Optimizing Apache Arrow on the JVM for Streaming Writes
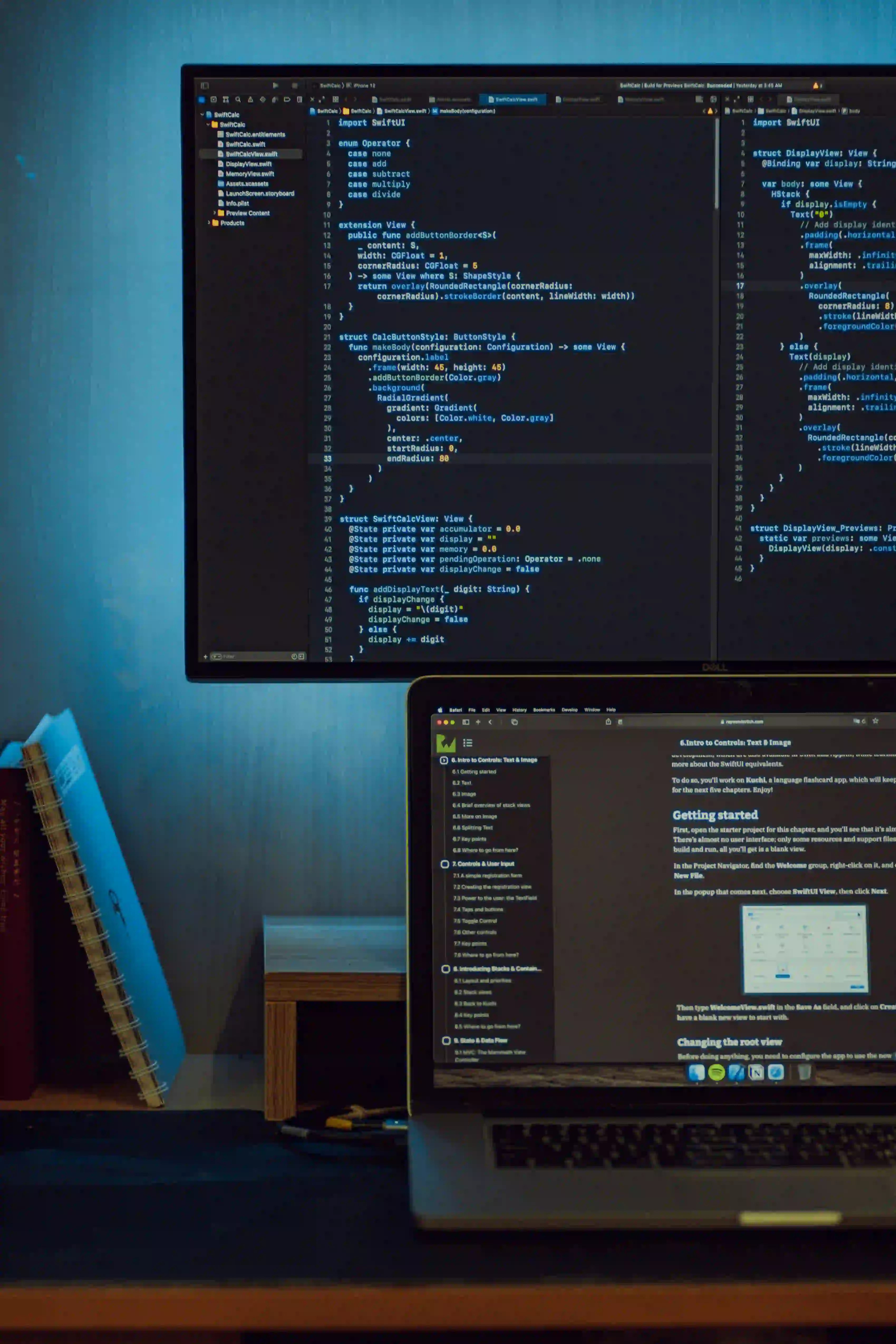
Optimizing Apache Arrow on the JVM for Streaming Writes
Apache Arrow is a widely-used in-memory columnar data format that provides a powerful way to represent data for analytical processing. In a streaming data environment, efficient writes in Apache Arrow on the JVM are crucial for performance and scalability. In this post, we will explore how to optimize Apache Arrow on the JVM for streaming writes, focusing on key strategies and best practices to achieve high throughput and low latency.
Understanding Apache Arrow
Apache Arrow is designed to efficiently represent structured data in a cross-language in-memory format. It provides a standardized language-agnostic columnar memory format for flat and hierarchical data, organized for efficient analytic operations on modern hardware.
Stream Writing in Apache Arrow
In a streaming data scenario, continuous ingestion of data necessitates efficient writing capabilities. When dealing with a high volume of incoming data in a streaming fashion, optimizing write performance becomes paramount. In Apache Arrow, the ArrowStreamWriter
class provides the functionality for efficiently writing Arrow data to an output stream.
try (FileOutputStream fileOutputStream = new FileOutputStream("output.arrow");
ArrowStreamWriter arrowStreamWriter = new ArrowStreamWriter(rootAllocator, rootSchema, fileOutputStream.getChannel())) {
// Write Arrow data in a streaming fashion
arrowStreamWriter.writeBatch();
} catch (IOException e) {
// Exception handling
}
The ArrowStreamWriter
class enables writing Arrow data in a streaming fashion. The writeBatch()
method can be called to persist the Arrow data to the output stream. This approach facilitates continuous writing of data into Arrow format, which is crucial for streaming use cases.
Optimizing Stream Writing Performance
Optimizing stream writing performance in Apache Arrow involves several key considerations. Let's delve into some best practices to achieve optimal throughput and low latency in streaming writes on the JVM.
1. Memory Management
Efficient memory management is critical for achieving high performance in streaming writes. Apache Arrow utilizes memory allocation pools to manage memory efficiently. By reusing memory buffers and minimizing unnecessary allocations, the overhead of memory management can be significantly reduced, leading to improved write throughput.
try (VectorSchemaRoot root = VectorSchemaRoot.create(rootSchema, rootAllocator)) {
// Use the VectorSchemaRoot for writing data
// Ensure proper handling and recycling of memory buffers
}
By leveraging the VectorSchemaRoot
and employing proper handling and recycling of memory buffers, the memory management overhead can be optimized, resulting in enhanced streaming write performance.
2. Buffer Reuse
Effective buffer reuse can have a substantial impact on stream writing performance. Reusing memory buffers, such as Arrow data buffers, reduces the need for frequent allocations and deallocations, thereby minimizing unnecessary overhead. This practice is particularly beneficial in high-throughput streaming scenarios where minimizing overhead is crucial for sustained performance.
ArrowBuf dataBuffer = rootAllocator.buffer(1024);
// Reuse the dataBuffer for writing Arrow data
// Ensure proper recycling of the buffer
By reusing memory buffers and ensuring proper recycling, the overhead associated with buffer management can be minimized, contributing to improved stream writing performance.
3. Parallelization
In streaming data processing, parallelization plays a vital role in maximizing throughput. Leveraging parallelization techniques, such as multi-threaded write operations, can help distribute the data writing workload across multiple threads, thereby increasing overall throughput. However, it is essential to ensure thread safety and proper synchronization when implementing parallel write operations to avoid data integrity issues.
ExecutorService executorService = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors());
// Execute parallel write tasks using executorService
Utilizing an ExecutorService
with appropriate thread management can facilitate parallel write operations, thus enhancing streaming write performance in Apache Arrow.
4. Data Serialization
Efficient data serialization is crucial for optimizing streaming writes in Apache Arrow. Leveraging high-performance serialization frameworks, such as Apache Avro or Apache Parquet, can significantly enhance the serialization process, leading to improved write throughput and reduced latency.
// Utilize Apache Avro or Apache Parquet for efficient data serialization
// Write serialized data to the ArrowStreamWriter
By integrating efficient data serialization techniques, the overall serialization overhead can be minimized, resulting in enhanced streaming write performance on the JVM.
5. I/O Optimization
I/O operations can be a significant bottleneck in streaming writes. Utilizing buffered I/O streams and asynchronous I/O techniques can mitigate the overhead of I/O operations, thereby improving write performance. Additionally, employing direct memory mapping for writing Arrow data can further optimize I/O efficiency.
// Implement buffered I/O streams and asynchronous I/O techniques
// Utilize direct memory mapping for writing Arrow data
By optimizing I/O operations through buffered and asynchronous techniques, as well as leveraging direct memory mapping, the overall I/O overhead can be minimized, leading to improved streaming write performance in Apache Arrow on the JVM.
Final Considerations
Optimizing Apache Arrow on the JVM for streaming writes involves a thorough understanding of key performance considerations and best practices. By focusing on efficient memory management, buffer reuse, parallelization, data serialization, and I/O optimization, high throughput and low latency can be achieved in streaming data environments. Leveraging the discussed strategies and integrating them into streaming write implementations can lead to significant performance enhancements, making Apache Arrow a compelling choice for high-performance streaming data processing on the JVM.
In conclusion, mastering the intricacies of Apache Arrow optimization for streaming writes empowers developers to harness the full potential of this powerful in-memory columnar data format in high-throughput streaming scenarios on the JVM.
For further insights into Apache Arrow optimization and best practices, explore the official Apache Arrow documentation.
Start optimizing your Apache Arrow streaming writes on the JVM today and unleash the potential of high-performance data processing in streaming environments!