Achieving Smooth UI Updates with ZK's Asynchronous Processing
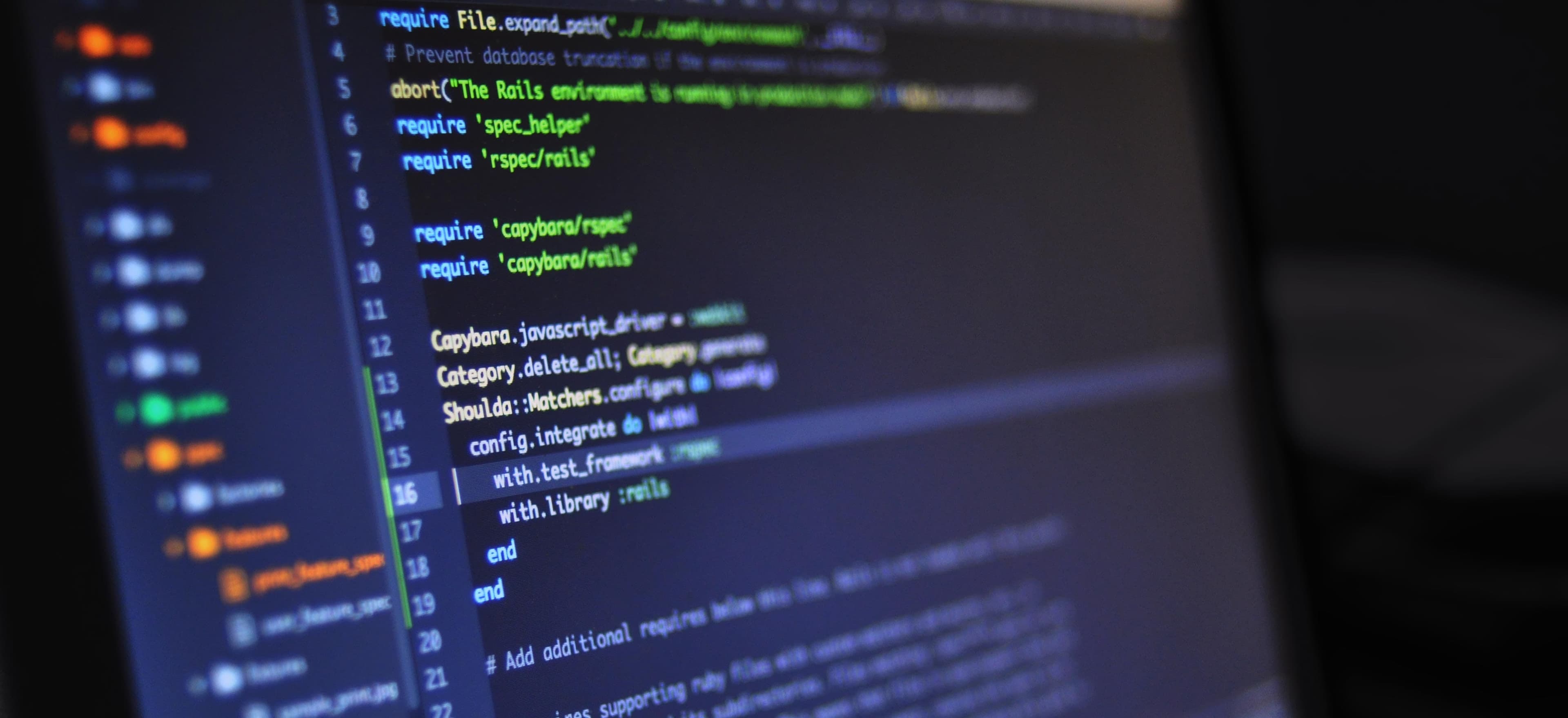
- Published on
Achieving Smooth UI Updates with ZK's Asynchronous Processing
In modern web development, creating a seamless user experience is crucial to the success of any web application. Asynchronous processing plays a key role in achieving smooth and responsive user interfaces by allowing the UI to remain interactive while background tasks are executed. In this article, we'll explore how to leverage ZK's asynchronous processing capabilities to achieve smooth UI updates in Java web applications.
Understanding Asynchronous Processing
Asynchronous processing allows tasks to be executed independently from the main application thread, preventing the user interface from becoming unresponsive during long-running operations. This is particularly important when dealing with tasks such as making network requests, performing complex calculations, or fetching data from a database.
In a traditional synchronous setup, long-running tasks would block the main thread, causing the UI to freeze until the task is completed. Asynchronous processing, on the other hand, allows these tasks to run in the background, freeing up the main thread to continue responding to user input.
Leveraging ZK's Asynchronous Features
ZK is a powerful open-source Java web framework that provides a rich set of components and features for building interactive web applications. One of ZK's key strengths is its built-in support for asynchronous processing, which allows developers to create responsive and fluid user interfaces without sacrificing functionality.
ZK's asynchronous features are particularly useful for scenarios where long-running tasks need to be performed without impacting the user experience. By leveraging ZK's asynchronous capabilities, developers can ensure that the UI remains responsive and continues to provide feedback to the user while background tasks are executed.
Let's dive into some code examples to illustrate how ZK's asynchronous processing can be used to achieve smooth UI updates.
Example 1: Using ZK's @Listen
Annotation for Asynchronous Event Handling
@Listen("onClick = #asyncButton")
public void onAsyncButtonClick() {
// Simulate a time-consuming task
new Thread(() -> {
try {
Thread.sleep(5000); // Simulate a 5-second task
// Update the UI after the task is completed
Executions.schedule(desktop, () -> {
asyncLabel.setValue("Task completed!");
}, new Event("onAsyncTaskComplete"));
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
In this example, we have a button with the ID asyncButton
and a label with the ID asyncLabel
. When the button is clicked, the onAsyncButtonClick
method is invoked asynchronously. Within this method, we simulate a time-consuming task using a separate thread. Once the task is completed, we use Executions.schedule
to update the UI with the result.
The key here is the use of the @Listen
annotation, which allows us to handle the button click event asynchronously without blocking the main thread.
Example 2: Asynchronous Data Loading with ZK's Listbox Component
@Listen("onCreate = #asyncListbox")
public void onAsyncListboxCreate() {
// Simulate fetching data from a remote server
new Thread(() -> {
try {
Thread.sleep(3000); // Simulate a 3-second data fetch operation
ListModelList<String> data = new ListModelList<>(Arrays.asList("Item 1", "Item 2", "Item 3"));
// Update the Listbox with the fetched data
Executions.schedule(desktop, () -> {
asyncListbox.setModel(data);
}, new Event("onAsyncDataLoad"));
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
In this example, we have a Listbox component with the ID asyncListbox
. When the Listbox is created, the onAsyncListboxCreate
method is invoked asynchronously. Within this method, we simulate fetching data from a remote server using a separate thread. Once the data is fetched, we update the Listbox with the fetched data using Executions.schedule
.
This approach ensures that the UI remains responsive while the data is being fetched, providing a smooth and seamless user experience.
Final Considerations
In summary, ZK's asynchronous processing capabilities provide a powerful mechanism for achieving smooth UI updates in Java web applications. By leveraging features such as the @Listen
annotation and Executions.schedule
, developers can ensure that the user interface remains responsive and interactive even during long-running operations.
To learn more about ZK's asynchronous processing and other features, check out the ZK documentation and explore the ZK showcase for interactive examples.
Incorporating asynchronous processing into your Java web applications with ZK will not only lead to a more seamless user experience but also improve the overall performance and responsiveness of your application. Happy coding!