Maximizing Proxy Performance in JavaOne 2014
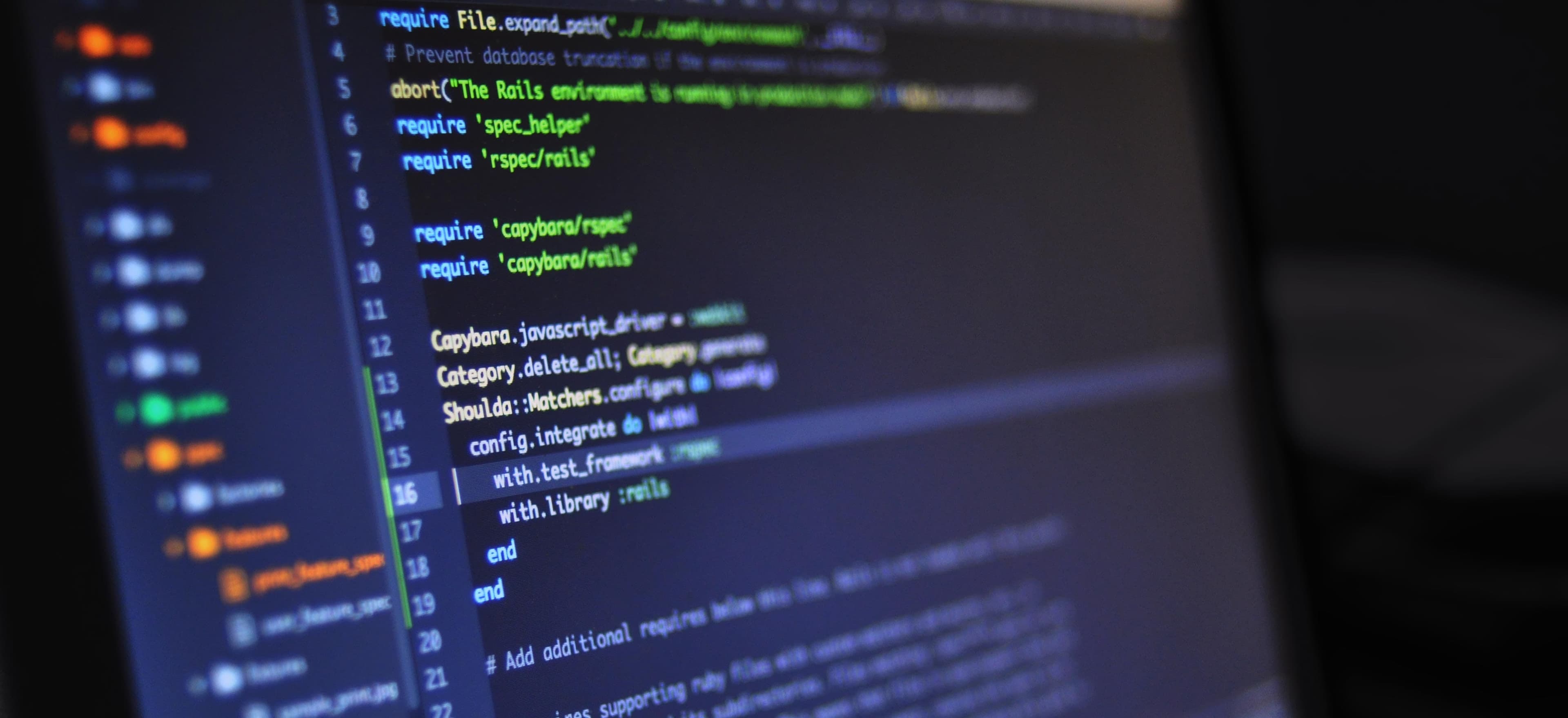
- Published on
Maximizing Proxy Performance in Java
In the age of the internet, proxies play a crucial role in enhancing security, improving performance, and providing access to region-restricted content. Java, being a versatile language, offers various tools and libraries for creating and optimizing proxies. In this blog post, we will discuss techniques to maximize proxy performance in Java, focusing on key areas such as connection management, concurrency, and caching.
Efficient Connection Management
When creating a proxy in Java, efficient connection management is crucial for maximizing performance. The Apache HttpClient library is a popular choice for managing HTTP connections in Java.
CloseableHttpClient httpClient = HttpClients.custom()
.setMaxConnTotal(100)
.setMaxConnPerRoute(20)
.build();
In the above code snippet, we configure the maximum total connections and maximum connections per route. This prevents resource exhaustion and allows for proper utilization of connections, ultimately improving proxy performance.
Concurrency Considerations
Concurrency plays a vital role in proxy performance optimization. Java provides various constructs, such as ExecutorService
, to manage concurrent tasks efficiently.
ExecutorService executorService = Executors.newFixedThreadPool(10);
By using a fixed thread pool, we can control the concurrency level, preventing resource contention and excessive thread creation. This is especially important when dealing with a large number of concurrent proxy requests.
Leveraging Caching
Caching is another essential aspect of proxy performance optimization. In Java, libraries like Ehcache provide robust caching mechanisms that can be integrated into proxy implementations.
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("proxyCache", CacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, String.class, ResourcePoolsBuilder.heap(100)))
.build(true);
The above code snippet demonstrates the configuration of a simple, in-memory cache using Ehcache. By caching frequently accessed data, we can minimize redundant requests and improve overall proxy performance.
Asynchronous Processing
In scenarios where the proxy needs to handle asynchronous tasks, Java's CompletableFuture can be leveraged to improve performance.
CompletableFuture<String> futureResult = CompletableFuture.supplyAsync(() -> {
// Perform asynchronous proxy task
return "Task completed";
}, executorService);
By utilizing CompletableFuture, we can delegate non-blocking tasks to separate threads, effectively utilizing resources and improving overall proxy responsiveness.
Optimizing Network IO
Java's NIO (New I/O) package provides a way to perform high-performance I/O operations, which is crucial for proxy servers dealing with a large number of concurrent connections.
Selector selector = Selector.open();
ServerSocketChannel serverSocket = ServerSocketChannel.open();
serverSocket.bind(new InetSocketAddress(8080));
serverSocket.configureBlocking(false);
serverSocket.register(selector, SelectionKey.OP_ACCEPT);
In the above code, we create a non-blocking server socket channel using Java's NIO package, allowing for efficient handling of network I/O operations in the proxy server.
Monitoring and Profiling
To truly maximize proxy performance, it's essential to continuously monitor and profile the proxy application. Java provides tools such as JVisualVM and Java Mission Control, which offer insights into memory usage, CPU utilization, and thread management.
By analyzing the profiling data, we can identify performance bottlenecks and fine-tune the proxy implementation for optimal performance.
To Wrap Things Up
Maximizing proxy performance in Java requires a combination of efficient connection management, concurrency control, caching strategies, asynchronous processing, network I/O optimization, and continuous monitoring. By incorporating these techniques and leveraging Java's robust toolset, developers can build high-performance proxies capable of meeting the demands of modern applications.
In conclusion, optimizing proxy performance in Java involves a meticulous approach that considers various aspects of application design and resource utilization. By implementing the discussed techniques and staying abreast of performance monitoring best practices, developers can ensure that their Java proxies operate at peak efficiency.
For further reading and exploration, consider the following resources from the Java ecosystem:
I hope this blog post provides valuable insights into maximizing proxy performance in Java. Happy coding!