Reducing Code Size: Lombok's Impact on Java Compilation
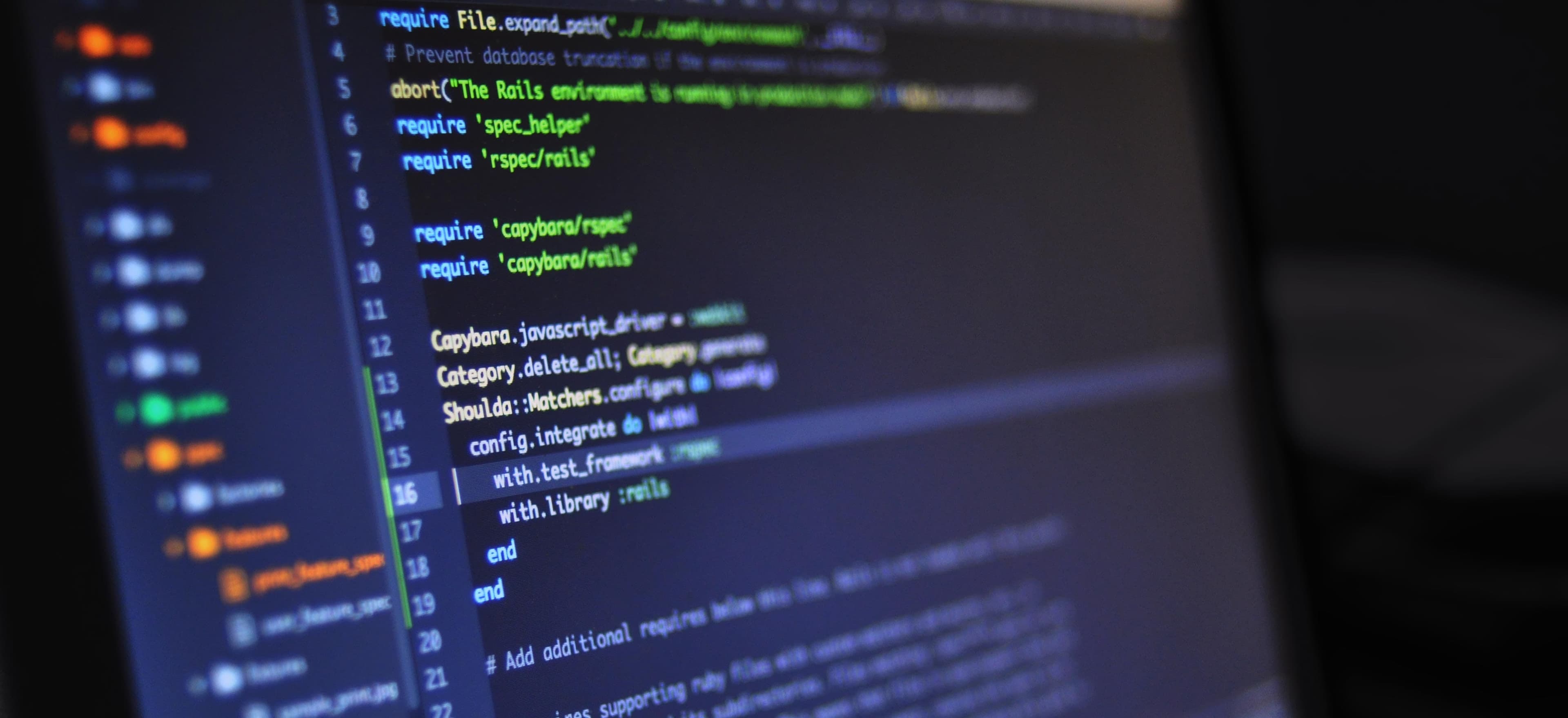
- Published on
Reducing Code Size: Lombok's Impact on Java Compilation
In the world of Java development, code verbosity is a common issue that developers frequently encounter. This verbosity often leads to larger and more complex codebases, which can make maintenance and collaboration more challenging. However, there are tools and libraries available to help streamline and simplify Java code. One such tool is Project Lombok, a popular library that aims to reduce boilerplate code and improve code readability.
In this article, we will explore how Project Lombok can impact Java compilation, reduce code size, and improve code maintainability. We will cover the basics of Project Lombok, its key features, and how it can be integrated into Java projects to enhance productivity and code quality.
Understanding Project Lombok
What is Project Lombok?
Project Lombok is a Java library that helps reduce the verbosity of Java code by automatically generating common boilerplate code, such as getters, setters, constructors, and more. It achieves this through the use of annotations, allowing developers to write more concise and readable code without sacrificing functionality.
One of the primary goals of Lombok is to address the common issue of writing repetitive, boilerplate code in Java classes. By leveraging the power of annotations, Lombok effectively reduces the need for writing and maintaining boilerplate code, resulting in cleaner and more maintainable codebases.
Key Features of Project Lombok
Project Lombok provides a wide range of features to simplify Java code, some of which include:
-
Automatic Getter and Setter Generation: With Lombok, you can annotate fields in your Java classes to automatically generate getter and setter methods, reducing the need to write these methods manually.
-
Builder Pattern Support: Lombok simplifies the creation of builder pattern-based APIs by automatically generating the builder pattern code for your classes, reducing boilerplate code and improving API readability.
-
Annotation Processors: Lombok uses annotation processors to modify the Java Abstract Syntax Tree (AST) during compilation, enabling the generation of code at compile time without affecting the runtime behavior of the application.
-
Immutable Data Classes: By using Lombok annotations, you can easily create immutable data classes, eliminating the need to write boilerplate code for constructors, getters, and equals/hashCode methods.
Integrating Lombok into Java Projects
Adding Lombok to Your Project
Integrating Project Lombok into your Java project is a straightforward process. You can add Lombok as a dependency in your project using a build automation tool such as Maven or Gradle.
For Maven, you can add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
For Gradle, you can add the following dependency to your build.gradle
file:
implementation 'org.projectlombok:lombok:1.18.20'
After adding the dependency, you need to ensure that your IDE supports Lombok annotations. Most popular IDEs, such as IntelliJ IDEA and Eclipse, have plugins or native support for Lombok, allowing you to leverage its features seamlessly.
Annotating Your Java Classes
Once Lombok is integrated into your project, you can start using its annotations to simplify your Java classes. Let's take a look at an example to understand how Lombok can reduce code size and improve readability.
Consider a simple Java class representing a Person
with name
and age
fields. Without Lombok, you would typically write getter and setter methods for these fields manually. However, with Lombok, you can achieve the same functionality with just a few annotations:
import lombok.Data;
@Data
public class Person {
private String name;
private int age;
}
In the example above, the @Data
annotation from Lombok automatically generates the getter and setter methods for the name
and age
fields, effectively reducing the amount of boilerplate code that needs to be written and maintained.
Understanding the Impact on Java Compilation
When you use Lombok annotations in your Java classes, it's essential to understand how they impact the compilation process. Unlike traditional code generation tools, Lombok modifies the AST during compilation, which means that the generated code is not present in the source files but is injected into the compiled classes.
This approach minimizes the impact on the source code size as the generated code is not directly visible or editable in the source files. It also ensures that the compiled output remains free of the additional Lombok-generated code, resulting in smaller bytecode and reduced JAR file size.
Potential Drawbacks and Considerations
While Project Lombok offers significant benefits in terms of code size reduction and codebase simplification, it's essential to consider potential drawbacks and issues that may arise when using Lombok in Java projects.
IDE and Tooling Support
Although most popular IDEs have native or plugin support for Lombok, there may be cases where certain IDE features, such as code analysis or refactoring tools, might not fully support Lombok-generated code. While the community and Lombok developers actively address such issues, it's crucial to stay informed about the compatibility of Lombok with your development environment.
Learning Curve
For teams or developers new to Project Lombok, there might be a learning curve associated with understanding and effectively utilizing Lombok's annotations and features. It's essential to invest time in educating team members about Lombok best practices and potential pitfalls to ensure smooth adoption and usage.
Debugging and Stack Traces
Another consideration when using Lombok is related to debugging and stack traces. Since Lombok generates code that does not directly appear in the source files, it may affect the readability of stack traces, making it slightly more challenging to trace issues back to the original source.
Key Takeaways
In conclusion, Project Lombok is a powerful tool that significantly impacts Java compilation, reduces code size, and enhances code maintainability. By leveraging Lombok's annotations and features, developers can streamline their Java code, eliminate boilerplate code, and improve overall code readability.
While Lombok offers numerous benefits, it's crucial to weigh the potential drawbacks and considerations, such as IDE support, learning curve, and debugging implications, before integrating Lombok into Java projects. With careful consideration and appropriate usage, Project Lombok can be a valuable addition to Java development, enabling developers to write cleaner, more concise code while improving productivity and codebase maintainability.
By embracing tools like Project Lombok, Java developers can continue to evolve and optimize their coding practices, ultimately enhancing the quality and efficiency of their software development processes.
As you dive deeper into Java development, understanding how tools like Project Lombok impact your codebase is crucial. The seamless integration of Lombok into your projects can be a game-changer, reducing code size and improving maintainability. If you want to learn more about optimizing your Java projects, check out our article on Evolving Java Development Practices for further insights.