Java development experience today!