Converting Legacy Code to Testable Code: Overloading for Improved Testability
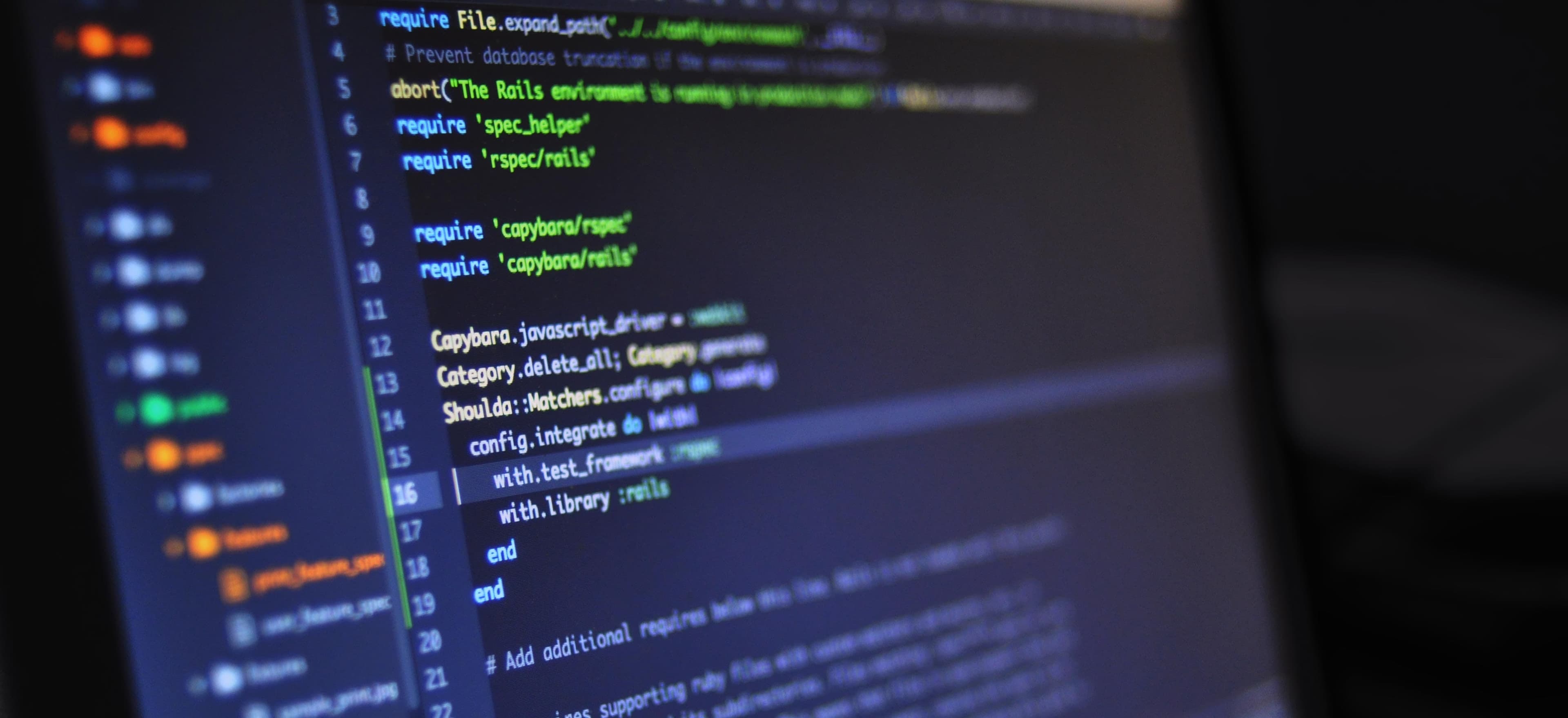
- Published on
Converting Legacy Code to Testable Code: Overloading for Improved Testability
In the world of Java development, legacy code often poses a significant challenge, especially when it comes to writing unit tests. Legacy code is typically hard to test because it wasn’t written with testing in mind. However, there are techniques that can be applied to make legacy code more testable, and one such technique is overloading.
Understanding Overloading
Overloading is a feature in Java that allows a class to have more than one method having the same name, if their parameter lists are different. This is also known as method overloading. When overloading methods, the return type may or may not be different, but the parameter list must differ.
Consider the following example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In this example, the add
method is overloaded to accept both int
and double
parameters.
Overloading for Testability
When dealing with legacy code, overloading can be leveraged to improve testability. Legacy code often has methods that perform multiple tasks making them difficult to test in isolation. By overloading these methods with variations that accept fewer parameters or have simpler behaviors, we can make the code more modular and testable.
Let’s consider a scenario with a legacy Calculator
class that has the following method:
public class LegacyCalculator {
public int calculateTotal(int num1, int num2, int num3) {
return num1 + num2 + num3;
}
}
This method takes three integers and returns their sum. Testing this method in isolation can be challenging due to the multiple parameters and complex logic.
By overloading the calculateTotal
method, we can create simpler variations to test specific behaviors. For example:
public int calculateTotal(int num1, int num2) {
return num1 + num2;
}
By overloading the calculateTotal
method in this manner, we enable writing tests that focus specifically on the logic for adding two numbers, without the need to provide a third number.
Why Overloading Improves Testability
-
Modularity: Overloading allows breaking down complex methods into simpler and smaller units. Each overloaded method can then be tested independently, focusing on a specific behavior or subset of the original method's functionality.
-
Simplicity: Overloaded methods can encapsulate specific behaviors, making the testing process simpler and more focused.
-
Readability: Overloading can improve code readability by providing more descriptive method names for specific use cases, leading to better understanding and easier maintenance.
Guidelines for Overloading Legacy Code for Testability
When overloading methods for improved testability, it’s essential to follow certain guidelines to ensure the effectiveness of the process.
1. Identify Complex Methods
Look for methods in the legacy codebase that are complex and perform multiple tasks. These are the methods that could benefit from overloading for improved testability.
2. Determine Testable Units
Identify the specific behaviors or tasks within the complex methods that can be separated into individual testable units. These will form the basis for the overloaded methods.
3. Decouple Dependencies
When overloading methods, ensure that the overloaded methods are not directly dependent on the internal state of the original method. They should be self-contained and not rely on shared mutable state.
4. Maintain Consistency
Ensure that the overloaded methods maintain consistency in naming and behavior. Each overloaded method should represent a coherent and specific behavior.
5. Document Clearly
Document the newly overloaded methods clearly, highlighting their specific purpose and usage. This documentation will be crucial for understanding and maintaining the codebase.
Example: Overloading Legacy Code in Practice
Let’s consider a practical example of overloading methods for improved testability in legacy code.
Legacy Code
Suppose we have a legacy UserService
class with the following method:
public class UserService {
public boolean validateUser(String username, String password, String email) {
// Complex validation logic
// ...
}
}
Overloaded Methods
We can improve the testability of the validateUser
method by overloading it as follows:
public boolean validateUser(String username, String password) {
// Simple validation logic for username and password
// ...
}
public boolean validateUser(String email) {
// Simple validation logic for email
// ...
}
By overloading the validateUser
method, we have created two simpler and more focused methods that can be tested independently without the need to validate all three parameters at once.
To Wrap Things Up
Overloading methods in legacy code for improved testability is a valuable technique in the arsenal of a Java developer. By breaking down complex methods into simpler and more focused units, overloading allows for more targeted and effective unit testing, ultimately leading to a more maintainable and robust codebase.
When dealing with legacy code, it’s important to assess the suitability of overloading as a means to enhance testability, keeping in mind the principles of modularity, simplicity, and consistency.
Incorporating overloading for improved testability not only facilitates the testing process but also contributes to the overall quality and agility of the codebase, enabling easier maintenance and future enhancements.
To delve deeper into the world of Java development, explore the official Java documentation and consider learning about advanced topics such as design patterns and clean code principles.