Developing Scalable and Reactive Applications
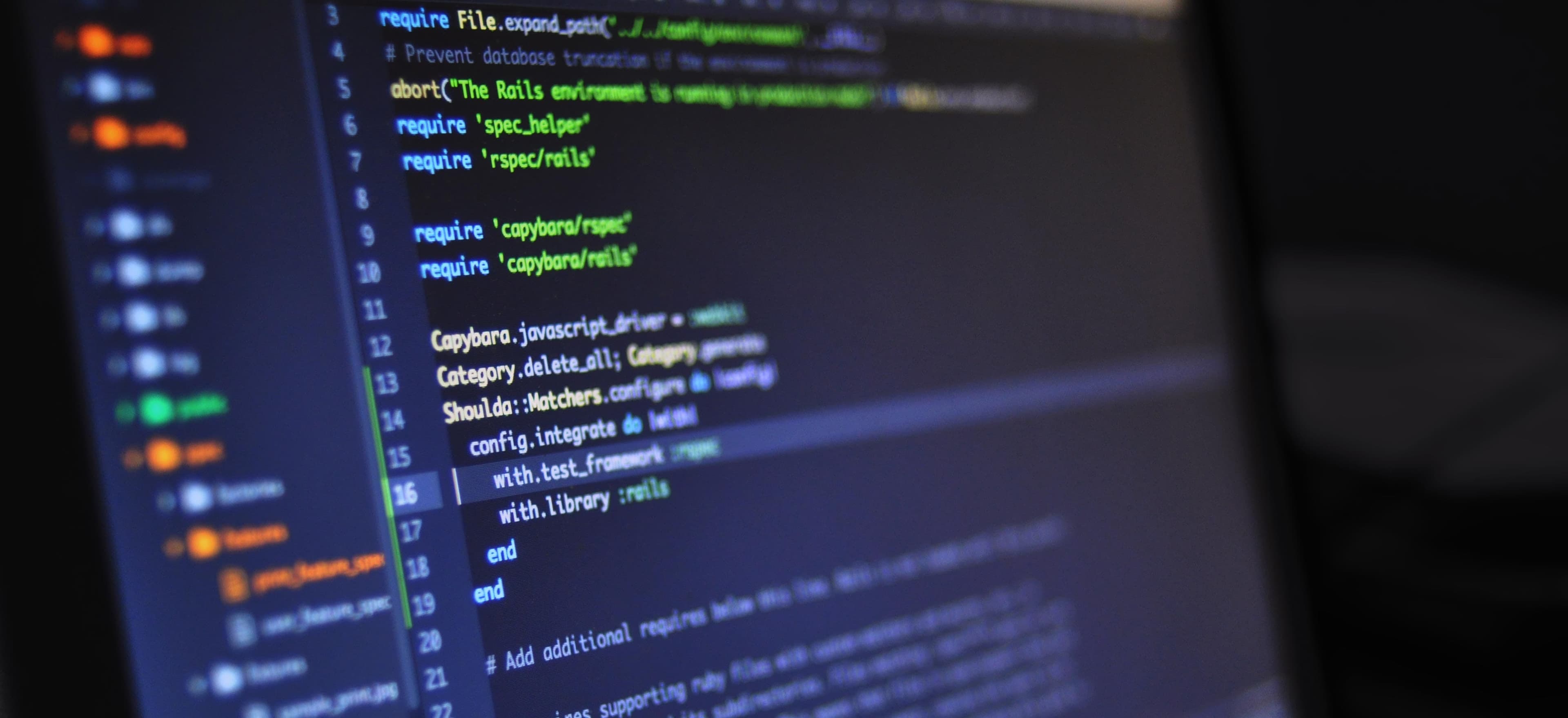
- Published on
Building Scalable and Reactive Applications with Java
In today's fast-paced and demanding technological landscape, the need for responsive and scalable applications is more critical than ever. As user bases grow and the amount of data processed increases, traditional, rigid applications can quickly become bottlenecks.
This is where reactive programming comes into play. It allows developers to build applications that are responsive, resilient, and message-driven. Java, in particular, has evolved to embrace reactive programming, enabling developers to create scalable applications that can handle a large number of concurrent users and data streams.
The Reactive Manifesto
At the heart of reactive programming lies the Reactive Manifesto, which outlines four key principles:
- Responsive: The system responds in a timely manner.
- Resilient: The system stays responsive in the face of failure.
- Elastic: The system stays responsive under varying workloads.
- Message-Driven: The system is built on asynchronous message passing.
Leveraging Java for Reactive Programming
Java, a language known for its robustness and versatility, has embraced reactive programming through libraries and frameworks. Two popular choices for building reactive applications in Java are Vert.x and Reactor.
Vert.x
Vert.x is a toolkit for building reactive applications on the Java Virtual Machine (JVM). It provides a powerful event-driven programming model and supports various languages, including Java, Kotlin, and Groovy.
Let's take a look at a simple example of using Vert.x to create a reactive HTTP server:
import io.vertx.core.Vertx;
public class Main {
public static void main(String[] args) {
Vertx vertx = Vertx.vertx();
vertx.createHttpServer()
.requestHandler(req -> {
req.response().end("Hello, Reactive World!");
})
.listen(8080);
}
}
In this example, we create an HTTP server that responds with "Hello, Reactive World!" to any incoming requests. The server utilizes Vert.x's event-driven model, making it scalable and non-blocking.
Reactor
Reactor is a foundational library for building reactive fast-data applications on the JVM. It provides the building blocks for creating reactive sequences and composing them in a declarative manner.
Here's a simple example of using Reactor's Flux
to create a stream of data:
import reactor.core.publisher.Flux;
public class Main {
public static void main(String[] args) {
Flux<Integer> numbers = Flux.just(1, 2, 3, 4, 5);
numbers.subscribe(System.out::println);
}
}
In this example, we create a Flux that emits the numbers 1 to 5 and then subscribe to it to print each emitted number. Reactor's declarative approach allows for easy composition and manipulation of reactive sequences.
Benefits of Reactive Programming in Java
Reactive programming in Java offers several key benefits:
- Scalability: Reactive applications can handle a large number of concurrent users and data streams, making them well-suited for modern, data-intensive use cases.
- Resilience: Reactive systems are designed to stay responsive in the face of failure, making them more robust and reliable.
- Asynchronous and Non-blocking: Reactive applications leverage asynchronous and non-blocking patterns, leading to improved resource utilization and responsiveness.
- Composability: The declarative nature of reactive programming allows for easy composition and manipulation of data streams, leading to more maintainable and readable code.
Best Practices for Building Reactive Applications
When building reactive applications in Java, certain best practices should be followed to ensure optimal performance and maintainability:
- Use Backpressure: When dealing with potentially infinite streams of data, it's essential to handle backpressure to prevent overwhelming the system.
- Avoid Blocking Calls: Reactive applications should minimize or entirely avoid blocking calls to maintain responsiveness.
- Choose the Right Data Structures: Use data structures that are optimized for asynchronous and non-blocking operations, such as those provided by Vert.x and Reactor.
- Understand Concurrency: Gain a deep understanding of concurrency in Java to effectively manage concurrent data streams and prevent race conditions.
- Monitor and Tune: Regularly monitor and tune your reactive applications to ensure optimal performance and responsiveness.
By adhering to these best practices, developers can harness the full potential of reactive programming in Java and build high-performing, scalable applications.
Bringing It All Together
In conclusion, the shift towards reactive programming in Java has paved the way for building scalable, responsive, and resilient applications. Leveraging frameworks like Vert.x and libraries like Reactor empowers developers to embrace the reactive manifesto and create systems that are well-equipped to handle the demands of modern, data-intensive use cases.
As the industry continues to evolve, mastering reactive programming in Java will be a valuable skill for developers looking to stay at the forefront of application development.
By following best practices and continuously refining their expertise, developers can unlock the full potential of reactive programming and ensure their applications meet the demands of today's dynamic and data-driven world.