Optimizing Java Code Style for Consistency
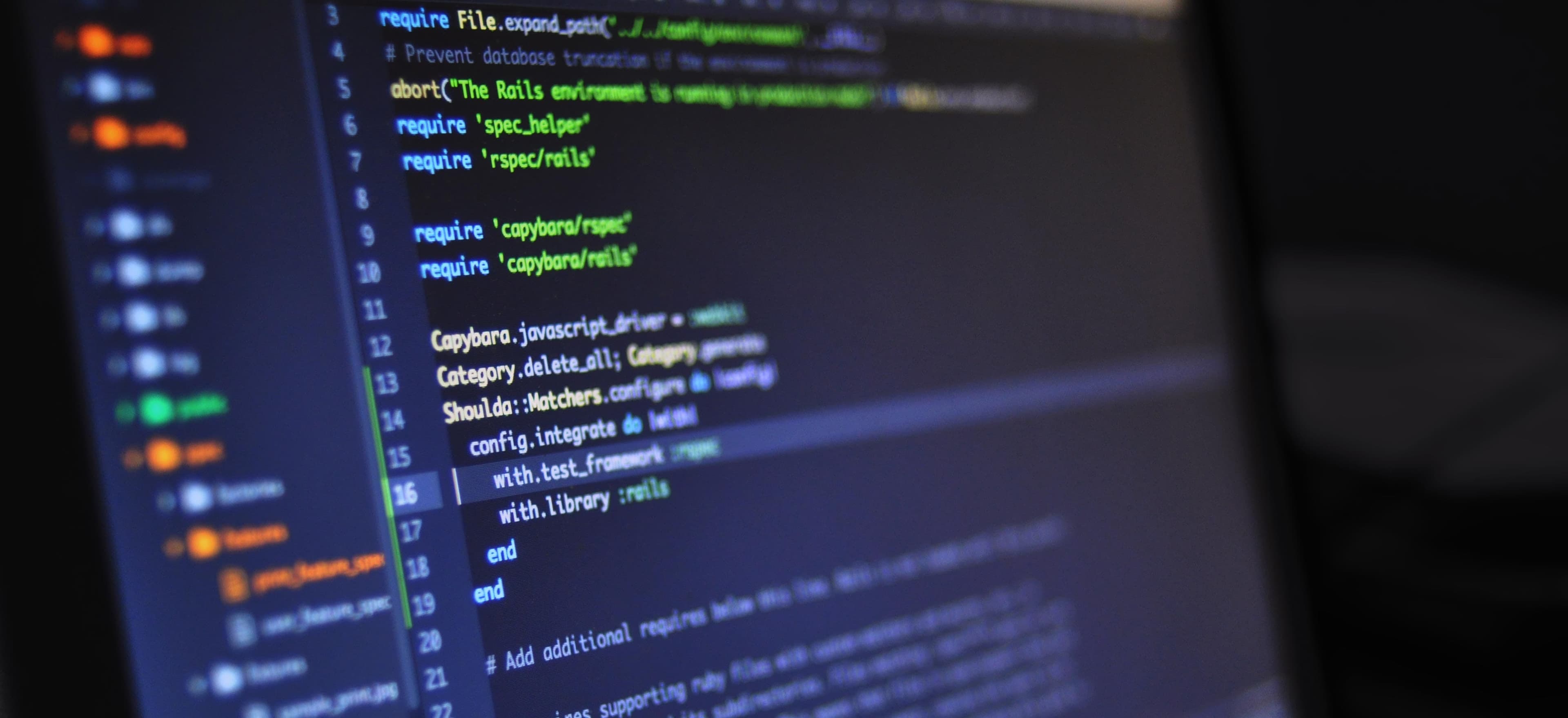
- Published on
Optimizing Java Code Style for Consistency
When it comes to Java programming, maintaining a consistent code style is crucial for readability, maintainability, and collaboration. It ensures that all team members can easily understand and work with each other's code. In this blog post, we'll discuss the importance of code style consistency in Java, explore the best practices for achieving it, and demonstrate how tools such as Checkstyle and SonarQube can help enforce and maintain a consistent code style.
Importance of Code Style Consistency
Consistency in code style makes code easier to read, understand, and maintain. It reduces cognitive overhead for developers, allowing them to focus on the logic and functionality of the code rather than decoding inconsistent formatting. Consistent code style also leads to better collaboration within a team, as it creates a unified and predictable codebase. Additionally, it contributes to the overall quality of the code, making it easier to identify and fix issues through systematic approaches.
Best Practices for Achieving Code Style Consistency
1. Follow Official Style Guidelines
Adhering to official style guidelines, such as those provided by Oracle for Java, sets a strong foundation for code consistency. These guidelines cover aspects such as naming conventions, indentation, brace placement, and commenting standards. By following established conventions, developers can ensure that their code aligns with industry best practices and is readily familiar to other Java developers.
2. Utilize IDE Code Formatting
Modern integrated development environments (IDEs), such as IntelliJ IDEA and Eclipse, offer powerful code formatting features. By configuring these tools to apply a consistent code style automatically, developers can enforce adherence to the established guidelines. IDEs also provide options to customize formatting rules, allowing teams to tailor the code style to their specific preferences while maintaining consistency across the project.
3. Leverage Code Analysis Tools
Integrating code analysis tools like Checkstyle and SonarQube into the development workflow can provide automated checks for adherence to code style guidelines. These tools can flag deviations from the established code style, allowing for quick identification and resolution of stylistic inconsistencies. Furthermore, they enable the enforcement of code style rules directly within the continuous integration process, ensuring that all code contributions meet the defined standards.
Using Checkstyle for Code Style Enforcement
Checkstyle is a popular tool for enforcing coding standards and style guidelines in Java. Let's take a look at how Checkstyle can be integrated into a Java project to achieve consistent code style.
Step 1: Add Checkstyle Configuration
First, create a Checkstyle configuration file (e.g., checkstyle.xml
) that defines the desired coding standards. This configuration file can specify rules related to indentation, Javadoc comments, naming conventions, and more. By tailoring the rules to reflect the team's agreed-upon code style, Checkstyle can enforce consistency throughout the codebase.
<module name="Checker">
<module name="TreeWalker">
<module name="Indentation">
<property name="basicOffset" value="4"/>
<property name="braceAdjustment" value="0"/>
</module>
<module name="JavadocStyle"/>
<module name="NamingConvention">
<property name="format" value="pattern"/>
<property name="format" value="^[a-z][a-zA-Z0-9]*$"/>
<property name="format" value="^[A-Z][A-Za-z0-9]*$"/>
</module>
</module>
</module>
In the example above, the Checkstyle configuration includes modules for checking indentation, Javadoc style, and naming conventions. The Indentation
module ensures that code is consistently indented with a basic offset of 4 spaces, while the JavadocStyle
module checks for proper formatting of Javadoc comments. The NamingConvention
module enforces naming rules for classes, methods, and variables.
Step 2: Integrate Checkstyle with Build Process
Integrate Checkstyle into the build process, such as using Maven or Gradle, to execute the style checks automatically. By incorporating Checkstyle as part of the build pipeline, developers receive instant feedback on code style violations, allowing them to address issues early in the development cycle.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version>
<executions>
<execution>
<id>validate</id>
<phase>validate</phase>
<configuration>
<configLocation>checkstyle.xml</configLocation>
<consoleOutput>true</consoleOutput>
<failsOnError>true</failsOnError>
</configuration>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
In the Maven configuration above, the maven-checkstyle-plugin
is included with an execution bound to the validate
phase. This ensures that the Checkstyle checks are run at an early stage of the build process, allowing for quick identification of code style violations.
Step 3: Review and Resolve Checkstyle Findings
After integrating Checkstyle into the project's build process, developers can review the Checkstyle findings and address any reported violations. This iterative process supports the continuous improvement of code style, ultimately leading to a more consistent and readable codebase.
Leveraging SonarQube for Code Quality and Style Analysis
SonarQube provides comprehensive capabilities for code quality and style analysis, offering an integrated approach to maintaining code consistency alongside other aspects of code quality. Let's delve into how SonarQube can be utilized for code style analysis in a Java project.
Step 1: Set Up SonarQube Integration
Begin by setting up SonarQube integration within the project's build environment. This may involve configuring the project to track code quality metrics and perform periodic analyses using SonarQube's static analysis features.
Step 2: Define Quality Profiles for Code Style
Within SonarQube, define quality profiles that encapsulate the desired code style rules. These profiles can encompass checks for naming conventions, formatting consistency, commenting standards, and other aspects of code style. By tailoring the quality profiles to align with the project's code style guidelines, SonarQube can enforce and monitor consistency across the codebase.
Step 3: Continuous Monitoring of Code Style
Integrate SonarQube analysis into the continuous integration pipeline to enable regular assessment of code style. By leveraging SonarQube's reporting capabilities, teams can gain insights into code style violations, track improvement over time, and ensure that the codebase adheres to the defined standards.
Key Takeaways
Consistent code style is essential for fostering readability, maintainability, and collaboration within Java projects. By adhering to official style guidelines, utilizing IDE code formatting, and leveraging tools such as Checkstyle and SonarQube, developers can enforce and maintain a consistent code style. These practices contribute to the overall quality of the codebase, ultimately leading to more efficient development processes and higher-quality software.
Enabling and enforcing consistent code style is not only a best practice, but it is also a hallmark of a professional approach to software development. By adopting the strategies and tools discussed in this post, Java development teams can elevate their code quality and enhance the overall development experience.
Remember, consistency in code style is not just about aesthetics—it's about fostering a collaborative and efficient development environment where code is not only functional but also delightful to work with.