Optimizing Application Lifecycle Management for Efficiency
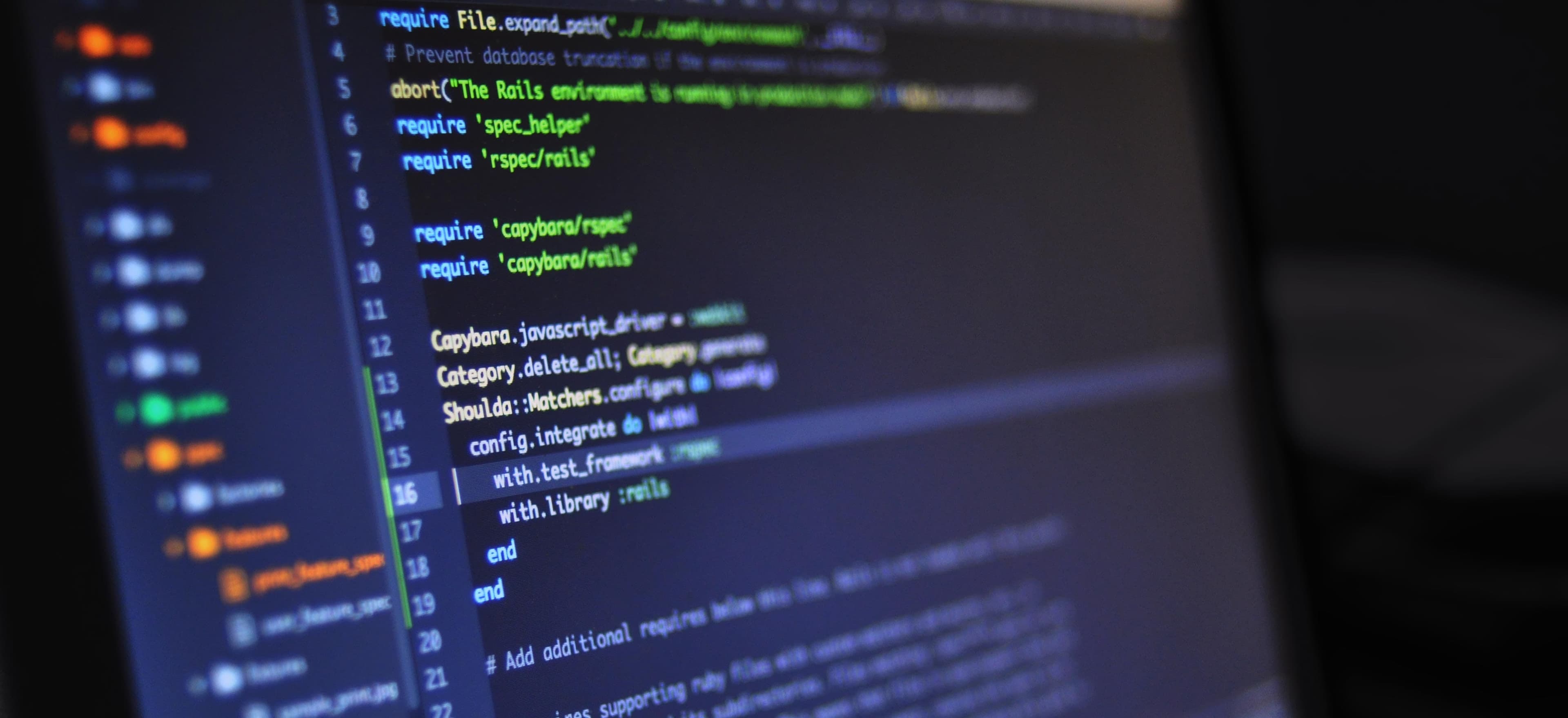
- Published on
Optimizing Application Lifecycle Management for Efficiency
In the world of software development, Application Lifecycle Management (ALM) plays a crucial role in ensuring the seamless flow of processes from the initial concept to the final release. It encompasses everything from the conception of an idea, through development, testing, deployment, and maintenance. Efficient ALM practices can significantly impact the success of a project by ensuring timely delivery, high quality, and cost-effectiveness.
The Role of ALM in Software Development
ALM involves the management of every phase in the software development process, including requirements gathering, design, coding, testing, deployment, and maintenance. Effective ALM ensures that the software is delivered on time, within budget, and with the anticipated features and performance. It also facilitates collaboration among different teams involved in the development process, such as developers, testers, and operations personnel.
Java's Role in ALM
Java is a popular programming language widely used in enterprise software development. Its platform independence, strong ecosystem, and robustness make it an excellent choice for building scalable and reliable software applications. When it comes to ALM, Java offers a plethora of tools, frameworks, and best practices to streamline the development, testing, and deployment processes.
Streamlining ALM with Java
1. Version Control with Git
Version control is a fundamental aspect of ALM, allowing teams to collaborate efficiently and track changes in the codebase. Git, with its distributed nature and powerful branching model, has become the de facto standard for version control. Integrating Git with Java projects using tools like GitKraken or SourceTree can enhance collaboration and streamline the development process.
// Sample Git integration using JGit library in Java
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
public class GitIntegration {
public static void main(String[] args) {
try (Git git = Git.open(new File("/path/to/repo"))) {
// use JGit API to perform version control operations
} catch (IOException | GitAPIException e) {
e.printStackTrace();
}
}
}
2. Continuous Integration with Jenkins
Continuous Integration (CI) is vital for ensuring that code changes are regularly and automatically tested and integrated into the main codebase. Jenkins, a popular open-source automation server, provides extensive support for building, deploying, and automating Java projects. Integrating Jenkins with Java projects allows teams to automate repetitive tasks, such as running tests and generating build artifacts, leading to increased productivity and quality.
3. Automated Testing with JUnit
Unit testing is an integral part of the development process, and JUnit is the de facto standard for unit testing in Java. Writing unit tests using JUnit ensures that individual units of code are tested comprehensively, leading to robust and maintainable code. Integrating JUnit tests into the build process and CI pipeline guarantees that any code changes are thoroughly tested, reducing the likelihood of introducing bugs or regressions.
// Example of a JUnit test case
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MyMathUtilsTest {
@Test
public void testAdd() {
assertEquals(5, MyMathUtils.add(2, 3));
}
}
4. Containerization with Docker
Docker has revolutionized the way applications are developed, shipped, and run. By creating lightweight, portable containers for applications, Docker streamlines the deployment process and ensures consistency across different environments. Containerizing Java applications with Docker allows for easy deployment, scaling, and management, improving the overall efficiency of the deployment phase in ALM.
5. Monitoring and Logging with ELK Stack
Effective monitoring and logging are crucial for identifying and resolving issues in production environments. The ELK (Elasticsearch, Logstash, Kibana) stack provides a powerful solution for collecting, searching, analyzing, and visualizing log and operational data. Integrating the ELK stack with Java applications enables teams to gain valuable insights into the application's behavior and performance, facilitating proactive issue resolution and optimization.
The Bottom Line
In conclusion, optimizing Application Lifecycle Management for efficiency is essential for the success of software development projects. Java, with its comprehensive set of tools, libraries, and frameworks, provides ample support for streamlining ALM processes. By embracing version control, continuous integration, automated testing, containerization, and monitoring, teams can enhance their productivity, code quality, and deployment efficiency. Embracing these best practices not only improves the development workflow but also contributes to the overall success of the software project.
By harnessing the power of Java and integrating it with modern ALM practices, teams can ensure smooth and efficient software delivery, ultimately leading to greater customer satisfaction and business success.
For further insights into optimizing ALM with Java, check out this In-depth Guide to Application Lifecycle Management. Additionally, the official Java documentation provides comprehensive information on utilizing Java for efficient application development and management.