When to Use Mock Objects in Unit Testing
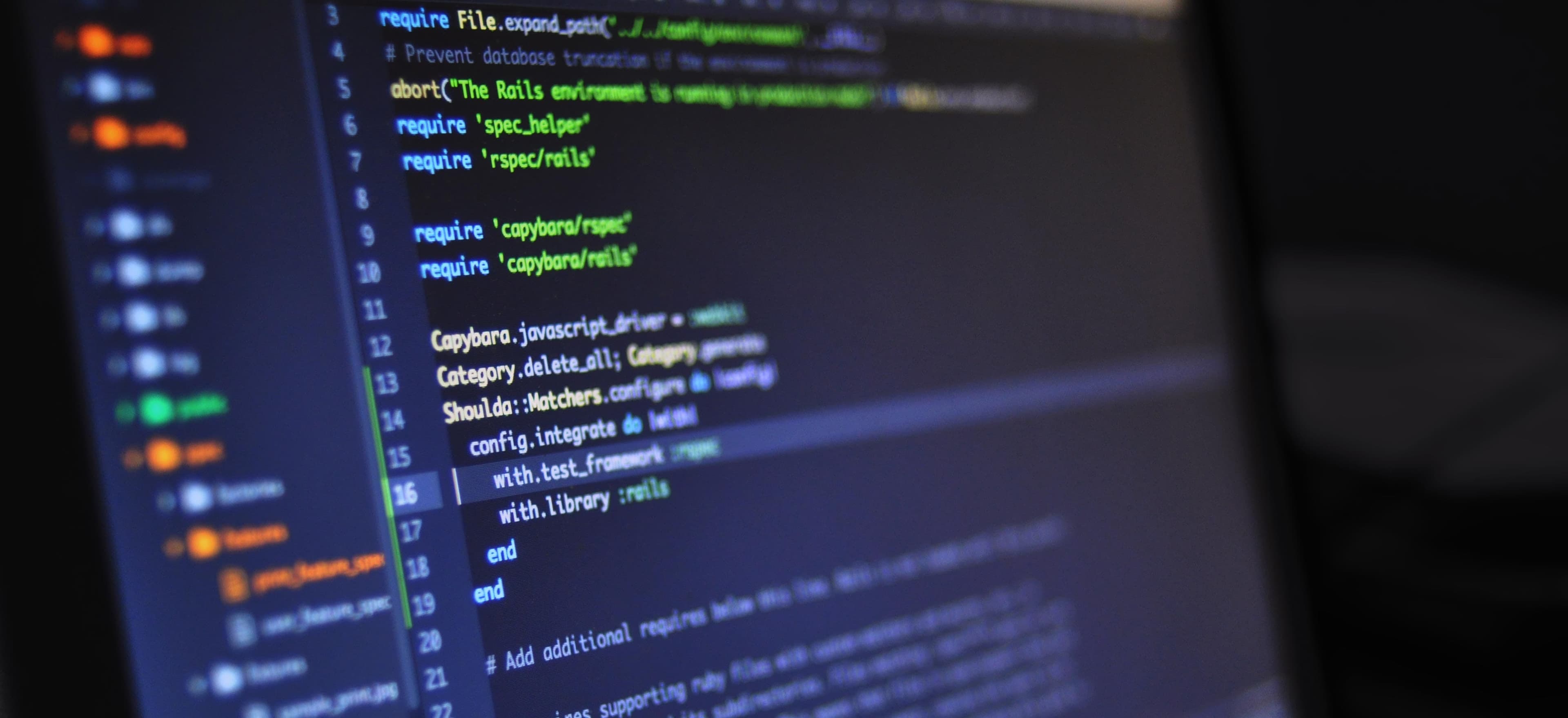
- Published on
When to Use Mock Objects in Unit Testing
In the world of Java unit testing, mock objects play a crucial role in isolating the code under test and verifying its behavior. But when should you use mock objects? What benefits do they offer, and how can they improve the effectiveness of your unit tests? Let's delve into the realm of mock objects and explore their applications in Java unit testing.
What are Mock Objects?
Mock objects are simulated objects that mimic the behavior of real objects in controlled ways. In the context of unit testing, mock objects are used to mimic the behavior of complex, real objects that are impractical to include in the unit test scenario. By creating these simulated objects, developers can verify how the code under test interacts with them while keeping the test environment isolated and predictable.
Use Cases for Mock Objects
Testing Collaboration Between Classes
When a class under test collaborates with other classes, using real instances of these collaborators can introduce complexity and potential instability to the unit tests. Mock objects allow you to simulate these interactions, providing controlled responses to method calls and enabling you to focus solely on the behavior of the class under test.
Simulating External Dependencies
In real-world applications, classes often rely on external services or components, such as databases, web APIs, or network resources. Integrating these external dependencies into unit tests can be cumbersome, slow, and prone to errors. Mock objects serve as stand-ins for these external dependencies, ensuring that the focus remains on the unit of code being tested rather than on the intricacies of external systems.
Handling Unpredictable or Unstable Behavior
Certain classes may exhibit unpredictable behavior due to factors such as randomness, time, or external state. Mock objects provide a predictable and stable alternative, allowing you to simulate various scenarios and edge cases that would be difficult to reproduce with real instances.
Benefits of Using Mock Objects
Isolation of Code Under Test
Mock objects help isolate the code under test by replacing its dependencies with predictable behavior. This isolation ensures that any failures or issues in the test are directly attributable to the code being tested, rather than to external factors.
Improved Test Performance
By replacing heavyweight or slow external dependencies with lightweight and fast mock objects, unit tests can run more efficiently, leading to shorter feedback loops during development.
Enhanced Test Control and Predictability
Mock objects empower developers to define specific behaviors and responses, leading to more controlled and predictable test scenarios. This control allows for thorough testing of edge cases and exceptional conditions.
Facilitates Test-Driven Development (TDD)
Mock objects align well with the principles of Test-Driven Development (TDD) by enabling developers to write tests that drive the design of the code. They allow for the creation of test cases before the actual implementation, guiding the development process.
Example Usage of Mock Objects in Java
Let's dive into a Java code example to illustrate the use of mock objects in unit testing. In this example, we'll use the popular mocking framework Mockito to create and utilize mock objects for testing a service class.
Assume we have a simple service interface UserService
with a method to retrieve a user by their ID:
public interface UserService {
User getUserById(long userId);
}
Now, we have a class UserServiceImpl
that depends on the UserService
interface:
public class UserServiceImpl {
private UserService userService;
public UserServiceImpl(UserService userService) {
this.userService = userService;
}
public String getUserNameById(long userId) {
User user = userService.getUserById(userId);
return user.getName();
}
}
To test the getUserNameById
method in UserServiceImpl
, we can use Mockito to create a mock object of the UserService
interface.
Here's how we can do that:
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.*;
@RunWith(MockitoJUnitRunner.class)
public class UserServiceImplTest {
@Mock
private UserService userServiceMock;
@InjectMocks
private UserServiceImpl userServiceImpl;
@Test
public void testGetUserNameById() {
// Arrange
long userId = 123L;
User mockUser = new User(userId, "John Doe");
when(userServiceMock.getUserById(userId)).thenReturn(mockUser);
// Act
String userName = userServiceImpl.getUserNameById(userId);
// Assert
assertEquals("John Doe", userName);
verify(userServiceMock).getUserById(userId);
}
}
In this example, we use Mockito's @Mock
annotation to create a mock object for the UserService
interface and the @InjectMocks
annotation to inject the mock into the UserServiceImpl
instance. We then proceed to define the behavior of the mock object using Mockito's when
and thenReturn
methods and verify that the expected interactions occur using verify
.
The Last Word
Mock objects are valuable tools in Java unit testing, providing a way to isolate code under test, simulate dependencies, and enhance test control and predictability. By understanding when and how to use mock objects effectively, developers can write more robust unit tests that accurately verify the behavior of their code. When used judiciously, mock objects contribute to the overall quality and reliability of Java applications.
In summary, the strategic application of mock objects in unit testing leads to more resilient, maintainable, and testable codebases in Java development. Embrace the power of mock objects to take your unit testing practices to the next level.