Improving JMeter Performance for Large-scale Applications
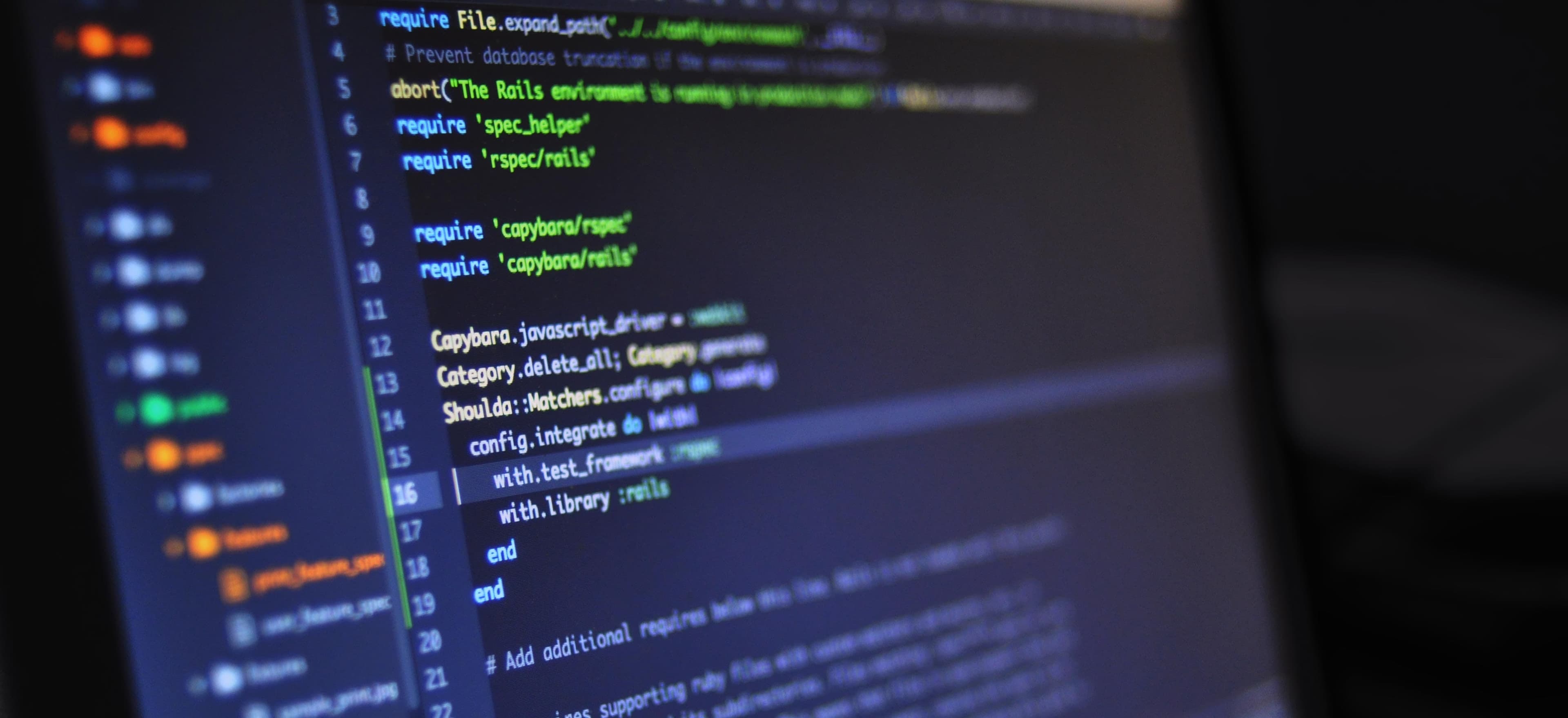
- Published on
Understanding the Importance of Performance Testing in Java Applications
Performance testing is a critical aspect of Java application development. It ensures that the application performs efficiently under expected workloads and usage scenarios. Apache JMeter is a popular open-source tool used for performance testing in Java applications. However, when dealing with large-scale applications, it becomes crucial to optimize JMeter for better performance.
In this blog post, we will explore various strategies for improving JMeter performance in the context of large-scale Java applications, including efficient test plan design, resource allocation, and result analysis.
Efficient Test Plan Design
Thread Group Configuration
The Thread Group element in JMeter allows you to simulate the desired number of users for your application. When dealing with large-scale applications, it's essential to optimize the thread configuration to ensure realistic load testing. Use the Concurrency Thread Group plugin to handle a large number of threads efficiently.
ConcurrencyThreadGroup threadGroup = new ConcurrencyThreadGroup();
threadGroup.setNumThreads(1000);
threadGroup.setRampUp(300);
threadGroup.setHoldFor(1800);
threadGroup.setScheduler(true);
Here, the setNumThreads
method sets the number of threads, setRampUp
determines the time to start all the threads, setHoldFor
specifies the duration to hold the threads, and setScheduler
enables the scheduler.
Targeted Controller and Samplers
Utilize the Logic Controllers in JMeter to create a well-structured and organized test plan. For large-scale applications, using the Throughput Controller to control the throughput of samplers can help distribute the load effectively.
ThroughputController controller = new ThroughputController();
controller.setPercentThroughput(70);
In this example, the Throughput Controller ensures that the contained samplers execute at a specified throughput.
Resource Allocation and Monitoring
Memory Allocation
When running JMeter for large-scale applications, it's vital to allocate sufficient memory to JMeter to handle the increased workload. This can be achieved by modifying the jmeter.bat
or jmeter.sh
file to increase the heap size.
set HEAP=-Xms2g -Xmx2g
Here, the -Xms
parameter sets the initial heap size, and the -Xmx
parameter sets the maximum heap size.
Monitoring Tools
Incorporate monitoring tools, such as JVisualVM or Taurus, to analyze the memory usage, CPU load, and thread activity of the JMeter instance during the test execution. This helps in identifying potential bottlenecks and optimizing the resource allocation.
Result Analysis and Reporting
Aggregate Report and Summary Report
After executing the performance tests, analyze the results using the Aggregate Report and Summary Report listeners in JMeter. These listeners provide essential metrics such as response times, throughput, and error rates, enabling you to identify performance bottlenecks and areas for improvement.
Backend Listener and InfluxDB
For large-scale applications, consider using the Backend Listener with InfluxDB to store test results. InfluxDB provides a scalable time-series database, suitable for storing and analyzing large volumes of performance data.
<BackendListener guiclass="BackendListenerGui" testclass="BackendListener" ...>
<stringProp name="influxdbMetricsSender">org.apache.jmeter.visualizers.backend.influxdb.services.HttpMetricsSender</stringProp>
...
</BackendListener>
The Backend Listener configuration includes specifying the InfluxDB metrics sender to store the test metrics in the InfluxDB database.
The Last Word
In the context of large-scale Java applications, optimizing JMeter for performance testing is crucial to ensure accurate simulation of user load and efficient analysis of test results. By focusing on efficient test plan design, resource allocation, and result analysis, you can enhance the effectiveness of performance testing for your Java applications.
For more in-depth understanding and advanced techniques, the Official JMeter Documentation provides comprehensive guidance on configuring, executing, and analyzing performance tests using JMeter. Additionally, exploring case studies and best practices from industry experts can offer valuable insights into optimizing JMeter for large-scale applications.