Handling UUID Identifiers in Hibernate: Best Practices
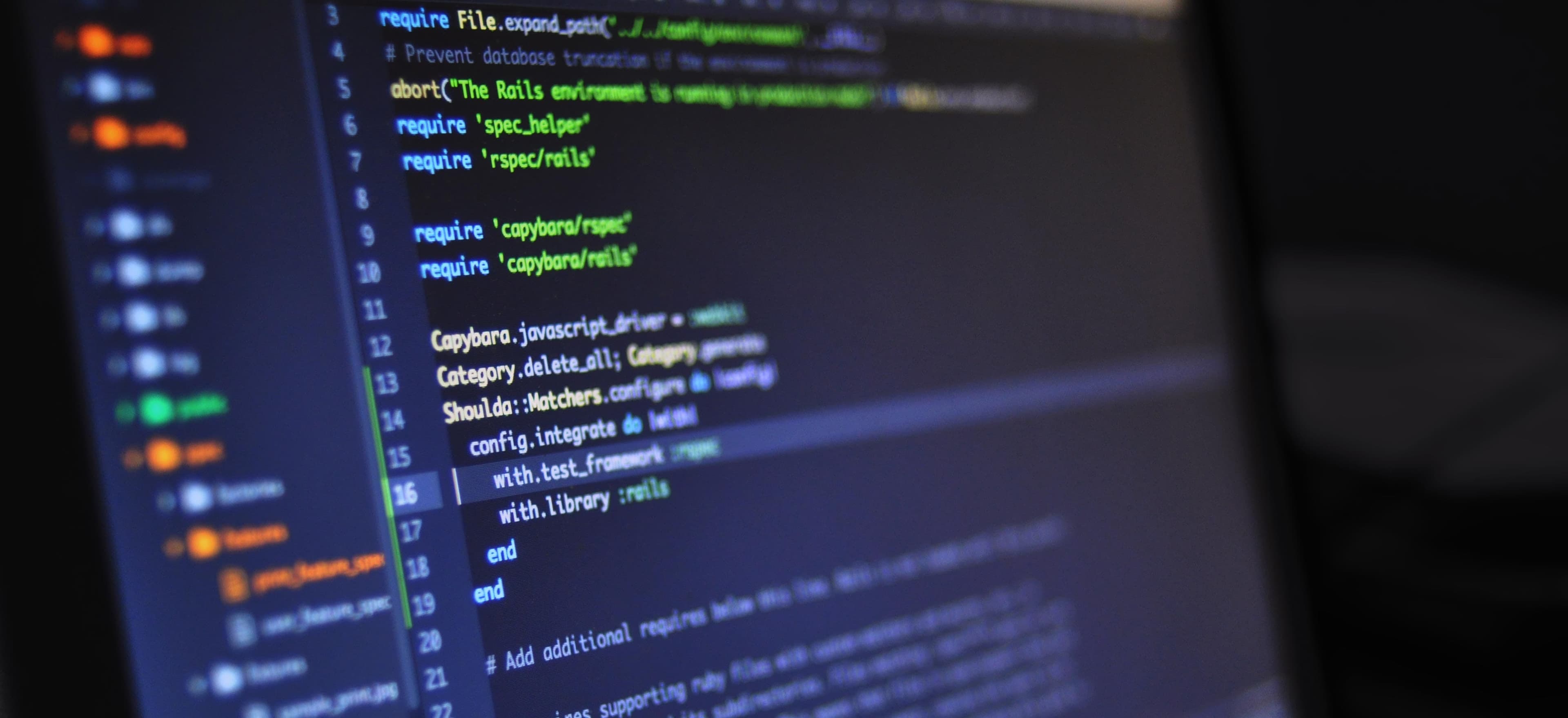
- Published on
Handling UUID Identifiers in Hibernate: Best Practices
Developers often face the challenge of selecting the right type of identifier for their Hibernate entities. While the traditional approach involves using numeric identifiers (such as auto-incrementing primary keys), there is an increasing trend towards using UUID (Universally Unique Identifier) as identifiers for the entities. In this blog post, we will explore the best practices for handling UUID identifiers in Hibernate, the benefits of using UUIDs, and delve into the implementation details.
Why Use UUIDs as Identifiers?
UUIDs are 128-bit numbers that guarantee uniqueness across space and time, even when generated on separate devices. Using UUIDs as identifiers offers several advantages:
-
Uniqueness: Unlike auto-incrementing primary keys, UUIDs can be generated across different systems without the risk of collision.
-
Security: UUIDs do not reveal any information about the entity's creation order or quantity, which can be a security concern with sequential numeric identifiers.
-
Decoupling from the Database: UUIDs allow entities to be identified uniquely without relying on the database to generate and manage keys.
Now, let's delve into the implementation details for using UUIDs as identifiers in Hibernate.
Mapping UUIDs in Hibernate
To use UUIDs as identifiers for entities in Hibernate, we need to map the UUID type in the entity class. Let's consider an example of an entity Product
with a UUID identifier.
@Entity
public class Product {
@Id
@GeneratedValue(generator = "uuid2")
@GenericGenerator(name = "uuid2", strategy = "org.hibernate.id.UUIDGenerator")
@Type(type = "uuid-char")
private UUID id;
// Other entity properties and methods
}
In the above code snippet, the @GeneratedValue
annotation along with @GenericGenerator
configures Hibernate to use a UUID generator for the id
field. The @Type
annotation specifies the mapping of the UUID to a CHAR column in the database.
By using the org.hibernate.id.UUIDGenerator
strategy, Hibernate will generate UUID values for the id
when a new Product
entity is persisted.
Best Practices for Using UUIDs in Hibernate
While using UUIDs as identifiers in Hibernate, it's important to adhere to some best practices to ensure efficient and optimal performance. Let's discuss some of these best practices.
1. Use the @GenericGenerator
Annotation
When mapping UUID identifiers, it is crucial to use the @GenericGenerator
annotation to specify the strategy for generating UUIDs. This allows for flexibility in choosing the UUID generation strategy that best suits the application's requirements.
2. Consider the Database Column Type
When mapping UUIDs to the database, it's essential to consider using an appropriate column type. In the example above, the @Type(type = "uuid-char")
annotation ensures that the UUID is stored as a CHAR data type in the database. Depending on the database system used, the column type for UUID may vary (e.g., BINARY(16)
for MySQL, UUID
for PostgreSQL).
3. Generating UUIDs in the Application Layer
While Hibernate provides built-in support for generating UUIDs, in some scenarios, it may be beneficial to generate UUIDs in the application layer before persisting the entity. This approach allows for customizing the UUID generation logic based on specific requirements.
4. Performance Considerations
When using UUIDs as identifiers, especially in high-performance systems, it's important to consider the performance implications. UUIDs are larger and more complex than numeric identifiers, which can impact indexing, querying, and storage requirements. Evaluating the trade-offs between uniqueness and performance is essential.
Wrapping Up
In conclusion, using UUIDs as identifiers in Hibernate offers various benefits such as uniqueness, security, and decoupling from the database. By following best practices such as utilizing the @GenericGenerator
annotation, considering the database column type, and evaluating performance implications, developers can effectively handle UUID identifiers in Hibernate.
By implementing the discussed best practices, developers can leverage the power of UUIDs as identifiers while ensuring optimal performance and maintainability of their Hibernate entities.
Incorporating UUIDs as identifiers in Hibernate enhances the robustness and security of the application's data model, providing a solid foundation for scalable and reliable systems.
To further explore the concepts discussed in this blog post, refer to the official Hibernate documentation for UUID Generation and Custom UUID Generation.
Start integrating UUID identifiers in your Hibernate entities to unlock the potential of unique and secure entity identification!