Uncovering Hotspot Options in Java 8
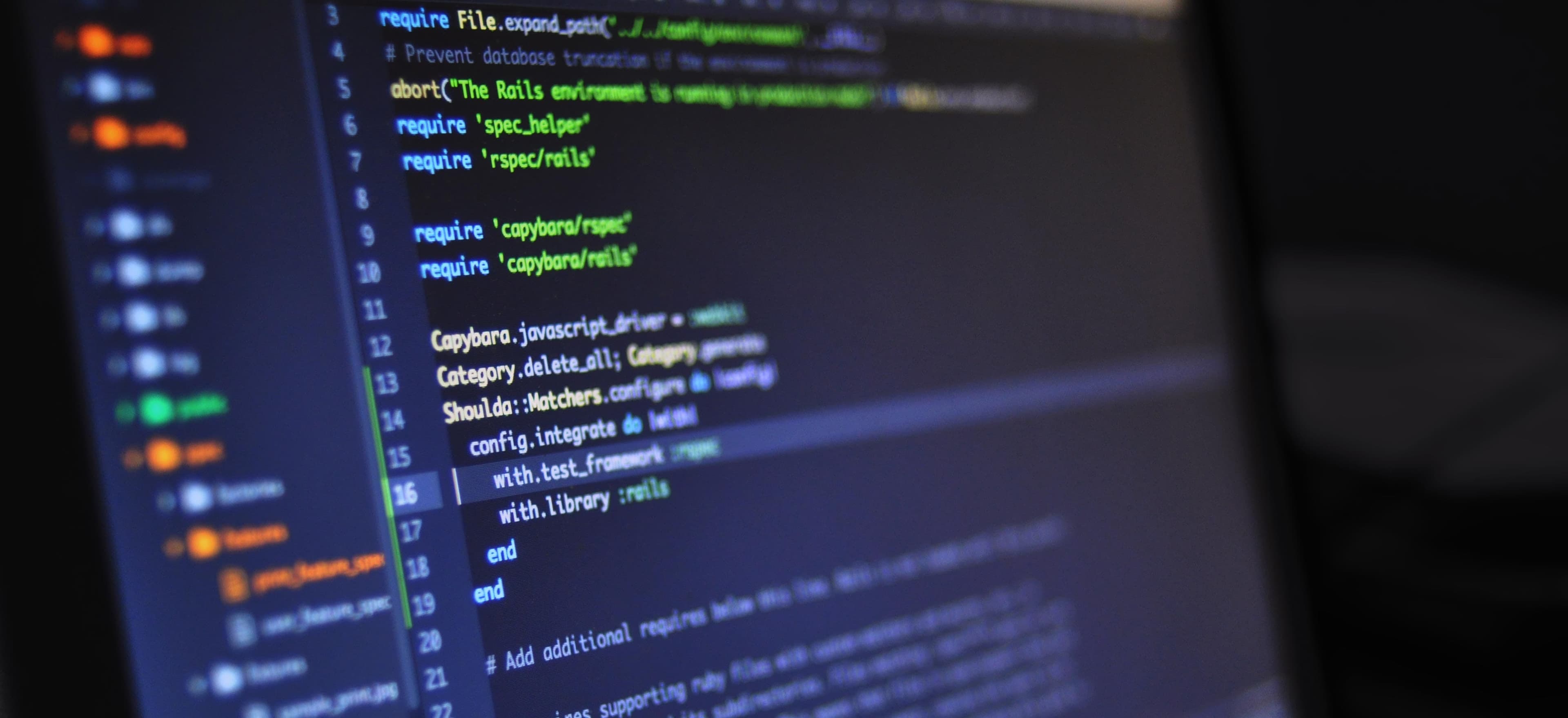
- Published on
Uncovering Hotspot Options in Java 8
Java is a popular programming language known for its versatility and performance. In Java development, optimizing the Java Virtual Machine (JVM) through Hotspot options is crucial for achieving peak performance. Java 8, in particular, introduced several Hotspot options that can be leveraged to enhance the performance of Java applications. In this article, we'll delve into these Hotspot options, understand their significance, and learn how to utilize them effectively.
Understanding Hotspot Options
Hotspot is the JVM implementation provided by OpenJDK and Oracle. It includes a plethora of options that allow developers to tune the JVM behavior according to the specific requirements of their applications.
These options can be broadly categorized into the following types:
-
Standard Options: These are options that are recognized by all JVM implementations. They control the overall behavior of the JVM, such as heap size, garbage collection, and runtime performance monitoring.
-
Extra Options: These options are specific to Hotspot and are not guaranteed to be supported by other JVM implementations. They typically provide fine-grained control over various internal aspects of Hotspot.
Exploring Hotspot Options in Java 8
Java 8 introduced several Hotspot options that are instrumental in fine-tuning the JVM performance. Let's explore some of the notable Hotspot options in Java 8:
1. Parallel GC Options
One of the significant features introduced in Java 8 is the G1 (Garbage-First) garbage collector, which aims to provide improved garbage collection performance compared to the traditional Parallel GC. However, for applications that still use the Parallel GC, Java 8 introduced new options to fine-tune its behavior.
Example Code:
java -XX:+UseParallelGC -XX:ParallelGCThreads=4 MyApp
In this example, we enable the use of Parallel GC (-XX:+UseParallelGC
) and specify the number of parallel threads to be used for garbage collection (-XX:ParallelGCThreads=4
). This allows us to optimize garbage collection for better performance in multi-core systems.
2. Metaspace Options
In Java 8, the permanent generation (PermGen) was replaced by Metaspace to store class metadata. Metaspace is not a part of the heap and is not subject to the same memory constraints as the permanent generation.
Example Code:
java -XX:MaxMetaspaceSize=256m MyApp
In this example, we set the maximum size of the Metaspace to 256 MB. This allows us to control the amount of native memory used for class metadata and prevent Metaspace from growing uncontrollably, potentially causing native memory exhaustion.
3. Tiered Compilation Options
Java 8 introduced the concept of tiered compilation, where methods are initially interpreted and then gradually compiled to native machine code for better performance. This feature is enabled by default but can be further fine-tuned using Hotspot options.
Example Code:
java -XX:+TieredCompilation -XX:TieredStopAtLevel=1 MyApp
Here, we enable tiered compilation (-XX:+TieredCompilation
) and set the compilation level at which tiered compilation stops (-XX:TieredStopAtLevel=1
). This allows us to control the extent of compilation performed by tiered compilation and optimize the application startup time.
4. Compiler Control Options
Java 8 introduced the CompileCommand
option, which allows developers to specify custom commands for the JIT compiler. This feature is particularly useful for fine-tuning the compilation behavior of specific methods or classes.
Example Code:
java -XX:CompileCommand=print,MyClass.myMethod MyApp
In this example, we instruct the JIT compiler to print its actions for the myMethod
in MyClass
. This enables developers to analyze and optimize the compilation behavior of specific methods, leading to improved runtime performance.
Bringing It All Together
In conclusion, Hotspot options in Java 8 offer a wide array of tools for fine-tuning the performance of Java applications. By understanding and effectively utilizing these options, developers can optimize garbage collection, manage memory consumption, and enhance the overall runtime performance of their applications.
It's essential to note that while the Hotspot options discussed in this article are relevant to Java 8, newer versions of Java may introduce additional options or deprecate existing ones. Therefore, developers should always refer to the official documentation and stay updated with the latest JVM enhancements.
In your Java development journey, mastering Hotspot options can be a game-changer, enabling you to squeeze out every bit of performance from your applications.
Start exploring these Hotspot options in Java 8 and witness the significant impact they can have on the performance of your Java applications!
If you want to delve deeper into Java performance optimization, check out this comprehensive guide on Java Performance Tuning.
Happy coding! 🚀