Effective Documentation Strategies for RESTful APIs
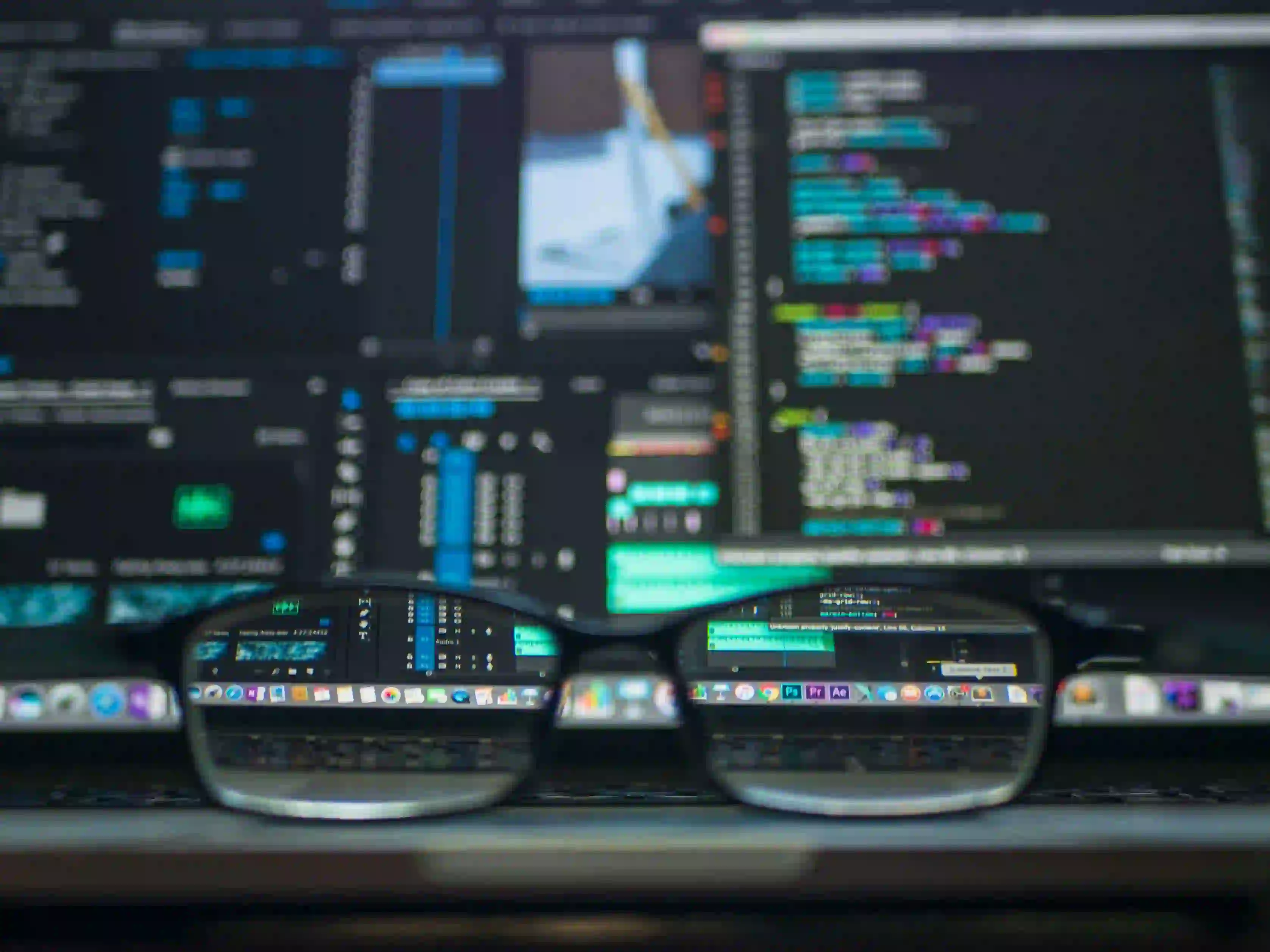
The Importance of Documentation for RESTful APIs
When developing a RESTful API, writing effective documentation is just as crucial as writing clean, efficient code. API documentation serves as the interface between the API provider and API consumers. It provides clarity on how to use the API, what endpoints are available, what request and response formats to expect, and any specific authentication requirements.
In this post, we’ll explore some strategies for creating clear, concise, and comprehensive documentation for your RESTful API using Java. We’ll cover the use of tools, best practices, and the importance of keeping the documentation up to date.
Choosing the Right Documentation Tool
Selecting the right tool for documenting your RESTful API is the first step towards creating effective documentation. In Java, one of the popular choices is Swagger, which allows for both API design and documentation. Swagger generates interactive API documentation, making it easier for consumers to understand and test the API endpoints.
Another widely used tool is Spring REST Docs, particularly for APIs built using the Spring framework. This tool integrates with testing frameworks like JUnit to produce accurate and concise documentation based on the tests written for the API.
Best Practices for Documenting RESTful APIs
Now, let’s dive into some best practices for documenting RESTful APIs using Java:
1. Use Descriptive Endpoint URLs
When defining endpoint URLs, use clear and descriptive path names to convey the purpose of the endpoint. For example, instead of /user/1234/orders
, use /users/{userId}/orders
to indicate that the endpoint retrieves orders for a specific user.
2. Provide Detailed Endpoint Descriptions
For each endpoint, provide a comprehensive description that explains its functionality, expected input parameters, and the structure of the response. Use clear and concise language to make it easy for developers to understand how to interact with the API.
3. Use Code Examples
Including code examples in your documentation can significantly aid developers in understanding how to make requests to the API. Provide examples in Java using popular libraries like RestTemplate
or HttpClient
to demonstrate how to consume the API from a client application.
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.example.com/users/1234/orders";
Order[] orders = restTemplate.getForObject(url, Order[].class);
In this example, we use RestTemplate
to make a GET request to the /users/1234/orders
endpoint and receive an array of Order
objects in response.
4. Document Request and Response Formats
Clearly document the expected request formats (such as JSON or XML) for each endpoint, including any required headers or query parameters. Similarly, outline the structure of the response and include example responses to illustrate the expected data format.
5. Explain Authentication and Authorization
If your API requires authentication or authorization, provide detailed instructions on how to authenticate requests, including the necessary headers or tokens. Explain the authorization mechanisms and any required permissions for accessing different endpoints.
6. Keep the Documentation Updated
As the API evolves and new features are added, it’s essential to keep the documentation updated. Changes to endpoints, request/response formats, or authentication mechanisms should be reflected in the documentation to avoid confusion for API consumers.
Final Considerations
Creating effective documentation for your RESTful API using Java is crucial for fostering successful API adoption. By choosing the right documentation tools, adhering to best practices, and keeping the documentation updated, you can provide a seamless experience for developers looking to integrate with your API.
Effective documentation serves as a bridge between the API provider and its consumers, enabling developers to understand, utilize, and ultimately benefit from the API's functionality. Incorporating these strategies into your API documentation process will enhance the developer experience and contribute to the overall success of your API.
By following these best practices and using the right tools, you can ensure that your RESTful API is well-documented and easily accessible to those who seek to leverage its capabilities.
For more in-depth understanding, check out the official documentation for Swagger and Spring REST Docs.