Improving Code Quality Through Test-Driven Discipline
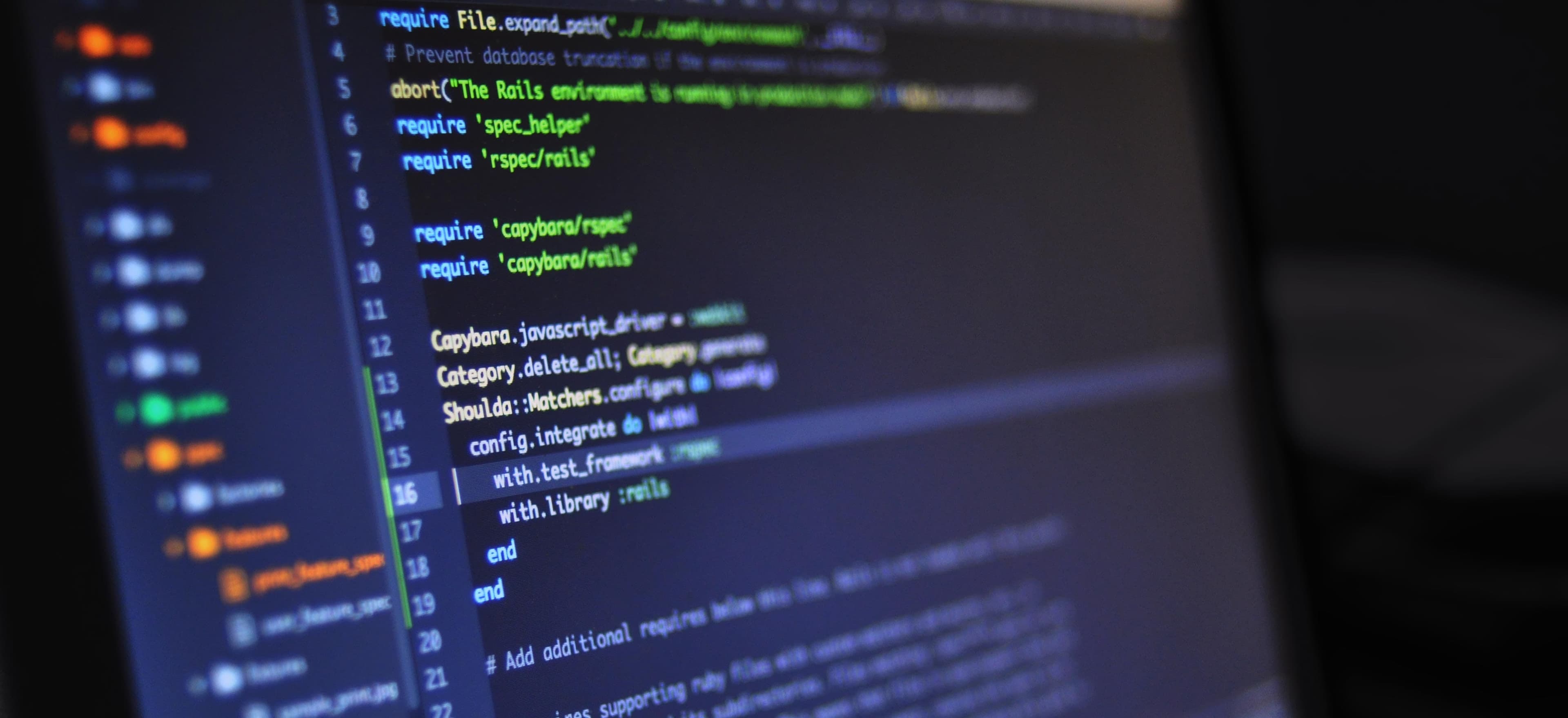
- Published on
Improving Code Quality Through Test-Driven Discipline
In the world of software development, the quest for achieving high-quality code is a never-ending journey. Code quality directly impacts the reliability, maintainability, and scalability of a software system. As a result, developers are constantly seeking ways to enhance code quality. One approach that has gained widespread acceptance is test-driven development (TDD).
Understanding Test-Driven Development (TDD)
Test-driven development is a software development approach where automated tests are written before the actual code. The development process in TDD follows a simple cycle: red, green, refactor. First, a new test is written and runs, resulting in a failure (red). Then, the necessary code changes are made to pass the test (green). Lastly, the code is refactored to improve its structure while keeping the tests passing.
Benefits of Test-Driven Development
1. Enhanced Code Quality
TDD forces developers to carefully think through the requirements and design of their code before writing it. This results in cleaner, more maintainable code.
2. Confidence in Refactoring
Since the code is backed by automated tests, developers can confidently refactor without the fear of breaking existing functionality.
3. Better Design
TDD encourages the development of modular and loosely coupled code, leading to a better overall design of the software.
Implementing Test-Driven Development in Java
Setting up the Project
Let's dive into implementing TDD in Java by setting up a simple project using Maven. First, ensure that Maven is installed on your system. Then, create a new Maven project and add the JUnit dependency to the pom.xml
file.
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
Writing the First Test
To demonstrate TDD in action, we will create a basic Calculator
class with a method for adding two numbers. Following the TDD approach, we start by writing a test case for the add
method.
import org.junit.Test;
import static org.junit.Assert.*;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Making the Test Pass
Now, we need to write the minimal amount of code in the Calculator
class to make the test pass.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
With the test in place, running it will result in a failure. After adding the add
method to the Calculator
class, running the test again will showcase a passing result.
Refactoring the Code
At this stage, the test has driven the development of the add
method, ensuring its correctness. Now, we can confidently refactor the code knowing that the existing test will catch any regressions.
Best Practices for Test-Driven Development
1. Write Focused Tests
Each test should focus on a specific behavior or scenario, allowing for better isolation and easier diagnosis of failures.
2. Refactor Continuously
Always refactor your code after making the test pass. This ensures that the codebase remains clean and maintainable.
3. Keep Tests Fast
Tests should execute quickly to allow for frequent run cycles, enabling a smooth development experience.
4. Embrace Red-Green-Refactor
Adhere strictly to the TDD cycle of writing a failing test, making it pass, and then refactoring the code.
Final Considerations
Test-driven development is a powerful practice for improving code quality, and when applied diligently, it can lead to a more robust and maintainable codebase. By using TDD, developers can gain confidence in their code, ensure a clearer design, and maintain a higher level of overall quality in their software.
Incorporating TDD into your Java projects can be a game-changer, and with the ample support provided by tools and frameworks like JUnit, adopting TDD becomes even more seamless. The discipline of TDD not only enhances the quality of code but also fosters a mindset of precision and clarity in the development process.
Start practicing TDD in your Java projects today, and experience the transformative impact it can have on your codebase!