Securing HTTP APIs with Digital Signatures
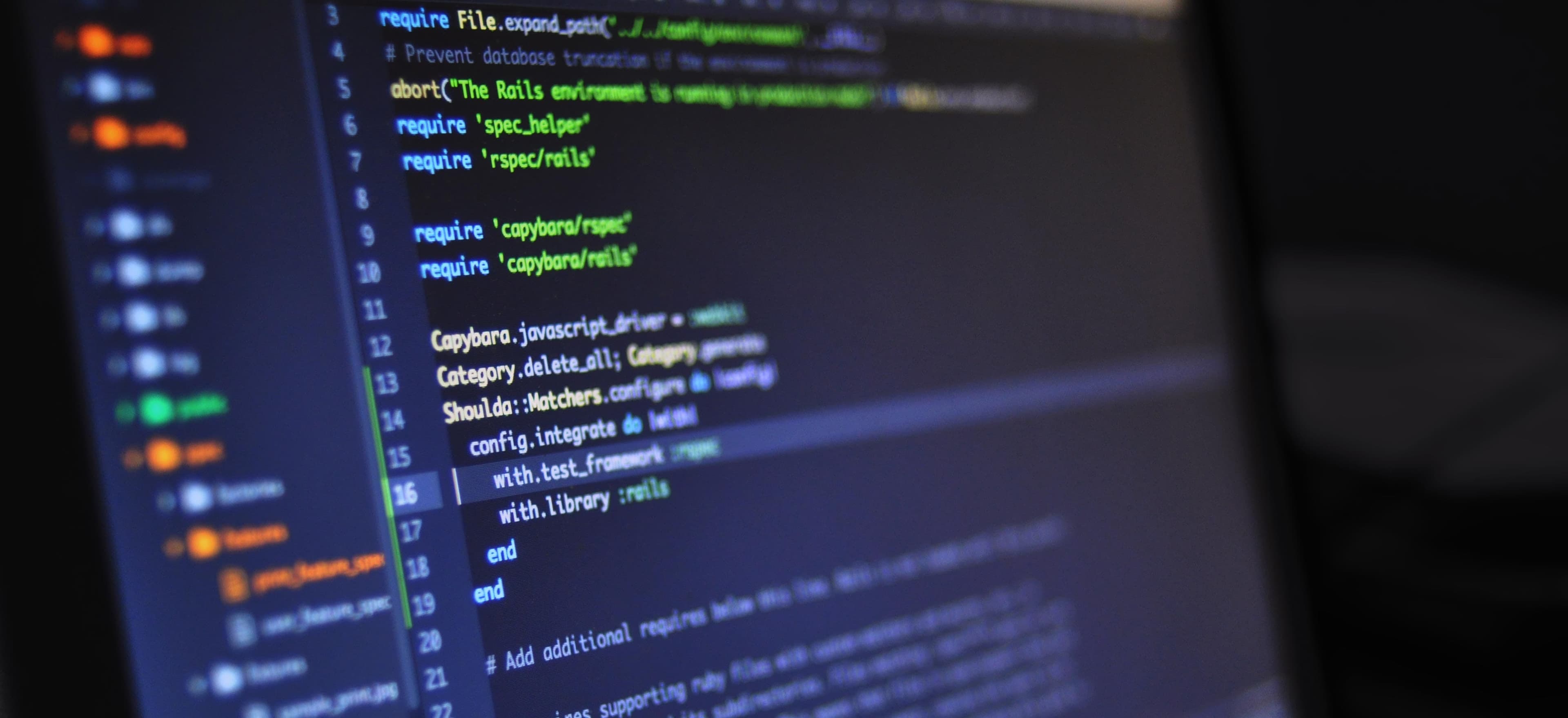
- Published on
Securing HTTP APIs with Digital Signatures
In the world of web development, securing HTTP APIs is of paramount importance. Digital signatures provide a robust method of ensuring the authenticity and integrity of data exchanged between clients and servers. In this post, we'll delve into the concept of digital signatures and explore how to secure HTTP APIs using them in Java.
Understanding Digital Signatures
Digital signatures involve the use of cryptographic techniques to sign data and verify its authenticity. This process involves a private key to sign the data and a corresponding public key to verify the signature. Digital signatures provide non-repudiation, ensuring that the sender cannot deny sending the message and that the recipient cannot deny receiving it.
Using Digital Signatures in Java
In Java, digital signatures are implemented using the java.security
package, which provides classes for the generation and verification of digital signatures. Let's explore how to use digital signatures to secure HTTP APIs in Java.
Generating a Digital Signature
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.Signature;
public class DigitalSignatureExample {
public static byte[] generateDigitalSignature(byte[] data, PrivateKey privateKey) throws Exception {
Signature signature = Signature.getInstance("SHA256withRSA");
signature.initSign(privateKey);
signature.update(data);
return signature.sign();
}
}
In the above code, we first generate a key pair using KeyPairGenerator
and then initialize the Signature
object with the private key for signing the data. Finally, we call the sign
method to obtain the digital signature.
Verifying a Digital Signature
public class DigitalSignatureExample {
public static boolean verifyDigitalSignature(byte[] data, byte[] signature, PublicKey publicKey) throws Exception {
Signature verifier = Signature.getInstance("SHA256withRSA");
verifier.initVerify(publicKey);
verifier.update(data);
return verifier.verify(signature);
}
}
Similarly, the code above demonstrates the verification of a digital signature. We initialize the Signature
object with the public key and call the verify
method to validate the signature.
Securing HTTP APIs with Digital Signatures
Now that we understand how to generate and verify digital signatures in Java, let's apply this knowledge to secure an HTTP API. For this example, we'll use the popular Spring Boot framework for building and securing the API.
Generating Key Pairs
Before we proceed to secure the API with digital signatures, we need to generate a key pair for signing and verifying the data.
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
public class KeyPairGeneratorExample {
public static KeyPair generateKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
return keyPairGenerator.generateKeyPair();
}
}
In the code above, we use the KeyPairGenerator
class to generate an RSA key pair with a key size of 2048 bits.
Securing the HTTP API
We'll now proceed to secure the HTTP API endpoints with digital signatures. We'll use Spring Boot's interceptor to intercept incoming requests, validate the digital signatures, and then allow or deny access based on the verification result.
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.security.PublicKey;
public class DigitalSignatureInterceptor extends HandlerInterceptorAdapter {
private PublicKey publicKey; // Load the public key from a secure location
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
String requestData = getRequestData(request); // Extract the data from the request
byte[] signature = getSignatureFromRequest(request); // Extract the signature from the request
if (verifyDigitalSignature(requestData.getBytes(), signature, publicKey)) {
// Signature verified successfully
return true;
} else {
// Invalid signature, deny access
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Invalid digital signature");
return false;
}
}
private String getRequestData(HttpServletRequest request) {
// Logic to extract the data from the request, such as request body or parameters
}
private byte[] getSignatureFromRequest(HttpServletRequest request) {
// Logic to extract the signature from the request, such as from headers or parameters
}
private boolean verifyDigitalSignature(byte[] data, byte[] signature, PublicKey publicKey) {
// Code for signature verification
}
}
In the code above, we create a custom interceptor DigitalSignatureInterceptor
that extends HandlerInterceptorAdapter
. In the preHandle
method, we extract the data and signature from the incoming request and then verify the digital signature using the previously defined verifyDigitalSignature
method. If the verification succeeds, the interceptor allows the request to proceed; otherwise, it denies access with an error response.
Registering the Interceptor in Spring Boot
Finally, we need to register the DigitalSignatureInterceptor
in our Spring Boot application to secure the API endpoints.
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import org.springframework.context.annotation.Configuration;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new DigitalSignatureInterceptor());
}
}
In the code above, we create a WebConfig
class that implements WebMvcConfigurer
and override the addInterceptors
method to register our DigitalSignatureInterceptor
with the interceptor registry.
Bringing It All Together
Securing HTTP APIs with digital signatures in Java is a critical aspect of building secure and reliable web applications. By implementing digital signatures and leveraging the powerful features of frameworks like Spring Boot, developers can ensure the authenticity and integrity of data exchanged over the web. With a solid understanding of digital signatures and the practical implementation demonstrated in this post, you are now well-equipped to enhance the security of your HTTP APIs.
In conclusion, implementing digital signatures for securing HTTP APIs in Java not only mitigates security risks but also fosters trust and reliability in the digital ecosystem.
By using Java's built-in cryptographic features and frameworks like Spring Boot, developers can seamlessly integrate digital signatures into their API security protocols.
To further explore digital signatures and their application in Java, consider delving into Java Cryptography Architecture (JCA) and exploring advanced cryptographic libraries such as Bouncy Castle.
Lastly, remember that robust security measures are vital in the digital era, and digital signatures stand as a stalwart defense in the realm of web security.
With this knowledge and practice, you are now empowered to fortify your HTTP APIs with digital signatures, setting a solid foundation for secure and resilient web applications.