Choosing the Correct JVM Bit Version
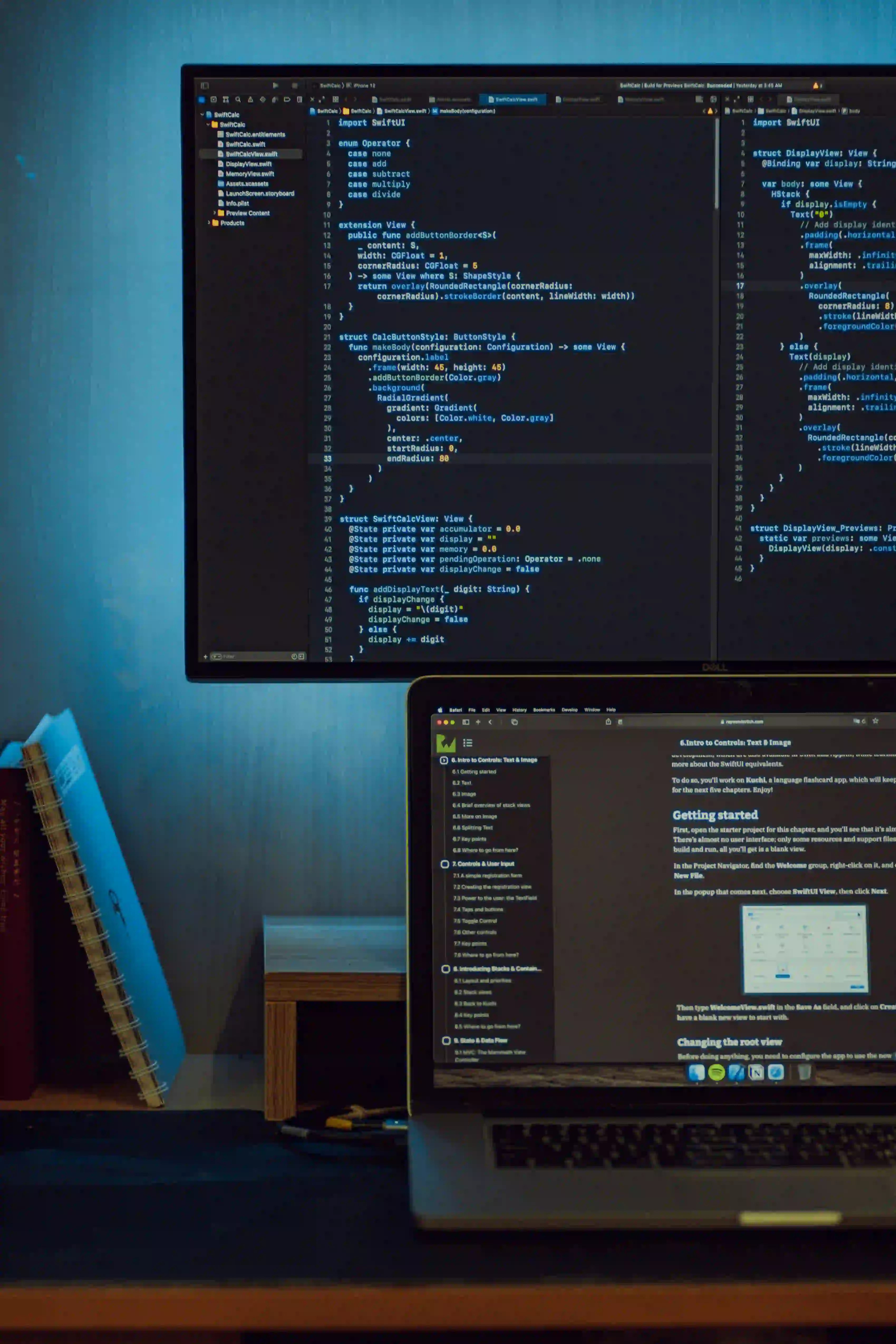
Title: Choosing the Correct JVM Bit Version for Your Java Applications
Introduction When developing Java applications, one crucial decision developers often face is selecting the appropriate Java Virtual Machine (JVM) bit version: 32-bit or 64-bit. The choice can significantly impact the performance, scalability, and compatibility of the application. In this guide, we will explore the differences between 32-bit and 64-bit JVMs, discuss the factors to consider when making a decision, and provide best practices for choosing the correct JVM bit version for your Java applications.
Understanding 32-bit and 64-bit JVMs Before delving into the decision-making process, it's essential to understand the fundamental disparities between 32-bit and 64-bit JVMs. The fundamental difference between them lies in memory addressing capabilities.
32-bit JVM:
- Limited to a maximum of 4GB of memory address space.
- Ideal for applications with smaller memory requirements.
- May encounter limitations when handling large datasets or memory-intensive operations.
64-bit JVM:
- Offers virtually unlimited memory addressing capabilities, depending on the underlying hardware and operating system.
- Suitable for memory-intensive applications, such as big data processing, scientific computing, and enterprise-level systems.
- Provides improved performance and scalability for larger workloads.
Factors Influencing the Decision Now that we comprehend the disparities, let's delve into the factors that should influence your choice of JVM bit version.
-
Application Requirements:
- Assess the memory needs of your application.
- Determining whether your application processes and stores substantial data, or if it requires enormous memory allocation during runtime, is crucial.
-
Platform Support:
- Consider the compatibility of your operating system and hardware with 64-bit JVMs.
- Ensure that all dependent libraries, frameworks, and third-party components are compatible with your chosen JVM bit version.
-
Performance Considerations:
- Evaluate the potential performance gains offered by a 64-bit JVM for your specific application workload.
- Determine if the improved memory handling and computational capabilities of a 64-bit JVM will positively impact your application's performance.
-
Future Scalability:
- Anticipate the future growth and scalability of your application.
- Choosing a 64-bit JVM can future-proof your application, allowing it to handle larger workloads as your business and user base expand.
Best Practices for Choosing the Correct JVM Bit Version Now that we have addressed the key considerations let's outline some best practices for selecting the appropriate JVM bit version for your Java applications.
-
Evaluate Memory Requirements:
- Conduct thorough memory profiling and analysis of your application to understand its memory consumption patterns.
- Use tools like Java Flight Recorder and Java Mission Control to gather insights into memory usage, object allocation, and garbage collection behavior.
-
Consult Java Vendor Recommendations:
- Different JVM providers may offer specific guidelines and recommendations for selecting the JVM bit version.
- Check the documentation and resources provided by vendors such as Oracle, OpenJDK, and AdoptOpenJDK for insights into the best practices for choosing the JVM bit version.
-
Test Performance and Compatibility:
- Perform extensive performance testing and compatibility checks with both 32-bit and 64-bit JVMs.
- Assess the impact of each JVM bit version on your application's performance, stability, and compatibility with external components.
-
Consider Long-Term Requirements:
- Factor in the long-term needs and growth projections for your application.
- Choosing a JVM bit version that aligns with your future scalability goals can save you from unforeseen limitations as your application evolves.
Example Code and Commentary Let's consider a scenario where an application's memory requirements are a crucial determinant for choosing the JVM bit version. The following Java code snippet illustrates the memory-intensive operations and the potential impact of using a 64-bit JVM for improved memory handling.
public class DataProcessingApplication {
public static void main(String[] args) {
// Simulating memory-intensive data processing
int[] data = new int[1000000000]; // Allocating a large array
// Perform memory-intensive operations
for (int i = 0; i < data.length; i++) {
data[i] = i * 2; // Manipulate large dataset
}
}
}
In this example, the application allocates a large array and performs memory-intensive operations on it. When executed with a 64-bit JVM, the application can efficiently handle the substantial memory allocation and manipulation, potentially resulting in improved performance and stability.
Conclusion Choosing the correct JVM bit version is a critical decision that can significantly impact the performance, scalability, and compatibility of your Java applications. By carefully evaluating your application's memory requirements, platform support, performance considerations, and long-term scalability needs, you can make an informed decision regarding whether to opt for a 32-bit or 64-bit JVM. Furthermore, following best practices, such as conducting thorough memory profiling, consulting Java vendor recommendations, and testing performance and compatibility, can help ensure that you select the most suitable JVM bit version for your specific use case.