Creating Spring 4 CGLIB Proxy Classes Without Default Constructor
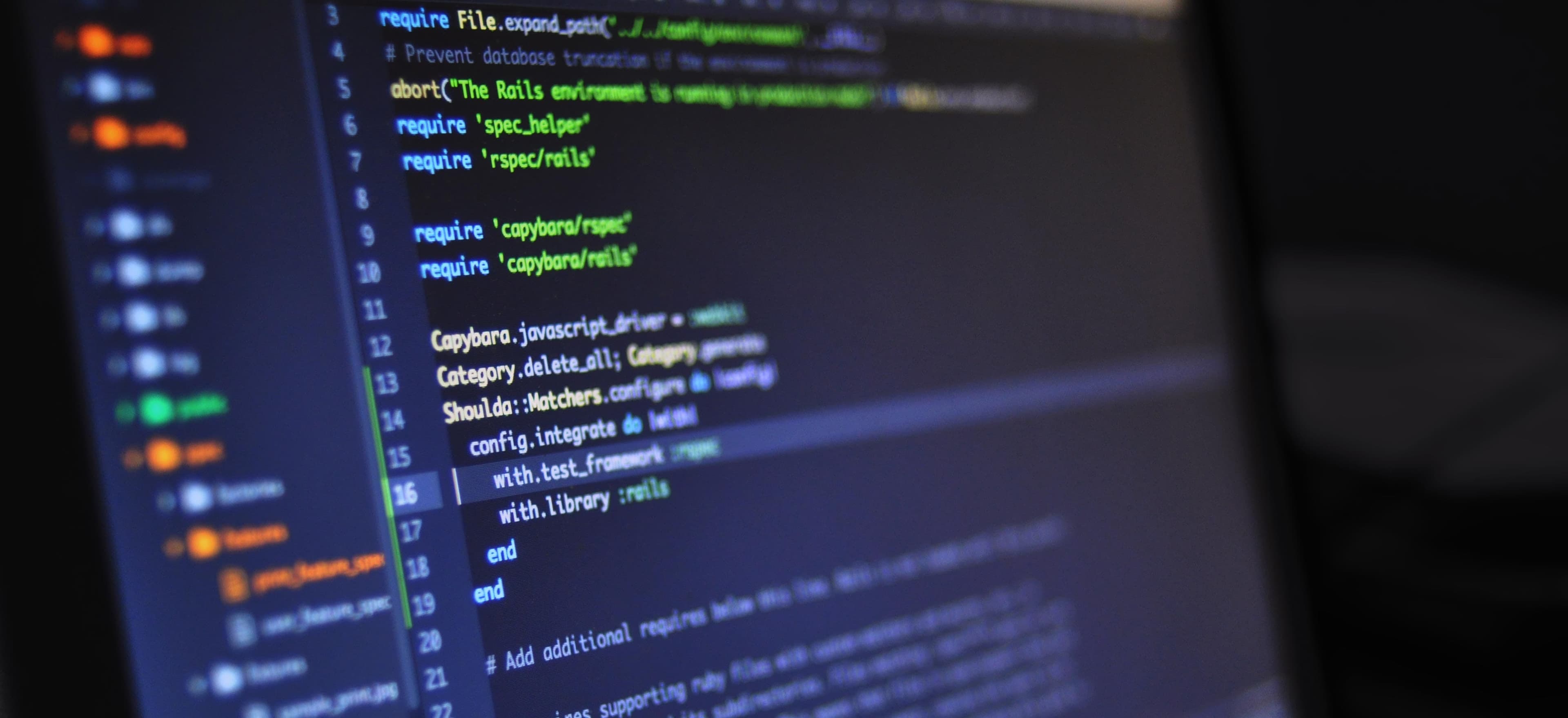
- Published on
How to Create Spring 4 CGLIB Proxy Classes Without Default Constructor
When working with Spring 4, you'll often find the need to create CGLIB proxy classes for your beans. However, a common issue that arises is when the target class does not have a default constructor. In such scenarios, Spring's default proxy creation mechanism using CGLIB fails. This article will guide you through the process of creating CGLIB proxy classes for such target classes.
Understanding CGLIB Proxy Classes
CGLIB is a powerful code generation library for Java, which is used to extend the capabilities of the Spring framework by creating runtime proxies. These proxies can be used to add additional behavior to classes without modifying their source code. When Spring AOP is configured to use CGLIB proxies (which is the default behavior), it generates these proxies on-the-fly to intercept method invocations and apply aspects.
The Issue with Default Constructor
The problem arises when the target class does not have a default, no-argument constructor. CGLIB, by default, creates the subclass by calling the no-argument constructor of the super class. If the superclass does not have a no-argument constructor, CGLIB fails to create the proxy class, resulting in an exception.
Solution using Enhancer and Constructor Args
To work around this limitation, we can use CGLIB's Enhancer
class to create the proxy class, providing the necessary constructor arguments. Let's take a look at an example to understand how this is done.
import net.sf.cglib.proxy.Enhancer;
public class CglibProxyFactory {
public static <T> T createCglibProxy(Class<T> targetClass, Class<?>[] constructorArgTypes, Object[] constructorArgs) {
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(targetClass);
enhancer.setCallback(/* Your MethodInterceptor */);
return (T) enhancer.create(constructorArgTypes, constructorArgs);
}
}
In the above example, we create a generic method createCglibProxy
which takes the targetClass
, constructorArgTypes
, and constructorArgs
as parameters. Instead of relying on the no-argument constructor, we use enhancer.create()
to specify the constructor arguments explicitly.
Example Usage
Let's assume we have a class TargetClass
with a constructor that takes a String
and an int
as arguments. We can create a CGLIB proxy using the CglibProxyFactory
as follows:
public class Main {
public static void main(String[] args) {
TargetClass target = CglibProxyFactory.createCglibProxy(TargetClass.class, new Class[]{String.class, int.class}, new Object[]{"example", 10});
target.doSomething();
}
}
The Closing Argument
By using the Enhancer
class and explicitly providing constructor arguments, we can create CGLIB proxy classes for target classes without default constructors in Spring 4. This approach allows us to overcome the limitations of CGLIB when dealing with non-default constructors.
For more details on CGLIB and Spring proxies, you can refer to the official documentation.
I hope this article helps you in creating CGLIB proxy classes for your non-default constructor target classes in Spring 4. Thank you for reading!