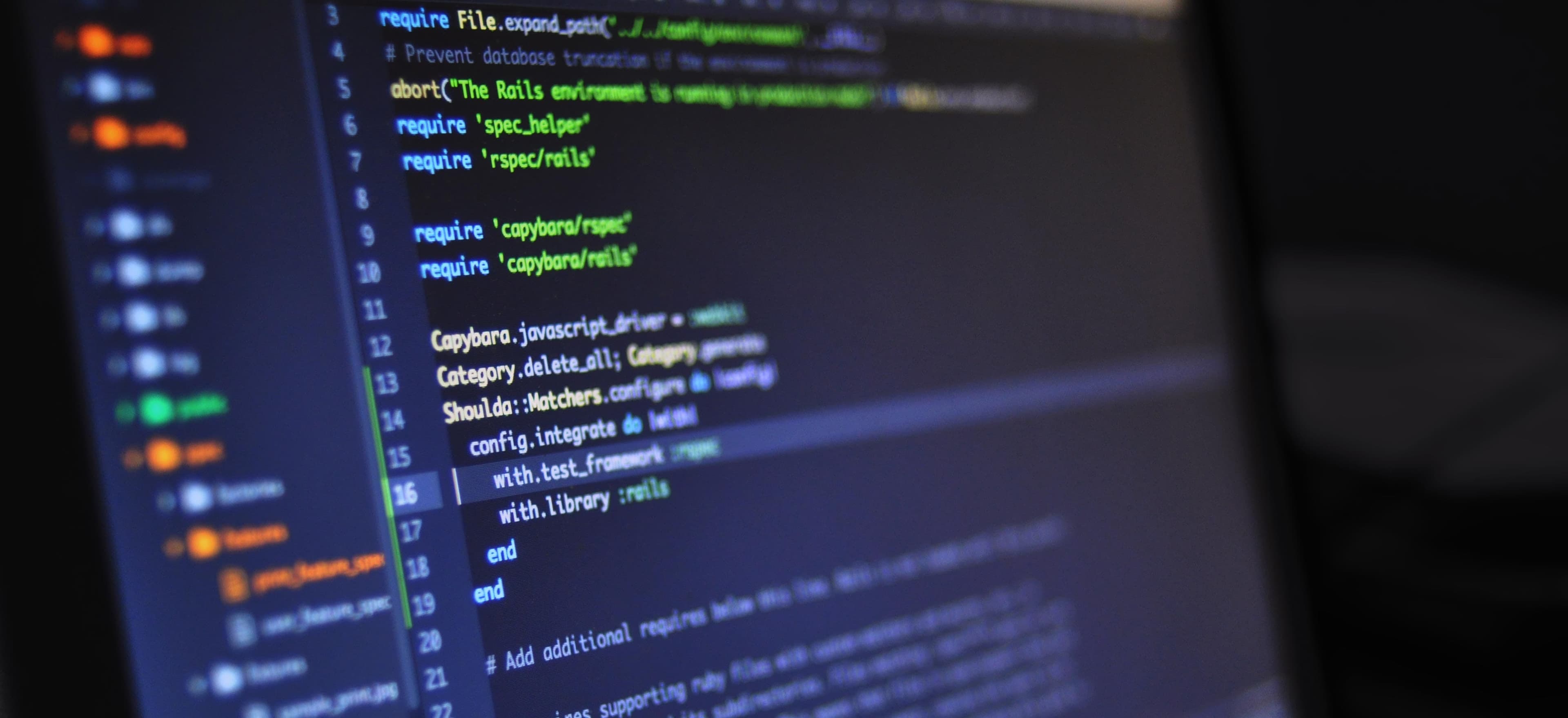
- Published on
Understanding Side Effects in Java
When writing code in Java, it's vital to grasp the concept of side effects. In programming, a side effect occurs when a function modifies state outside of its scope. This can lead to unexpected behavior, bugs, and an overall lack of clarity in the code.
The Importance of Avoiding Side Effects
Side effects can make code harder to reason about, test, and maintain. By minimizing side effects, code becomes more predictable, deterministic, and easier to understand. This is particularly important in functional programming, where immutability and pure functions are key principles.
Immutability in Java
Java, being an object-oriented language, does not enforce immutability by default. This means that objects can be modified freely unless explicitly prevented. Utilizing immutable objects in Java can help avoid side effects. An immutable object is one whose state cannot be modified after it is created.
public class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In the example above, the ImmutablePerson
class is immutable as its state cannot be altered after construction.
Pure Functions in Java
Pure functions are another tool for avoiding side effects in Java. A pure function always produces the same output for the same input and has no side effects. This makes them easy to reason about and test.
public class PureFunction {
public int add(int a, int b) {
return a + b;
}
}
In the add
method above, the function returns the sum of the input parameters without modifying any external state, making it a pure function.
Managing State with Java's Stream API
The Stream API introduced in Java 8 provides a powerful way to process collections of objects. It encourages a functional programming style by allowing operations to be performed on data without modifying the original collection.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(n -> n * 2)
.sum();
System.out.println(sum); // Output: 12
In the code snippet above, the original numbers
collection remains unchanged as the stream operations are performed. This approach minimizes side effects and facilitates clearer code.
Leveraging Immutability and Pure Functions Together
By combining immutability with pure functions, Java code can become more resilient to side effects. Immutable objects can be safely passed to pure functions, and the output can be relied upon without concern for unexpected changes to state.
public class ShoppingCart {
private final List<Item> items;
public ShoppingCart(List<Item> items) {
this.items = Collections.unmodifiableList(new ArrayList<>(items));
}
public List<Item> getItems() {
return new ArrayList<>(items);
}
public double calculateTotal() {
return items.stream()
.mapToDouble(Item::getPrice)
.sum();
}
}
In the ShoppingCart
example, immutability is enforced for the items
list, and the calculateTotal
method is a pure function, as it operates only on its input parameters without causing any side effects.
Using Functional Interfaces in Java
Functional interfaces, introduced in Java 8, play a crucial role in functional programming. An interface with a single abstract method is considered a functional interface, and can be used to leverage lambda expressions and method references.
@FunctionalInterface
interface MathOperation {
int calculate(int a, int b);
}
The MathOperation
interface above is an example of a functional interface. It specifies a single abstract method calculate
, making it suitable for lambda expressions or method references.
Leveraging Java's Functional Programming Libraries
Java provides a variety of functional programming libraries that can aid in writing code with reduced side effects. Libraries like Vavr and functional-utils offer additional functionalities such as persistent collections and advanced functional programming constructs.
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
int sum = numbers.filter(n -> n % 2 == 0)
.map(n -> n * 2)
.sum();
System.out.println(sum); // Output: 12
In the code snippet above, the Vavr library's List
offers immutability and functional operations, reducing the potential for side effects in the code.
Key Takeaways
Understanding and mitigating side effects is crucial in creating reliable, maintainable, and testable Java code. By embracing immutability, pure functions, Java's Stream API, functional interfaces, and functional programming libraries, developers can minimize side effects and build more robust applications. Following these practices not only enhances code quality but also aligns with the principles of functional programming, making codebases more resilient and easier to work with.