Maximizing Code Reusability in Declarative Component Examples
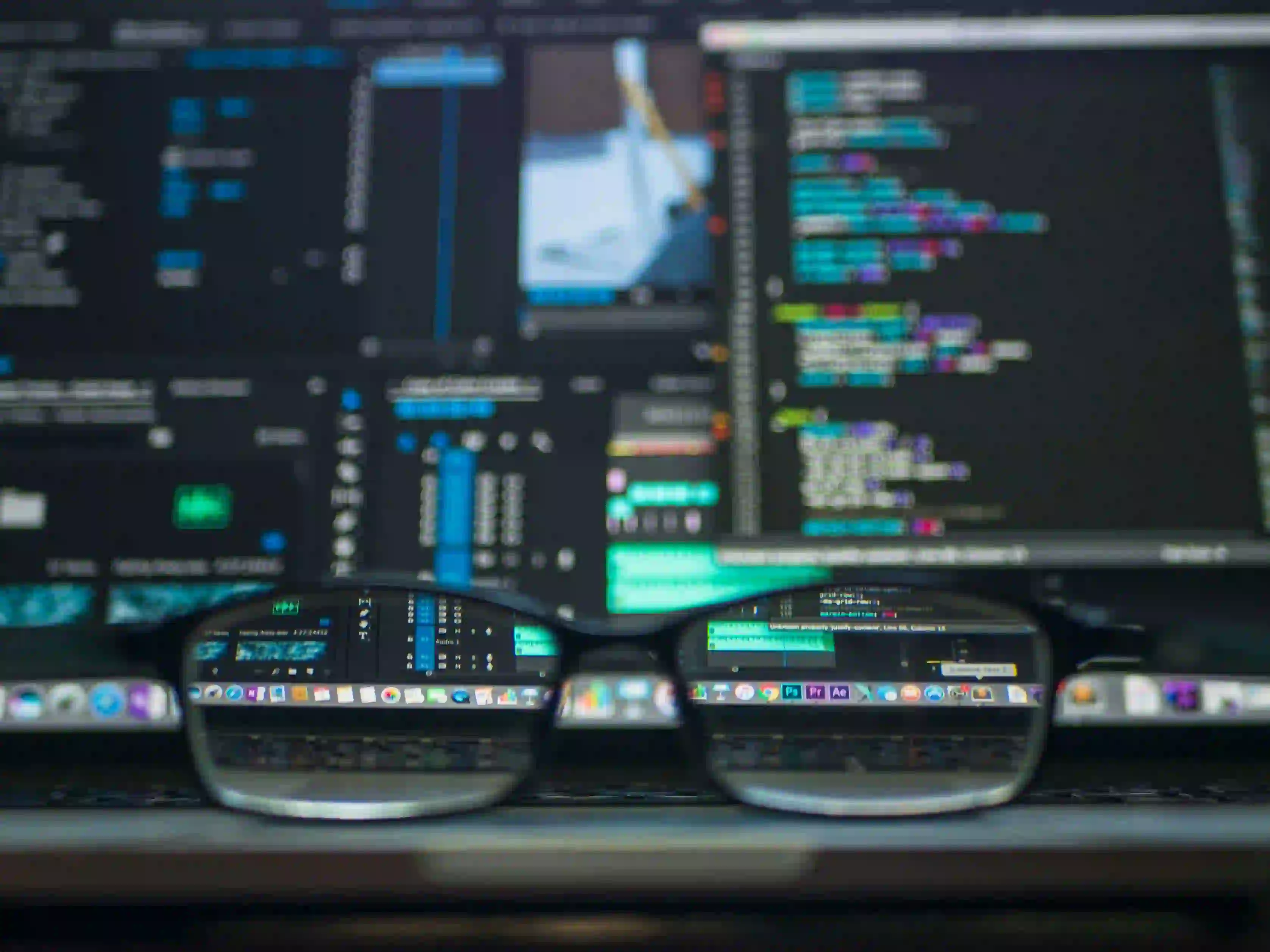
Maximizing Code Reusability in Declarative Components
In Java, maximizing code reusability is a crucial aspect of software development. Declarative components are a powerful tool for achieving this goal, as they allow you to define UI components in a descriptive manner. In this article, we will explore how to maximize code reusability in declarative components, using Java as the primary language.
Understanding Declarative Components
Declarative components allow you to define UI elements in a declarative, descriptive manner, rather than imperatively specifying every detail. This approach promotes reusability and maintainability, as components can be easily composed and reused in different parts of the application.
Leveraging Inheritance for Code Reusability
In Java, inheritance is a powerful mechanism for maximizing code reusability. By creating a base class that contains common functionality and then extending it to create specific components, you can ensure that the shared code is reused effectively.
For example, consider a base Component
class that contains generic properties and methods:
public class Component {
private String id;
private String className;
// Getters and setters for id and className
public void render() {
// Common rendering logic
}
}
Now, you can create specific components by extending the Component
class:
public class Button extends Component {
private String label;
// Getters and setters for label
@Override
public void render() {
super.render();
// Rendering logic specific to a button
}
}
By leveraging inheritance, you can reuse the common rendering logic defined in the Component
class and only focus on the specific details of the Button
component.
Utilizing Composition for Flexibility
While inheritance is a powerful tool, it has its limitations, especially when dealing with complex component hierarchies. In such cases, composition can offer more flexibility by allowing components to be composed of other components.
Consider a Panel
component that can contain multiple child components:
public class Panel extends Component {
private List<Component> children;
// Methods to add and remove children
@Override
public void render() {
super.render();
// Rendering logic for the panel and its children
}
}
With this approach, you can compose complex UI structures by combining different components within the Panel
, enabling greater flexibility and reusability.
Encapsulating Behavior with Interfaces
In addition to maximizing code reusability through class inheritance and composition, interfaces play a crucial role in encapsulating behavior. By defining interfaces for specific functionalities, you can ensure that different components can exhibit the same behavior while being completely independent in their implementation details.
For example, consider an Clickable
interface for components that are clickable:
public interface Clickable {
void onClick();
}
Now, both the Button
and Checkbox
components can implement the Clickable
interface, allowing them to be treated uniformly when it comes to click behavior.
Utilizing Generics for Flexibility
Generics enable you to create classes, interfaces, and methods that operate on objects of various types, offering flexibility and type safety. When designing declarative components, leveraging generics can lead to greater reusability by making components more adaptable to different data types.
For instance, consider a List
component that can render a list of items:
public class List<T> extends Component {
private List<T> items;
// Methods to manipulate items
@Override
public void render() {
super.render();
// Rendering logic for the list and its items
}
}
By using generics, the List
component becomes agnostic to the type of items it contains, allowing it to be reused for various data types without sacrificing type safety.
Lessons Learned
In Java, declarative components offer a powerful way to maximize code reusability by promoting a descriptive and composable approach to UI development. By leveraging inheritance, composition, interfaces, and generics, you can create flexible and reusable components that form the building blocks of complex UI structures. This approach not only leads to more maintainable code but also fosters a modular and scalable architecture, aligning with best practices in software development.
By understanding and applying these principles, Java developers can enhance their ability to create reusable and maintainable code, ultimately leading to more efficient and robust applications.
For further reading on Java declarative component development, you may find the JavaFX documentation on Creating a Custom Control and the official Java documentation for Generic Types to be valuable resources.