Troubleshooting MongoDB Connection Issues
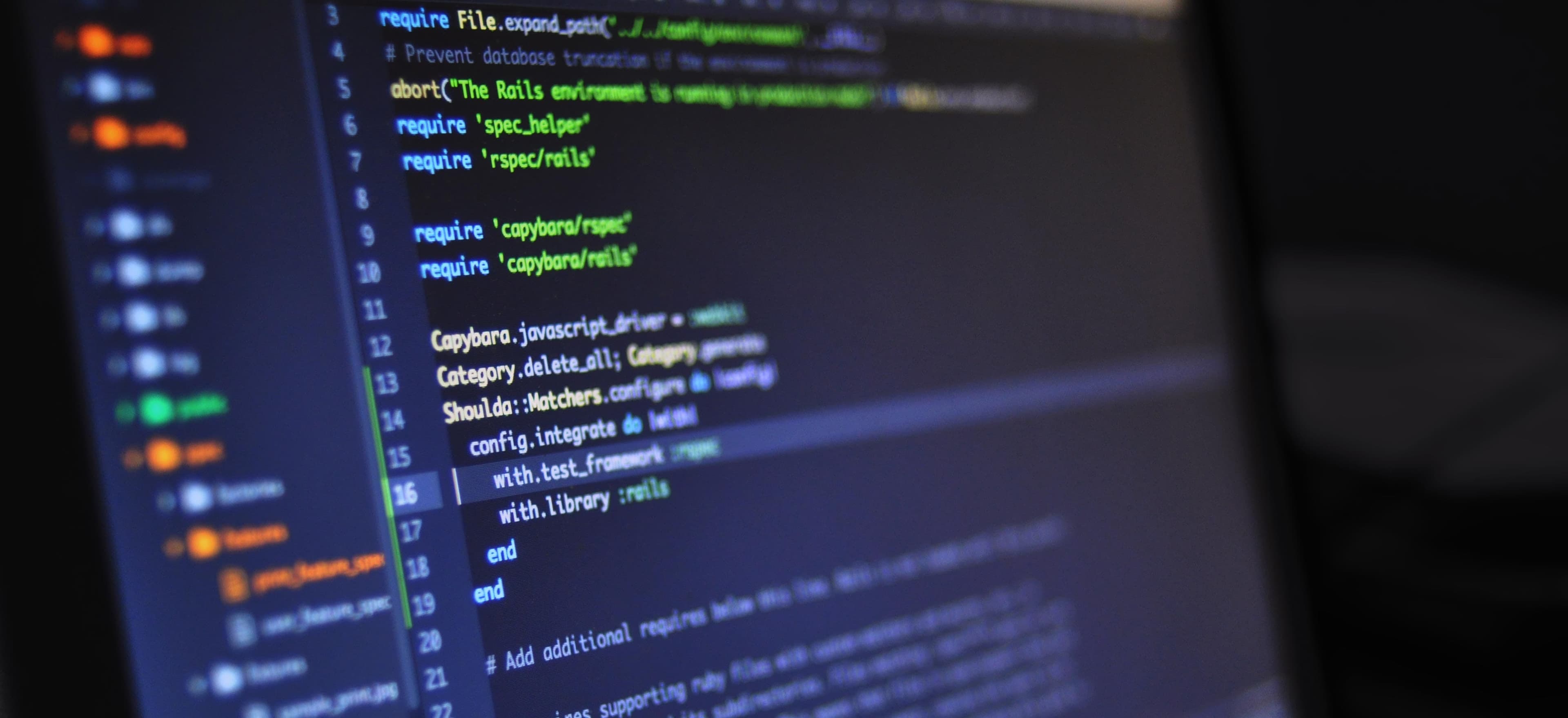
- Published on
Troubleshooting MongoDB Connection Issues
When working with MongoDB in a Java application, connection issues can be a common obstacle. Whether you are encountering connection timeouts, authentication failures, or general connectivity problems, effective troubleshooting is essential for maintaining a healthy and responsive application. In this post, we will explore common MongoDB connection issues in the context of a Java application and provide practical solutions for resolving them.
Checking MongoDB Server Status
Before delving into Java-specific troubleshooting, it's crucial to ensure that your MongoDB server is up and running. You can use the mongostat
command to check the server's current status, including connections, active operations, and queued operations. If the server is unresponsive or experiencing high load, it may be the root cause of your connection issues.
Verifying MongoDB Java Driver Dependency
In a Java application, the MongoDB Java driver serves as the bridge between your code and the MongoDB database. When encountering connection problems, the first step is to verify that you have included the correct MongoDB Java driver dependency in your project.
// Maven dependency for MongoDB Java driver
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongodb-driver-sync</artifactId>
<version>4.4.3</version>
</dependency>
Ensure that your pom.xml
or build configuration references the appropriate version of the MongoDB Java driver. Using an outdated or incompatible driver version can lead to connectivity issues and unexpected behavior.
Configuring Connection Parameters
When establishing a connection to MongoDB from your Java application, it's essential to configure the connection parameters correctly. The ConnectionString
class in the MongoDB Java driver provides a flexible way to specify connection options, including the host, port, and authentication details.
// Configure MongoDB connection
ConnectionString connectionString = new ConnectionString("mongodb://localhost:27017/?authSource=admin");
MongoClientSettings settings = MongoClientSettings.builder()
.applyConnectionString(connectionString)
.build();
MongoClient mongoClient = MongoClients.create(settings);
MongoDatabase database = mongoClient.getDatabase("mydb");
In the example above, the connection string includes the host and port of the MongoDB server, as well as the authentication source. Incorrect configuration of these parameters can result in connection failures or authentication errors.
Handling Connection Timeouts
Connection timeouts can occur when the MongoDB server is unreachable or the network latency is high. In a Java application, you can set the connection timeout using the ServerSettings
class to control the maximum time for establishing a connection.
// Set connection timeout
ServerSettings serverSettings = ServerSettings.builder()
.connectTimeout(30, TimeUnit.seconds)
.build();
MongoClientSettings settings = MongoClientSettings.builder()
.applyConnectionString(connectionString)
.applyToServerSettings(builder -> builder.applySettings(serverSettings))
.build();
MongoClient mongoClient = MongoClients.create(settings);
By specifying an appropriate connection timeout, you can prevent the application from waiting indefinitely for a connection to be established, allowing it to gracefully handle scenarios where the server is unresponsive.
Handling Authentication Failures
When authentication fails while connecting to MongoDB from your Java application, it's essential to verify the correctness of the authentication credentials provided in the connection string.
// Configure MongoDB authentication
ConnectionString connectionString = new ConnectionString("mongodb://username:password@localhost:27017/?authSource=admin");
// ...
Ensure that the username, password, and authentication source in the connection string are accurate and match the credentials required by the MongoDB server. Authentication failures can occur due to mismatched credentials, leading to connectivity issues.
Network Security Considerations
Firewalls, network restrictions, and security configurations can impact the connectivity between your Java application and the MongoDB server. If you are encountering connection problems, particularly in a cloud or network-restricted environment, ensure that the necessary network ports are open and accessible for MongoDB traffic.
Connection Pooling and Resource Management
In a production Java application, efficient management of MongoDB connections is crucial for performance and resource optimization. The MongoDB Java driver includes built-in connection pooling, allowing you to reuse connections and minimize the overhead of establishing new connections for each operation.
// Configure connection pooling
ConnectionPoolSettings poolSettings = ConnectionPoolSettings.builder()
.maxSize(50)
.maxWaitQueueSize(100)
.build();
MongoClientSettings settings = MongoClientSettings.builder()
.applyConnectionString(connectionString)
.applyToConnectionPoolSettings(builder -> builder.applySettings(poolSettings))
.build();
MongoClient mongoClient = MongoClients.create(settings);
By configuring the connection pool settings, you can control the maximum number of connections in the pool and define the behavior when the pool is exhausted, preventing resource exhaustion and improving the application's responsiveness.
Closing Remarks
Troubleshooting MongoDB connection issues in a Java application requires meticulous attention to configuration, networking, and resource management. By verifying the MongoDB server status, checking the Java driver dependency, configuring connection parameters, handling timeouts and authentication failures, and considering network security and connection pooling, you can effectively resolve and prevent connectivity problems.
Remember that understanding the nuances of MongoDB connectivity in a Java context empowers you to build robust, reliable, and high-performance applications that harness the full potential of the MongoDB database.
For further insights on MongoDB Java driver, you can refer to the official MongoDB Java Developer Hub.