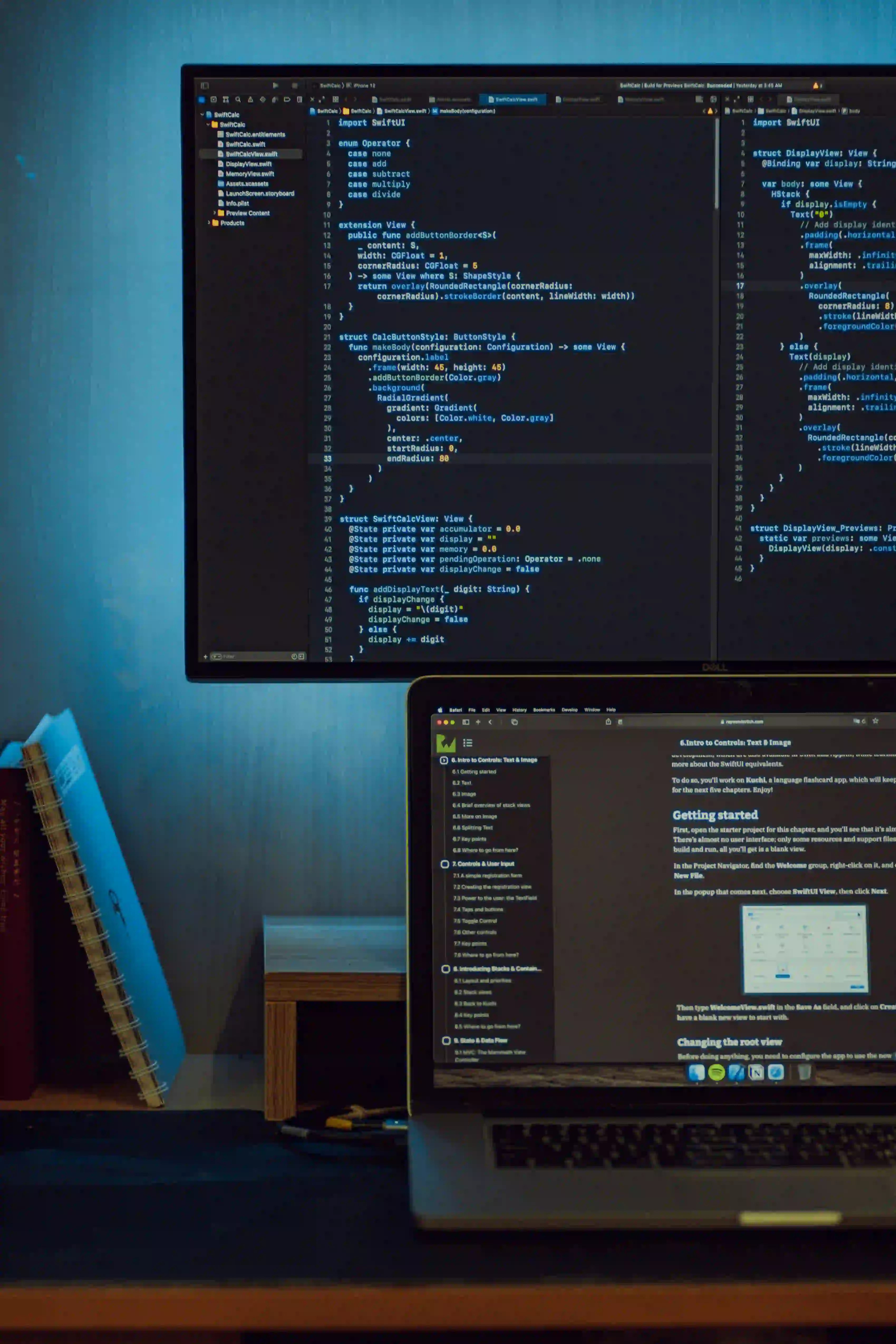
Understanding Zero Trust Security Model in Java
In today's digital landscape, the rise in cyber threats calls for robust security measures. One such approach gaining momentum is the Zero Trust security model. Originally introduced by Forrester Research, Zero Trust challenges the traditional security model, which relied on perimeter-based defenses. This blog post explores the concept of Zero Trust and how it can be implemented in Java to enhance the security of your applications.
What is Zero Trust?
Zero Trust is a security concept based on the principle of "never trust, always verify." Unlike traditional security models that assume trust once inside the network perimeter, Zero Trust advocates for a more rigorous approach. It requires continuous verification of the trustworthiness of users, devices, and applications, regardless of their location (inside or outside the corporate network).
The core tenets of Zero Trust can be summarized as follows:
- Verify Explicitly: All access requests are verified before granting entry.
- Least Privilege Access: Access is granted based on the principle of least privilege, ensuring that users have only the necessary access rights.
- Assume Breach: Instead of assuming that everything inside the network is safe, Zero Trust operates under the assumption that the network is already compromised.
Implementing Zero Trust in Java
Role of Identity and Access Management (IAM)
In a Java application, implementing Zero Trust begins with a robust Identity and Access Management (IAM) system. IAM solutions such as Keycloak provide capabilities for authentication, authorization, and user management. By integrating Keycloak with your Java applications, you can enforce Zero Trust principles by ensuring that every access request is explicitly verified and authenticated.
// Sample code integrating Keycloak for authentication and authorization
KeycloakSecurityContext session = (KeycloakSecurityContext) request.getAttribute(KeycloakSecurityContext.class.getName());
// Verify user's access rights
if (session.getToken().getRealmAccess().isUserInRole("admin")) {
// Grant access to admin resources
} else {
// Access denied
}
In the above snippet, the user's access rights are checked explicitly before granting access to admin resources. This aligns with the Zero Trust principle of least privilege access.
Microservices and API Security
Java applications, particularly those following microservices architecture, can benefit from Zero Trust by implementing robust API security mechanisms. Technologies such as OAuth 2.0 and JSON Web Tokens (JWT) play a pivotal role in ensuring that only authenticated and authorized entities can access the microservices.
// Sample code for validating JWT in a microservice
String token = // extract token from request
Claims claims = Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(token)
.getBody();
// Verify user's identity and role
if (claims.getSubject().equals("user123") && claims.get("role").equals("admin")) {
// Grant access to the microservice
} else {
// Access denied
}
In the code snippet above, the JWT is validated, and the user's identity and role are verified before granting access to the microservice. This aligns with the Zero Trust principle of continuously verifying the trustworthiness of users and applications.
Secure Communication
Another key aspect of Zero Trust is ensuring secure communication between services and clients. In Java, this can be achieved through the use of Transport Layer Security (TLS) to encrypt the data exchanged over the network. Additionally, implementing mutual TLS authentication further strengthens the Zero Trust model by requiring both the client and the server to present certificates to establish trust.
// Sample code for enabling mutual TLS in a Java web application
SSLContext sslContext = SSLContext.getInstance("TLS");
KeyManagerFactory keyManagerFactory = // initialize KeyManagerFactory
TrustManagerFactory trustManagerFactory = // initialize TrustManagerFactory
sslContext.init(keyManagerFactory.getKeyManagers(), trustManagerFactory.getTrustManagers(), new SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(sslContext.getSocketFactory());
The above code snippet demonstrates the use of mutual TLS to establish secure communication in a Java web application, aligning with the Zero Trust principle of assuming breach by encrypting all communication, even within the network perimeter.
Closing Remarks
In conclusion, Zero Trust presents a paradigm shift in the way security is approached in the digital age. By implementing Zero Trust principles in Java applications through robust IAM, API security, and secure communication, organizations can significantly enhance their security posture. This proactive approach, coupled with continuous verification and least privilege access, can help mitigate the ever-evolving cyber threats in today's interconnected world.
Embracing the Zero Trust model in Java is not merely a trend but a strategic imperative in safeguarding sensitive data and ensuring trust in the digital ecosystem. As threats continue to evolve, the Zero Trust model stands as a beacon of security, guiding organizations towards a safer, more resilient future.
To delve deeper into the topic of Zero Trust and its application in Java, feel free to explore the following resources:
Implementing the Zero Trust model in Java is not without its challenges, but it is undoubtedly a step in the right direction toward a more secure digital future.