Common Challenges in Implementing OAuth 2.0
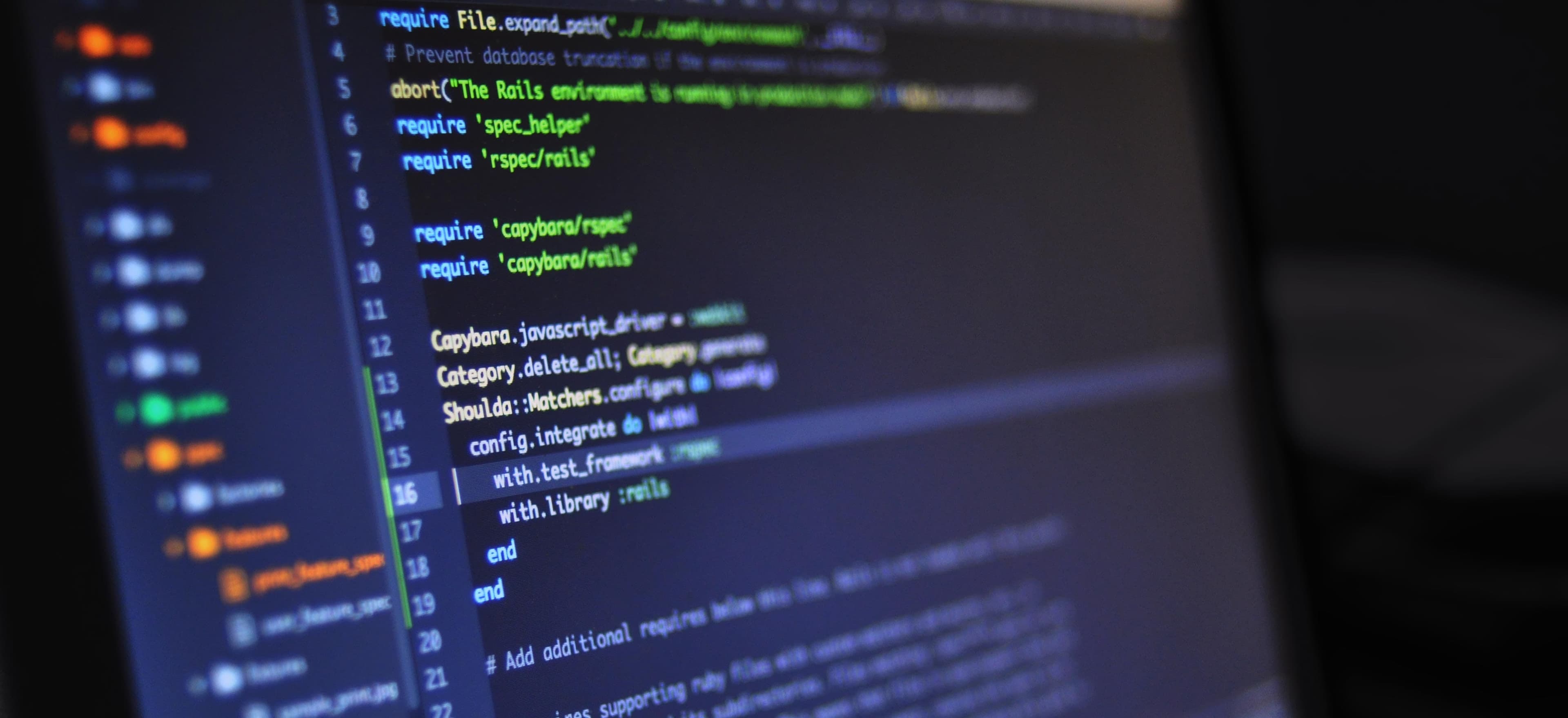
- Published on
Common Challenges in Implementing OAuth 2.0
OAuth 2.0 is a widely used authorization framework that allows applications to obtain limited access to user accounts on an HTTP service. While OAuth 2.0 provides a robust and secure method for authorization, it comes with its own set of challenges during implementation. In this article, we'll explore some common challenges developers face when implementing OAuth 2.0 and discuss strategies to overcome these challenges.
1. Understanding OAuth 2.0
One of the initial challenges in implementing OAuth 2.0 is understanding the specification itself. OAuth 2.0 is a complex protocol with various grant types, roles, and flows. Developers need to have a solid understanding of these concepts to effectively implement OAuth 2.0 in their applications.
Code Example
// Define OAuth 2.0 grant types
public enum GrantType {
AUTHORIZATION_CODE,
IMPLICIT,
CLIENT_CREDENTIALS,
PASSWORD
}
In the above example, defining the grant types as an enum helps in understanding and categorizing the different types of grants provided by OAuth 2.0.
2. Choosing the Right Grant Type
OAuth 2.0 defines several grant types, each suitable for different use cases. Selecting the appropriate grant type for a specific scenario is crucial but can be challenging without a clear understanding of the requirements.
Code Example
// Using OAuth 2.0 Authorization Code Grant
public void authorizeWithCodeGrant() {
// Implement authorization code grant flow
}
Here, the choice of using the Authorization Code Grant is based on the specific requirements of the application, ensuring the appropriate grant type is chosen for the scenario.
3. Token Management
Managing access tokens and refresh tokens is pivotal in OAuth 2.0. Handling token expiration, revocation, and securely storing tokens poses a challenge, requiring careful consideration to ensure the security and integrity of the tokens.
Code Example
// Validating Access Token
public boolean isAccessTokenValid(String accessToken) {
// Validate the access token
return true;
}
The above method demonstrates the necessity of validating access tokens to ensure their legitimacy, which is crucial for token management.
4. Security Considerations
Security is paramount in OAuth 2.0 implementation. Ensuring secure communication, proper authorization, and protection against common security threats such as CSRF and token leakage is a challenging aspect that needs to be carefully handled.
Code Example
// Preventing CSRF Attacks
public void validateCSRFToken(String tokenFromRequest, String tokenFromSession) {
// Compare tokens to prevent CSRF attacks
}
In the code snippet above, the method demonstrates the validation of CSRF tokens to prevent potential security threats, highlighting the importance of security considerations in OAuth 2.0 implementation.
5. Error Handling and User Experience
Handling errors and providing a seamless user experience in case of authorization failures or token expiration is a common challenge. A well-defined error handling strategy is crucial to ensure that users are informed appropriately without compromising security.
Code Example
// Handling Token Expiry
public void handleTokenExpiry() {
// Implement logic to handle token expiry
}
The example outlines the necessity of implementing logic to handle token expiry gracefully, contributing to a positive user experience.
6. Compliance with the OAuth 2.0 Specification
Adhering to the OAuth 2.0 specification can be challenging, especially with its evolving nature. Staying updated with the latest best practices and changes in the specification while ensuring compliance adds complexity to the implementation process.
Code Example
// Version-specific Implementation
public void implementOAuth2Spec() {
// Implement OAuth 2.0 as per the latest specification
}
The code snippet highlights the importance of keeping the implementation aligned with the latest OAuth 2.0 specification, ensuring compliance with the evolving standards.
Final Thoughts
In conclusion, implementing OAuth 2.0 in applications brings forth various challenges, ranging from understanding the protocol to ensuring security and compliance with the specification. By addressing these challenges with careful consideration and adherence to best practices, developers can effectively overcome the hurdles associated with OAuth 2.0 implementation. Embracing a thorough understanding of the protocol, selecting appropriate grant types, and prioritizing security considerations are crucial steps in achieving a robust and secure OAuth 2.0 implementation.
Adopting a strategic approach towards token management, error handling, and user experience can further contribute to a successful implementation of OAuth 2.0. Overall, navigating through these challenges while upholding the principles of OAuth 2.0 can lead to a secure, reliable, and effective authorization framework within applications.
By addressing these challenges with careful consideration and adherence to best practices, developers can effectively overcome the hurdles associated with OAuth 2.0 implementation. Embracing a thorough understanding of the protocol, selecting appropriate grant types, and prioritizing security considerations are crucial steps in achieving a robust and secure OAuth 2.0 implementation.