Customizing JavaFX Pie Chart Appearance
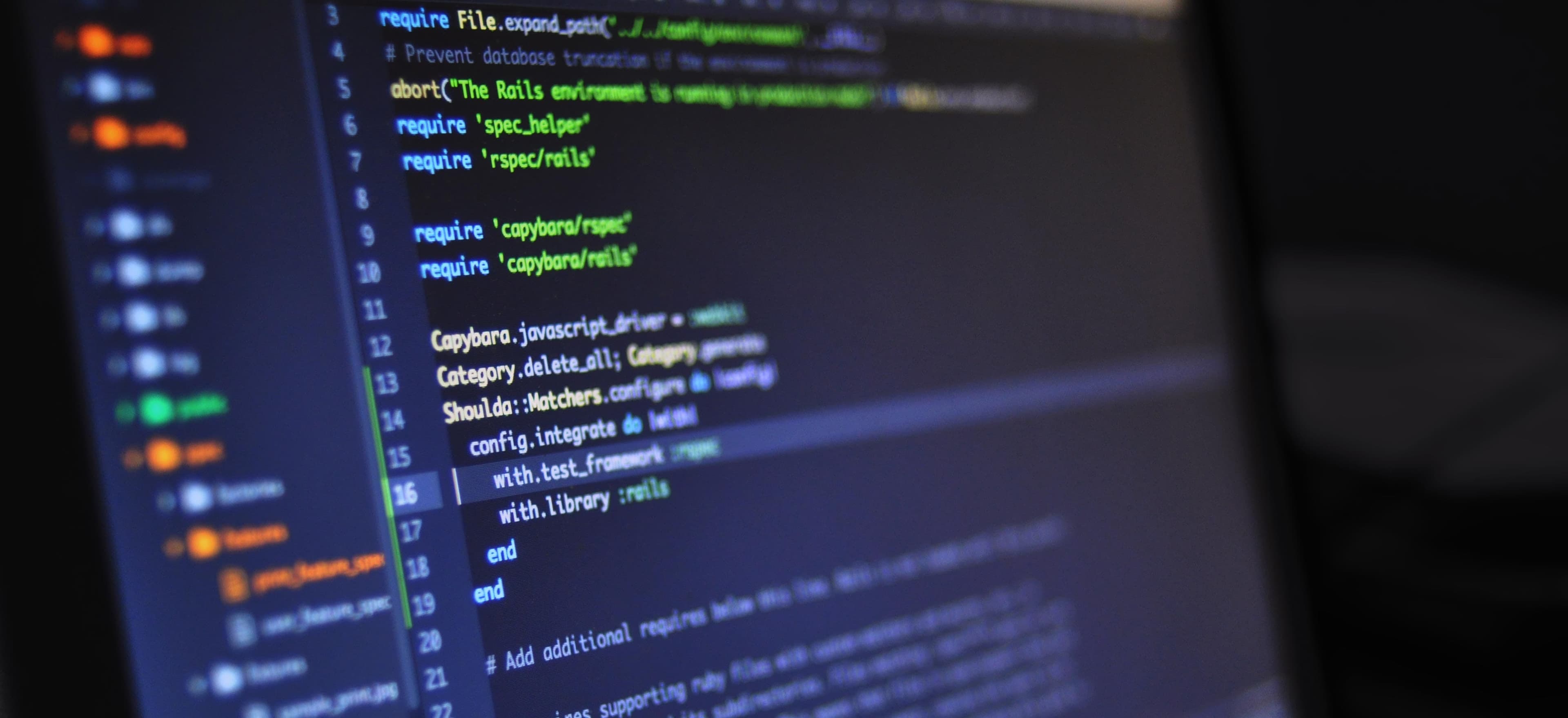
- Published on
Customizing JavaFX Pie Chart Appearance
JavaFX provides a versatile platform for creating interactive and visually appealing applications. Pie charts are a popular way to visualize data distribution, and JavaFX makes it easy to create and customize them according to specific design requirements. In this post, we will explore how to customize the appearance of a JavaFX pie chart to create a more engaging and professional visual representation of data.
Creating a Basic Pie Chart
Let's start by creating a basic pie chart in JavaFX. We will use the PieChart
class to initialize the chart and populate it with some sample data.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.chart.PieChart;
import javafx.stage.Stage;
public class CustomPieChartApp extends Application {
@Override
public void start(Stage stage) {
PieChart.Data slice1 = new PieChart.Data("Category A", 30);
PieChart.Data slice2 = new PieChart.Data("Category B", 25);
PieChart.Data slice3 = new PieChart.Data("Category C", 20);
PieChart chart = new PieChart();
chart.getData().addAll(slice1, slice2, slice3);
Scene scene = new Scene(chart);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, we have created a simple JavaFX application that displays a pie chart with three data slices representing different categories.
Customizing Pie Chart Appearance
Changing Slice Colors
One way to customize the appearance of a pie chart is to change the default colors of the data slices. This can be achieved by accessing the Node
that represents each slice and setting a new fill color.
// Customizing Slice Colors
slice1.getNode().setStyle("-fx-pie-color: #ff0000;"); // Red color
slice2.getNode().setStyle("-fx-pie-color: #00ff00;"); // Green color
slice3.getNode().setStyle("-fx-pie-color: #0000ff;"); // Blue color
By applying different fill colors to each slice, we can create a more visually appealing representation of the data. This level of customization allows for the alignment of the pie chart's appearance with specific design requirements or branding guidelines.
Setting Label Visibility and Location
The default pie chart in JavaFX displays labels for each data slice. We can control the visibility and location of these labels to improve the chart's readability and visual appearance.
// Setting Label Visibility and Location
chart.setLabelLineLength(10); // Adjust label line length
chart.setLegendVisible(false); // Hide legend
By adjusting the label line length and hiding the legend, we can declutter the chart and direct the focus towards the data slices, resulting in a cleaner and more professional appearance.
Adding Animation
To enhance the user experience, we can add animation to the pie chart, providing a more engaging and interactive visualization.
// Adding Animation
chart.setAnimated(true); // Enable animation
Enabling animation introduces a dynamic element to the chart, making it more visually appealing and capturing the user's attention.
A Final Look
Customizing the appearance of a JavaFX pie chart allows developers to create visually engaging and professional data visualizations tailored to specific design requirements. By changing slice colors, adjusting label visibility and location, and adding animation, we can elevate the visual appeal of pie charts in JavaFX applications, enhancing the overall user experience.
In this post, we have explored the various ways to customize the appearance of a JavaFX pie chart to create visually appealing and professional data visualizations. By incorporating these customization techniques, developers can create engaging and interactive applications with visually stunning pie charts.
By leveraging JavaFX's flexibility and customization options, developers can elevate the visual appeal of their applications, ultimately enhancing the user experience and delivering impactful data visualizations.
To explore more advanced customization options and best practices for JavaFX pie charts, check out JavaFX Pie Chart Documentation. Additionally, you can refer to the JavaFX CSS Reference Guide for in-depth information on customizing the appearance of JavaFX components using CSS.
Start customizing your JavaFX pie charts today and elevate your data visualization game!