Optimizing Data Access Layer Efficiency
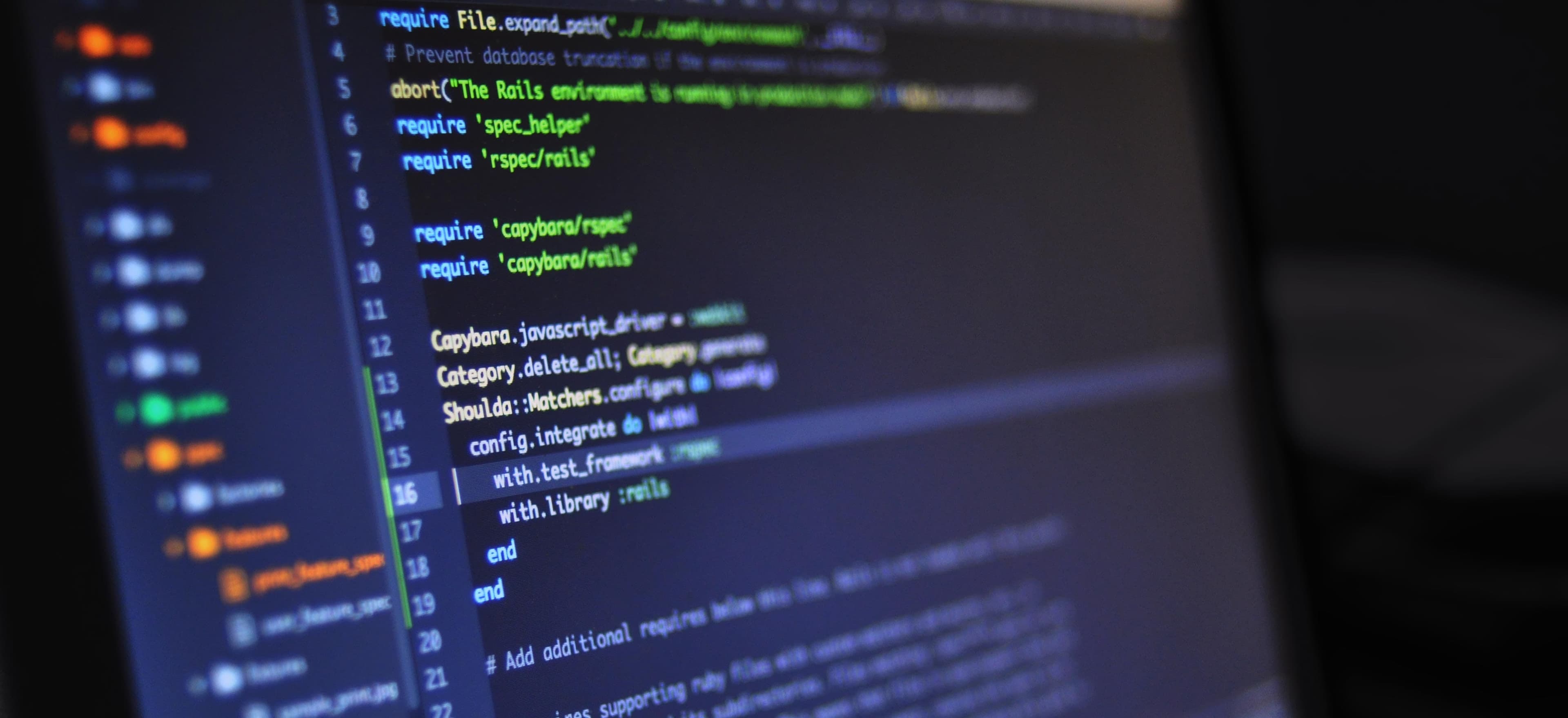
- Published on
Optimizing Data Access Layer Efficiency
In the realm of enterprise application development, it's imperative to optimize the performance of the Data Access Layer (DAL) to ensure minimal latency, optimal resource utilization, and scalable data retrieval. Java, with its rich ecosystem and robust libraries, offers a plethora of tools and techniques to enhance the efficiency of the Data Access Layer. In this blog post, we will delve into various strategies and best practices to optimize the Data Access Layer in Java applications.
Choosing the Right Data Access Technology
When optimizing the Data Access Layer, the choice of data access technology plays a pivotal role. Java offers several options, such as JDBC, JPA (Java Persistence API), Hibernate, and Spring Data. Each technology has its strengths and weaknesses, and selecting the most suitable one for your application is crucial for performance optimization.
JDBC
Using JDBC for low-level database interactions provides fine-grained control over SQL queries and can be highly efficient for custom-tailored data access logic. However, it necessitates manual management of resources and can lead to boilerplate code.
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users")) {
try (ResultSet resultSet = statement.executeQuery()) {
while (resultSet.next()) {
// Process the results
}
}
} catch (SQLException ex) {
// Handle the exception
}
JPA and Hibernate
JPA, with providers like Hibernate, abstracts the underlying database interactions and provides ORM (Object-Relational Mapping) capabilities, reducing the need for boilerplate code and enabling a more object-oriented approach. However, it's essential to understand the generated SQL queries and the performance implications of the ORM mappings.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// Other fields and methods
}
Spring Data
Spring Data provides a higher level of abstraction on top of JPA and Hibernate, simplifying the data access code with its repository interfaces and query derivation mechanisms. It fosters rapid development and reduces the verbosity of data access code.
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByUsername(String username);
}
Connection Pooling
In a high-traffic enterprise application, managing database connections efficiently is paramount. Connection pooling, offered by libraries like HikariCP and Apache DBCP, can significantly improve the performance of the Data Access Layer by recycling connections and minimizing the overhead of establishing new connections for each database operation.
HikariConfig config = new HikariConfig();
config.setJdbcUrl(url);
config.setUsername(user);
config.setPassword(password);
config.setMaximumPoolSize(10);
DataSource dataSource = new HikariDataSource(config);
Query Optimization
Efficient database queries are instrumental in optimizing the Data Access Layer. Techniques such as indexing relevant columns, minimizing the number of executed queries, and utilizing pagination can enhance the overall query performance.
Indexing
Identifying and indexing the appropriate columns in database tables can accelerate query execution by facilitating rapid data retrieval. It's imperative to analyze query patterns and use indexing judiciously to avoid unnecessary overhead.
CREATE INDEX idx_username ON users(username);
Query Batching
Batching multiple operations into a single database round-trip minimizes latency and reduces the overhead of network communication. This is particularly beneficial when dealing with bulk data operations.
entityManager.unwrap(Session.class).doWork(connection -> {
try (Statement statement = connection.createStatement()) {
// Execute multiple statements
}
});
Pagination
When dealing with large result sets, incorporating pagination in queries ensures that only a subset of the data is fetched at once, preventing excessive memory consumption and enhancing query responsiveness.
List<User> users = entityManager.createQuery("SELECT u FROM User u", User.class)
.setFirstResult(0)
.setMaxResults(10)
.getResultList();
Caching
Employing caching mechanisms can significantly reduce the database load and enhance the responsiveness of the Data Access Layer. Libraries like Ehcache and Redis offer robust caching solutions, allowing frequent query results to be cached for subsequent retrievals.
@Cacheable("users")
public List<User> findAllUsers() {
// Retrieve and cache the users
}
Asynchronous Data Access
Asynchronous data access can prevent blocking operations, especially in scenarios where concurrent access to the Data Access Layer is prevalent. Leveraging CompletableFuture or reactive programming with libraries like Project Reactor or RxJava can improve the responsiveness of data retrieval operations.
CompletableFuture<List<User>> futureUsers = CompletableFuture.supplyAsync(() -> userRepository.findAll());
Performance Monitoring and Tuning
Continuous monitoring of Data Access Layer performance, utilizing tools like JProfiler and Java Mission Control, is essential for identifying bottlenecks and fine-tuning data access operations. Profiling database interactions and optimizing the most frequently executed queries can yield substantial performance improvements.
Final Thoughts
Optimizing the Data Access Layer in Java applications is a multifaceted endeavor, encompassing the selection of appropriate data access technologies, efficient query optimization, resource management, and the incorporation of caching and asynchronous patterns. By adhering to best practices and leveraging the rich ecosystem of Java libraries and tools, developers can ensure the efficiency and scalability of the Data Access Layer, laying a robust foundation for high-performance enterprise applications.
In the dynamic landscape of enterprise application development, the quest for data access layer optimization is perpetual, and Java, with its versatility and extensibility, equips developers with an array of tools to navigate this terrain with finesse.