Troubleshooting Elasticsearch Indexing Issues
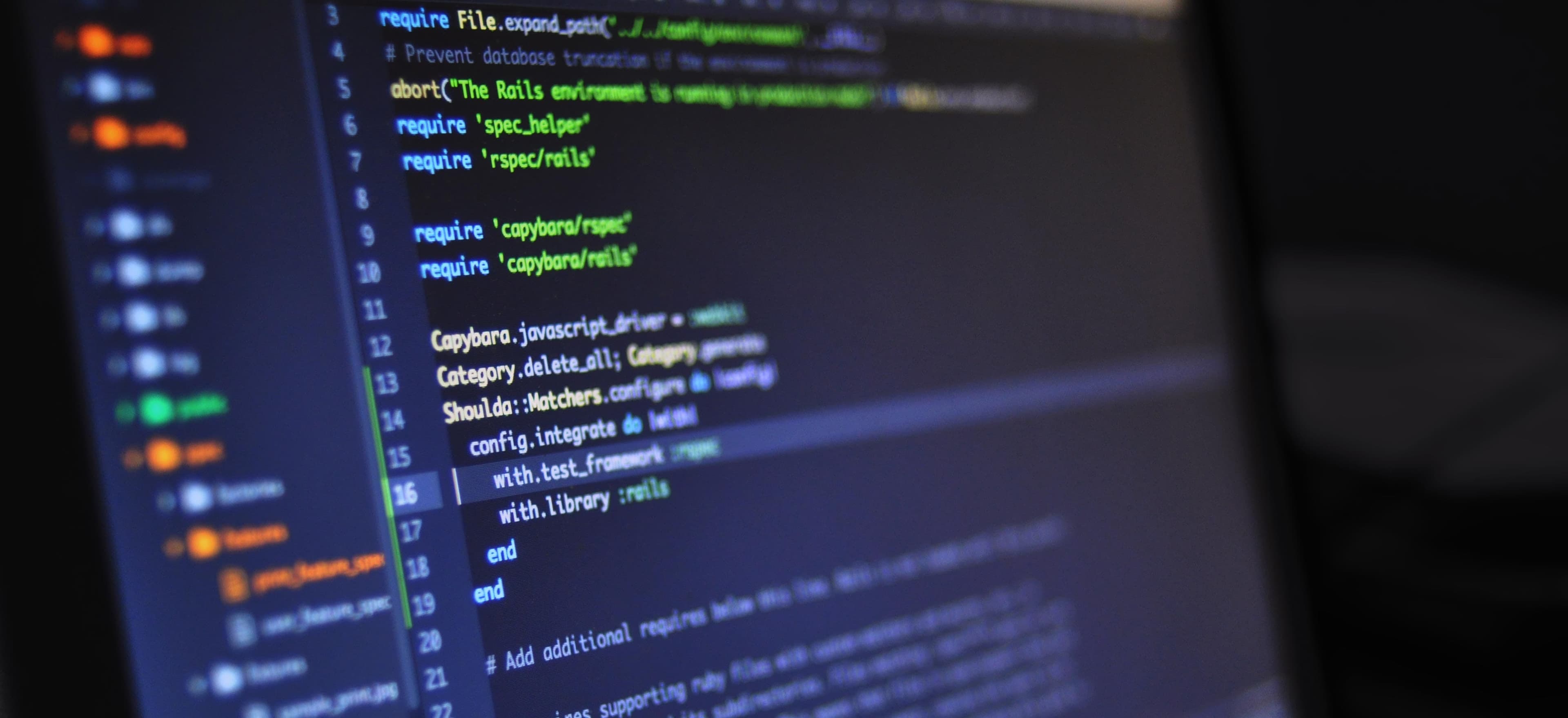
- Published on
Troubleshooting Elasticsearch Indexing Issues
Elasticsearch is a powerful and flexible search and analytics engine, but like any complex system, it can encounter indexing issues that affect its performance. In this blog post, we will explore common Elasticsearch indexing issues and provide practical solutions to troubleshoot and resolve them.
Understanding Elasticsearch Indexing
Before delving into troubleshooting, let's quickly refresh our understanding of Elasticsearch indexing. Indexing in Elasticsearch refers to the process of adding and updating documents in an index, making them searchable. When indexing, Elasticsearch analyzes the documents' content and structures the data for efficient search operations.
Common Indexing Issues
1. Mapping conflicts
Symptoms: Documents are not indexed, or certain fields are not searchable.
Possible Causes: Mapping conflicts occur when new fields have different data types than existing fields in the same index.
Solution: Use dynamic mapping templates to define explicit mappings for new fields or update the existing mappings to accommodate new data types.
2. Heap size issues
Symptoms: Frequent OutOfMemory errors during indexing operations.
Possible Causes: Insufficient heap memory allocated to Elasticsearch for handling indexing operations.
Solution: Increase the heap size allocated to Elasticsearch by modifying the jvm.options
file, and ensure that the node has enough physical memory to accommodate the increased heap size.
3. Thread pool rejections
Symptoms: High rejection rates on indexing thread pools.
Possible Causes: The default thread pool settings may not be suitable for the indexing load, leading to rejections.
Solution: Adjust the thread pool settings for indexing based on the workload and resource availability.
4. Slow disk I/O
Symptoms: Slow indexing performance, high disk queue length, or high I/O wait times.
Possible Causes: Inadequate disk I/O performance, often due to using slow or overloaded disks.
Solution: Upgrade to faster disks, distribute shards across multiple nodes, and optimize index settings for better disk utilization.
Troubleshooting Elasticsearch Indexing Issues
Now that we've identified some common indexing issues, let's dive into the troubleshooting process and explore practical solutions for each problem.
Analyzing Mapping Conflicts
When facing mapping conflicts, it's crucial to understand the structure of the documents being indexed and the current mappings in the index. One way to do this is by utilizing the _mapping
API in Elasticsearch.
// Retrieve mapping for a specific index
GetMappingsRequest request = new GetMappingsRequest();
request.indices("your_index_name");
Try {
GetMappingsResponse response = client.indices().getMapping(request, RequestOptions.DEFAULT);
Map<String, MappingMetadata> mappings = response.mappings();
// Analyze the retrieved mappings and document structure
} catch (IOException e) {
// Handle potential exceptions
}
By analyzing the mappings, you can identify any conflicts between the document structure and the defined mappings. If conflicts are found, you can update the mappings using the Put Mapping API.
// Update mappings to resolve conflicts
PutMappingRequest request = new PutMappingRequest("your_index_name");
request.source("{\n" +
" \"properties\": {\n" +
" \"new_field\": {\n" +
" \"type\": \"text\"\n" +
" }\n" +
" }\n" +
"}", XContentType.JSON);
Try {
AcknowledgedResponse putMappingResponse = client.indices().putMapping(request, RequestOptions.DEFAULT);
// Handle the response as needed
} catch (IOException e) {
// Handle potential exceptions
}
Optimizing Heap Size for Indexing
Inadequate heap size can significantly impact indexing performance. To optimize the heap size, it's essential to carefully monitor the memory usage of Elasticsearch during indexing operations. Tools like Elasticsearch's monitoring features or third-party monitoring solutions can provide insights into memory usage.
Once you have identified the need for a heap size adjustment, you can modify the jvm.options
file to allocate more memory to Elasticsearch.
Adjusting Thread Pool Settings
When encountering thread pool rejections during indexing, it's crucial to analyze the current thread pool settings and the indexing workload. The _nodes/stats/thread_pool
API can provide valuable information about the current thread pool rejections and queue sizes, aiding in determining the appropriate adjustments.
Based on the analysis, you can modify the thread pool settings using the Cluster Update Settings API to increase the queue size or adjust the number of threads for indexing operations.
Enhancing Disk I/O Performance
Improving disk I/O performance is vital for efficient indexing. Begin by evaluating the current disk performance using tools like iostat or Elasticsearch's Indexing Slowlog to identify any potential bottlenecks.
If slow disk I/O is identified as a bottleneck, consider upgrading to faster SSDs or optimizing the disk layout to distribute the I/O load effectively. Additionally, optimizing the index settings, such as the refresh interval and merge policies, can help enhance disk utilization during indexing.
Final Considerations
Troubleshooting Elasticsearch indexing issues requires a systematic approach, encompassing analysis, optimization, and proactive monitoring. By understanding the underlying causes of indexing problems and employing the appropriate solutions, you can ensure the stability and performance of your Elasticsearch cluster.
In this blog post, we have explored the common indexing issues faced in Elasticsearch and provided practical troubleshooting steps to address each issue. Armed with this knowledge, you are better equipped to tackle indexing challenges and maintain a robust Elasticsearch environment.
For further exploration of Elasticsearch troubleshooting and optimization, consider delving into Elasticsearch's official documentation and engaging with the vibrant Elasticsearch community. Happy troubleshooting!
Remember, efficient indexing leads to faster searches and a better user experience. Keep optimizing and happy coding!