Overcoming Challenges in Continuous Deployment
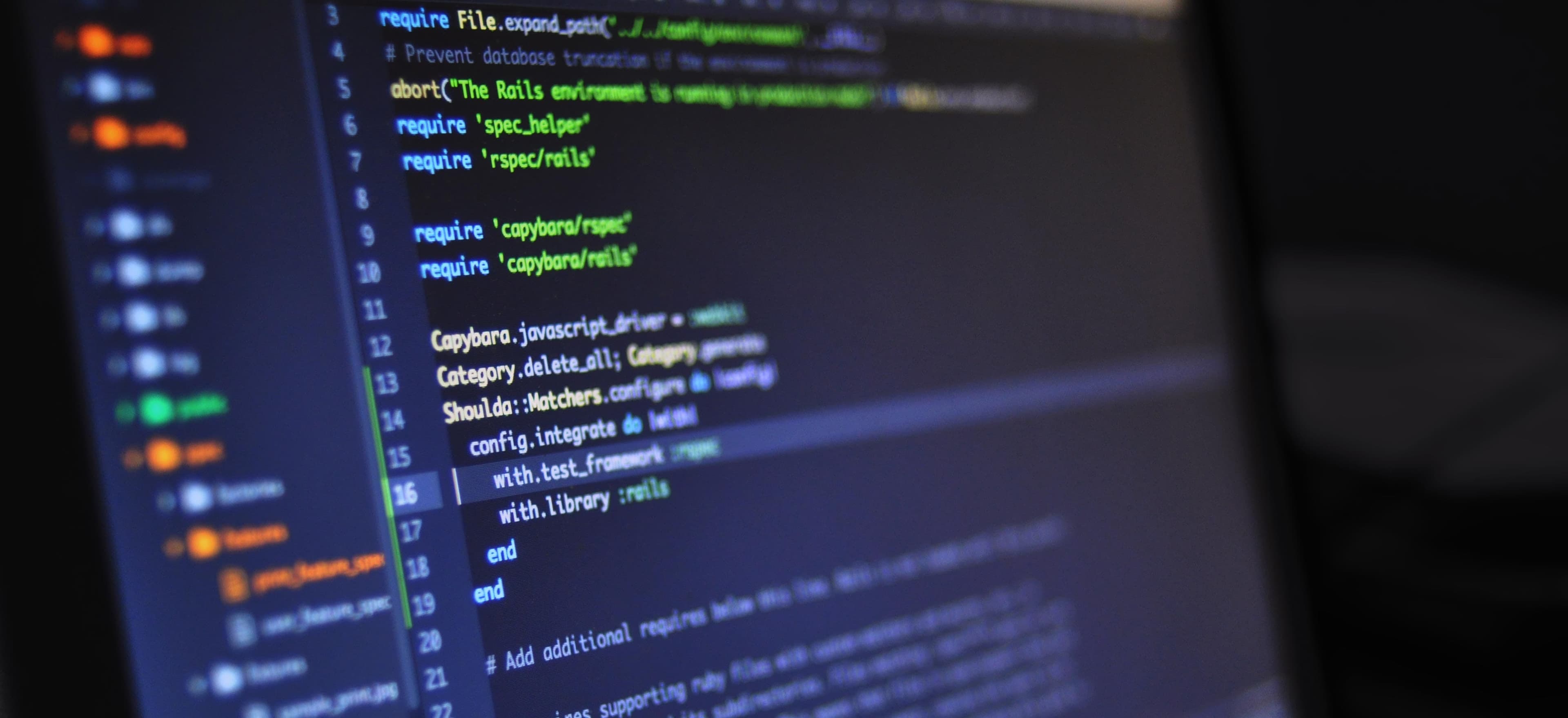
- Published on
Embracing Continuous Deployment in Java: Overcoming Challenges
In the fast-paced world of software development, continuous deployment has become a crucial practice for ensuring that changes are delivered to production in a reliable and efficient manner. For Java applications, implementing continuous deployment comes with its own set of challenges, from managing dependencies to automating complex build processes. In this post, we will explore some of the common challenges encountered when implementing continuous deployment for Java applications and discuss strategies for overcoming them.
Challenge 1: Managing Dependencies
Problem: Managing dependencies in Java projects can be complex, especially when dealing with a large number of external libraries. There is a risk of dependency conflicts, version mismatches, and compatibility issues.
Solution: Utilize a robust build automation tool like Apache Maven or Gradle. These tools not only handle the dependency management but also provide a standardized way to define, package, and distribute Java artifacts. By using a dependency management tool, teams can ensure that all developers are using the same libraries and versions, reducing the risk of compatibility issues.
// Example of Maven dependency management in pom.xml
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.2.8.RELEASE</version>
</dependency>
<!-- other dependencies -->
</dependencies>
Why: Centralizing dependency management with a tool like Maven ensures that all developers are working with the same set of dependencies, reducing the chances of runtime issues due to version mismatches.
Challenge 2: Unit Testing and Code Coverage
Problem: With continuous deployment, ensuring the quality of the code is crucial. Unit tests must be comprehensive, and code coverage should be sufficient to catch potential issues before they reach production.
Solution: Integrate a robust unit testing framework like JUnit and measure code coverage using tools like JaCoCo or Cobertura. By enforcing a minimum code coverage threshold and running unit tests as part of the build pipeline, teams can maintain high code quality throughout the development process.
// Example of a JUnit test case
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
Why: Unit testing ensures that individual components of the Java application work as expected, while code coverage measurement identifies areas of the code that lack test coverage, enabling teams to improve overall code quality.
Challenge 3: Automated Build and Deployment
Problem: Manually building and deploying Java applications is time-consuming and error-prone. Automating the build and deployment process is essential for continuous deployment.
Solution: Leverage continuous integration and deployment tools like Jenkins, CircleCI, or Travis CI to automate the build, test, and deployment pipelines. By defining build scripts and deployment configurations in version-controlled files (e.g., Jenkinsfile or .travis.yml), teams can establish consistent and repeatable deployment processes.
// Example of a Jenkinsfile for pipeline automation
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'scp target/application.jar user@production-server:/deployments/'
}
}
}
}
Why: Automation ensures that every code change goes through a standardized pipeline, reducing the chances of human error and ensuring consistent deployments.
Challenge 4: Managing Configuration
Problem: Configuration management for different environments (e.g., development, staging, production) can be challenging, leading to issues such as hardcoded configuration values and environment-specific bugs.
Solution: Utilize environment-specific configuration files (e.g., application.properties) and leverage tools like Spring Boot's externalized configuration to manage environment-specific settings. By decoupling configuration from the application code, teams can easily manage and maintain configurations for different environments.
// Example of Spring Boot configuration
// application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=user
spring.datasource.password=pass
Why: Separating configuration from the code simplifies the process of managing environment-specific configurations and avoids hardcoded values, making the application more portable across different environments.
Challenge 5: Canary Deployments and Rollbacks
Problem: Rolling back a faulty deployment and gradually rolling out new features (canary deployments) are essential parts of continuous deployment, but they can be challenging to implement in Java applications.
Solution: Utilize feature flagging and A/B testing frameworks like Togglz or LaunchDarkly to enable canary deployments and gradual feature rollouts. By controlling feature toggles through configuration, teams can safely roll out and roll back features without impacting the entire user base.
// Example of feature flag usage with Togglz
if (MyFeatures.FEATURE_ONE.isActive()) {
// new feature code
} else {
// old feature code
}
Why: Implementing feature flags allows teams to gradually roll out features and roll them back if necessary, minimizing the impact of potential issues on the overall user base.
In conclusion, implementing continuous deployment for Java applications comes with its own set of challenges, but with the right tools and strategies, these challenges can be overcome. By streamlining dependency management, enforcing code quality practices, automating the build and deployment process, and effectively managing configurations and feature rollouts, Java teams can embrace continuous deployment with confidence, delivering high-quality changes to production with minimal risk.
By embracing a combination of robust tools and best practices, Java teams can navigate the complexities of continuous deployment and reap the benefits of delivering reliable and frequent updates to their applications.
So let's embrace the challenges, equip ourselves with the right tools, and elevate our Java applications to a new level of continuous deployment excellence!
Checkout our other articles