Optimizing Google Trends Data Visualization
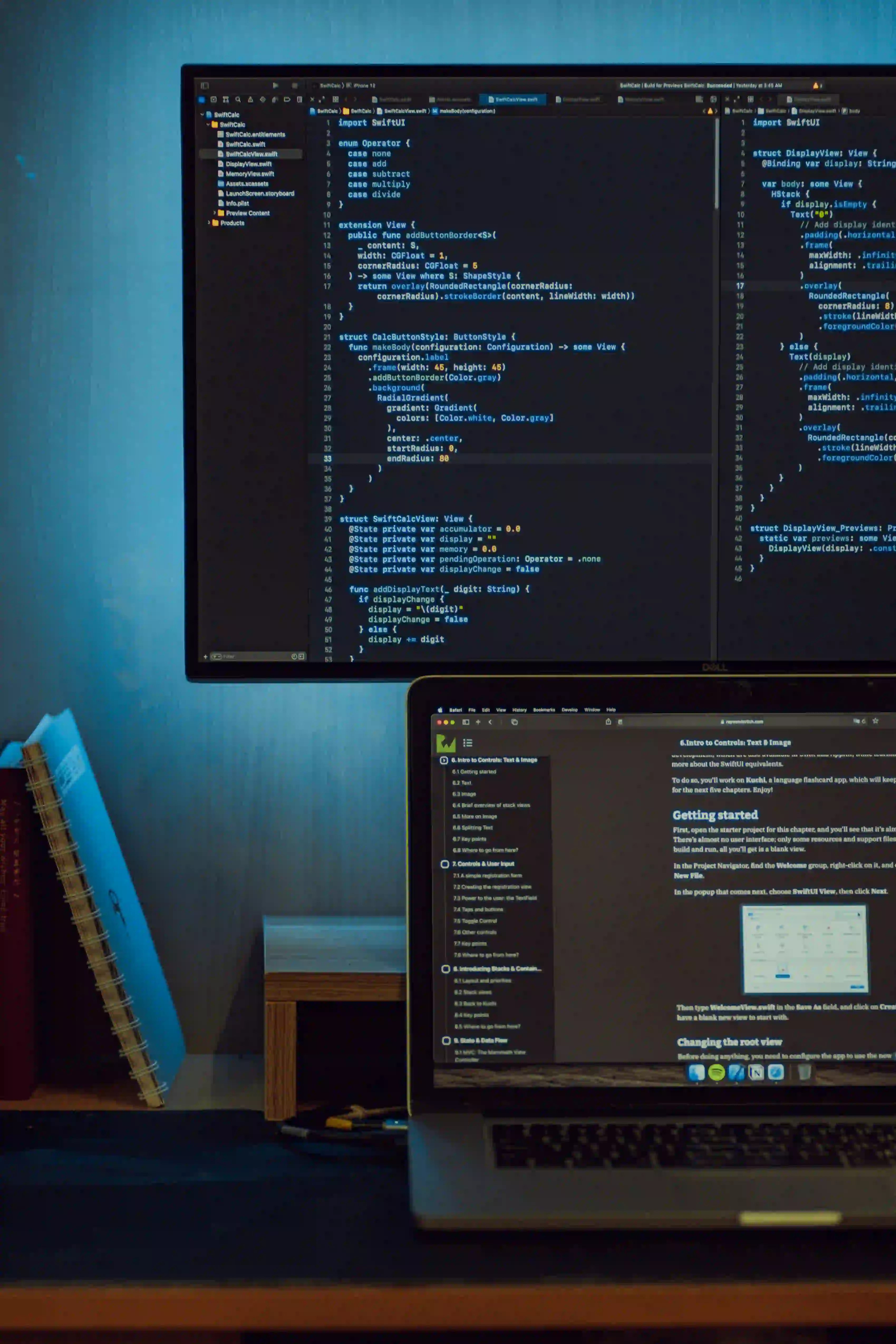
Understanding the importance of data visualization
In today's world, data is generated at an unprecedented pace. With a surge in the volume and variety of data, the ability to derive meaningful insights from it has become crucial. Data visualization plays a pivotal role in this process. It transforms raw data into visually appealing and easily understandable representations, allowing businesses and individuals to make informed decisions based on the insights gained.
Leveraging Google Trends as a data source
Google Trends is a powerful tool that provides insights into the popularity of search queries on Google. It allows users to analyze and compare the popularity of search terms over time and across different regions. Leveraging Google Trends data can offer valuable insights into user interests, trends, and market dynamics. Visualizing this data effectively can unlock its full potential.
The power of Java in data visualization
Java, with its rich ecosystem and powerful libraries, is a versatile language for data visualization. Its libraries, such as JFreeChart, JavaFX, and Apache ECharts, provide extensive capabilities for creating captivating visual representations of data. In this blog post, we will explore how Java can be employed to optimize the visualization of Google Trends data.
Setting up the project
Before diving into visualization, let's set up our Java project. We'll use Maven as the build tool and JFreeChart as the visualization library. First, we need to add the JFreeChart dependency to the pom.xml
file:
<dependency>
<groupId>org.jfree</groupId>
<artifactId>jfreechart</artifactId>
<version>1.5.3</version>
</dependency>
Next, we'll create a simple Java class to fetch Google Trends data using the Google Trends API, parse the response, and prepare it for visualization.
Fetching and preparing Google Trends data
In the following code snippet, we use the HttpClient
from Java 11 to make a request to the Google Trends API and fetch the popularity data for a specific search term over a period of time. We then process the JSON response to extract the relevant data points for visualization.
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import org.json.JSONArray;
public class GoogleTrendsDataFetcher {
public JSONArray fetchTrendsData(String searchTerm, String timeframe) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://trends.google.com/trends/api/widgetdata/multiline?hl=en-US&tz=-120&req={\"time\":\"" + timeframe + "\",\"resolution\":\"DAY\",\"locale\":\"en-US\",\"comparisonItem\":[{\"geo\":{\"country\":\"US\"},\"complexKeywordsRestriction\":{\"keyword\":[{\"type\":\"BROAD\",\"value\":\"" + searchTerm + "\"}]}a3}]\"}"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
String jsonData = response.body();
// Extract and return the data array from the JSON response
return new JSONArray(jsonData.substring(jsonData.indexOf('[')));
}
}
In this code, we are making an HTTP request to the Google Trends API, specifying the search term and the timeframe for which we want to retrieve the data. The JSON response is then parsed to extract the data array, which contains the popularity values over time.
Visualizing Google Trends data with JFreeChart
Now that we have fetched and processed the Google Trends data, it's time to visualize it using JFreeChart. JFreeChart offers a wide range of chart types and customization options, making it a suitable choice for visualizing time-series data like Google Trends.
Let's create a simple line chart using JFreeChart to visualize the popularity of a search term over time.
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.time.TimeSeries;
import org.jfree.data.time.TimeSeriesCollection;
import org.jfree.ui.ApplicationFrame;
import org.jfree.ui.RefineryUtilities;
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class GoogleTrendsChart extends ApplicationFrame {
public GoogleTrendsChart(String title, TimeSeriesCollection dataset) {
super(title);
JFreeChart chart = createChart(dataset);
ChartPanel chartPanel = new ChartPanel(chart);
chartPanel.setPreferredSize(new Dimension(800, 400));
setContentPane(chartPanel);
}
private JFreeChart createChart(TimeSeriesCollection dataset) {
JFreeChart chart = ChartFactory.createTimeSeriesChart(
"Google Trends Data Visualization",
"Date",
"Popularity",
dataset,
false,
true,
false
);
return chart;
}
public static void main(String[] args) {
String searchTerm = "Java Programming";
String timeframe = "now 7-d";
GoogleTrendsDataFetcher dataFetcher = new GoogleTrendsDataFetcher();
JSONArray trendsData;
try {
trendsData = dataFetcher.fetchTrendsData(searchTerm, timeframe);
TimeSeries series = new TimeSeries(searchTerm);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for (Object entry : trendsData.getJSONArray(0)) {
JSONArray dataPoint = (JSONArray) entry;
Date date = sdf.parse(dataPoint.get(0).toString());
double value = Double.parseDouble(dataPoint.get(1).toString());
series.add(new org.jfree.data.time.Day(date), value);
}
TimeSeriesCollection dataset = new TimeSeriesCollection(series);
GoogleTrendsChart chart = new GoogleTrendsChart("Google Trends Data Visualization", dataset);
chart.pack();
RefineryUtilities.centerFrameOnScreen(chart);
chart.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this code, we use JFreeChart to create a time series chart that visualizes the popularity of the search term "Java Programming" over the last 7 days. We fetch the Google Trends data using the previously defined GoogleTrendsDataFetcher
class and populate a TimeSeries
with the retrieved data points. We then create a TimeSeriesCollection
and pass it to the GoogleTrendsChart
constructor to display the chart.
Enhancing the visualization
To enhance the visualization further, we can add additional features such as trend lines, annotations, and custom styling to make the chart more informative and visually appealing. JFreeChart provides extensive customization options to achieve these enhancements.
Adding trend lines
Trend lines can provide valuable insights into the overall trend of the data. We can add a trend line to the chart to visualize the general direction of the popularity trend over the specified timeframe.
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
// Inside the GoogleTrendsChart class
private JFreeChart createChart(TimeSeriesCollection dataset) {
JFreeChart chart = ChartFactory.createTimeSeriesChart(
"Google Trends Data Visualization",
"Date",
"Popularity",
dataset,
false,
true,
false
);
TimeSeries series = dataset.getSeries(0);
XYSeries trendLine = createTrendLine(series);
XYSeriesCollection trendLineDataset = new XYSeriesCollection(trendLine);
// Adding the trend line to the chart
chart.getXYPlot().setDataset(1, trendLineDataset);
chart.getXYPlot().setRenderer(1, new org.jfree.chart.renderer.xy.XYLineAndShapeRenderer(true, false));
chart.getXYPlot().getRendererForDataset(trendLineDataset).setSeriesPaint(0, Color.RED);
return chart;
}
private XYSeries createTrendLine(TimeSeries series) {
XYSeries trendLine = new XYSeries("Trend Line");
// Logic to calculate trend line points and add them to the series
// ...
return trendLine;
}
In this code snippet, we enhance the chart by adding a trend line to visualize the overall trend of the popularity data. We calculate the trend line points based on the data and add them to a new XYSeries
. We then create an XYSeriesCollection
with the trend line series and add it to the chart's plot.
Adding annotations
Annotations can provide additional context or highlight specific data points on the chart. We can add annotations to mark significant events or points of interest in the Google Trends data.
import org.jfree.chart.annotations.XYTextAnnotation;
// Inside the GoogleTrendsChart class
private JFreeChart createChart(TimeSeriesCollection dataset) {
// ...
// Adding annotations to the chart
XYTextAnnotation annotation = new XYTextAnnotation("Peak Interest", series.getTimePeriod(peakIndex).getStart().getTime(), series.getValue(peakIndex));
annotation.setFont(new Font("Arial", Font.PLAIN, 12));
annotation.setPaint(Color.BLUE);
chart.getXYPlot().addAnnotation(annotation);
return chart;
}
In this code, we add an annotation to mark a peak interest point on the chart. We specify the text, position, font, and color for the annotation to make it stand out on the visualization.
Custom styling
Customizing the appearance of the chart can significantly impact its visual appeal and readability. We can customize various aspects such as colors, fonts, grid lines, and axis labels to align with the branding or specific requirements of the visualization.
private JFreeChart createChart(TimeSeriesCollection dataset) {
// ...
chart.setBackgroundPaint(Color.WHITE);
chart.getXYPlot().setDomainGridlinePaint(Color.LIGHT_GRAY);
chart.getXYPlot().setRangeGridlinePaint(Color.LIGHT_GRAY);
chart.getXYPlot().setDomainGridlinesVisible(true);
chart.getXYPlot().setRangeGridlinesVisible(true);
chart.getXYPlot().getDomainAxis().setLabelFont(new Font("Arial", Font.BOLD, 14));
chart.getXYPlot().getRangeAxis().setLabelFont(new Font("Arial", Font.BOLD, 14));
chart.getXYPlot().getDomainAxis().setTickLabelFont(new Font("Arial", Font.PLAIN, 12));
chart.getXYPlot().getRangeAxis().setTickLabelFont(new Font("Arial", Font.PLAIN, 12);
return chart;
}
In this snippet, we customize the background color, grid lines, axis labels, and fonts to align with the design and readability requirements of the visualization.
Closing the Chapter
In this blog post, we explored the significance of data visualization, leveraging Google Trends data as a valuable source of insights, and harnessing the power of Java and JFreeChart to create visually appealing and informative visualizations. By fetching and processing Google Trends data and employing JFreeChart for visualization, we demonstrated the potential of Java for optimizing Google Trends data visualization.
Data visualization is a vast and dynamic field, and the techniques discussed in this post serve as a starting point for creating compelling visuals from Google Trends data. As technology continues to evolve, new tools and techniques will undoubtedly emerge, further enhancing our ability to derive actionable insights from data visualization.
By consistently refining our skills and embracing innovation, we can unlock the full potential of data visualization to drive informed decision-making and stimulate meaningful discussions across various domains.
For further reading on data visualization using Java, you might find these resources helpful:
- JFreeChart Official Documentation
- JavaFX Charts
- Apache ECharts
Happy visualizing!