Handling Cardano Transaction Metadata in Java
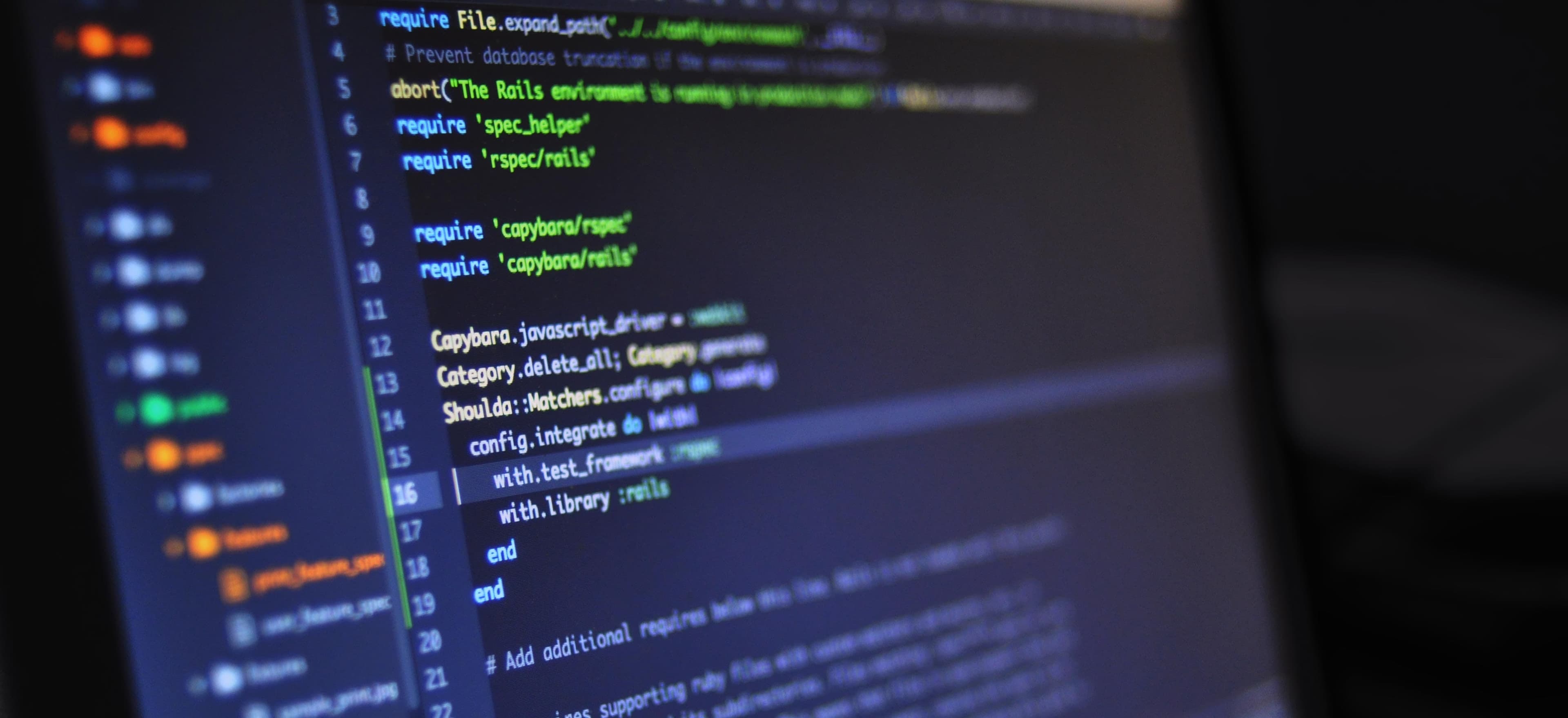
- Published on
A Guide to Handling Cardano Transaction Metadata in Java
Cryptocurrency transactions often involve more than just the transfer of funds. Additional data, known as metadata, can be attached to transactions to provide extra context or information. This metadata can be leveraged for various purposes, such as adding transaction notes, identifying the purpose of the transaction, or linking to external resources.
In this blog post, we'll explore how to handle Cardano transaction metadata in Java. Cardano is a popular cryptocurrency platform known for its focus on sustainability, scalability, and transparency. We'll delve into the process of retrieving metadata from Cardano transactions and demonstrate how Java can be used to work with this information.
Understanding Cardano Transaction Metadata
In the context of Cardano, transaction metadata is extra information that can be attached to a transaction. This metadata can be in the form of structured JSON data, allowing for flexibility in the types of information that can be included.
Metadata can serve various purposes, including but not limited to:
- Adding context to a transaction
- Associating transactions with specific applications or use cases
- Including human-readable information for interpretation
Understanding how to access and interpret this metadata is crucial for building robust applications that interact with the Cardano blockchain.
Retrieving Metadata from Cardano Transactions in Java
To retrieve metadata from Cardano transactions in Java, we can utilize the cardano-serialization-lib
library. This library provides the necessary tools to work with the binary serialization format used by Cardano.
First, you'll need to add the cardano-serialization-lib
dependency to your Java project. You can do this by including the following Maven dependency in your project's pom.xml
file:
<dependency>
<groupId>io.github.cadano</groupId>
<artifactId>cardano-serialization-lib</artifactId>
<version>1.0.2</version>
</dependency>
With the cardano-serialization-lib
dependency added, you can proceed to retrieve metadata from a Cardano transaction using the following Java code snippet:
import io.github.cadano.serialization.Encoding;
import io.github.cadano.serialization.Serialization;
import io.github.cadano.serialization.deserialization.TransactionMetadata;
public class TransactionMetadataHandler {
public void processTransactionMetadata(byte[] metadataBytes) {
TransactionMetadata metadata = Serialization.decodeTransactionMetadata(metadataBytes);
// Access and process the metadata as needed
}
}
In the above code, we import classes from the io.github.cardano.serialization
package to facilitate the decoding of transaction metadata. The decodeTransactionMetadata
method is used to decode the binary metadata into a usable format within the Java application.
It's important to note that the metadataBytes
parameter should be obtained from the Cardano transaction data, and this code assumes that the relevant transaction metadata bytes are provided as input.
Practical Use Case: Reading and Interpreting Metadata
Let's consider a practical use case where we want to read and interpret metadata from a Cardano transaction. Suppose we have a Java application that needs to fetch transaction metadata and display it to the user.
import io.github.cadano.serialization.Encoding;
import io.github.cadano.serialization.Serialization;
import io.github.cadano.serialization.deserialization.TransactionMetadata;
public class TransactionMetadataReader {
public String readTransactionMetadata(byte[] transactionBytes) {
TransactionMetadata metadata = Serialization.decodeTransactionMetadata(transactionBytes);
if (metadata != null) {
// Process the metadata
return metadata.toString();
} else {
return "No metadata found";
}
}
}
In the above example, the readTransactionMetadata
method decodes the transaction metadata and returns it as a string. If no metadata is found, a suitable message is returned.
This demonstrates a simple yet practical way to handle and interpret Cardano transaction metadata within a Java application.
Final Thoughts
In this guide, we've explored the process of handling Cardano transaction metadata in Java. By leveraging the cardano-serialization-lib
library, developers can work with transaction metadata in a convenient and efficient manner.
Understanding and working with transaction metadata is essential for building applications that interact with the Cardano blockchain. Whether it's adding context to transactions, associating transactions with specific use cases, or displaying human-readable information, the ability to handle metadata opens up a range of possibilities for developers.
By following the examples and leveraging the cardano-serialization-lib
, Java developers can seamlessly integrate the retrieval and interpretation of Cardano transaction metadata into their applications.
In conclusion, delving into Cardano transaction metadata in Java opens the door to creating intricate, context-rich applications that harness the full potential of blockchain technology.
For further exploration, you can refer to the official Cardano Developer Documentation and the cardano-serialization-lib GitHub repository to dive deeper into the intricacies of working with Cardano transaction metadata in Java. Happy coding!