Optimizing Dependency Injection with Spring Core Annotations
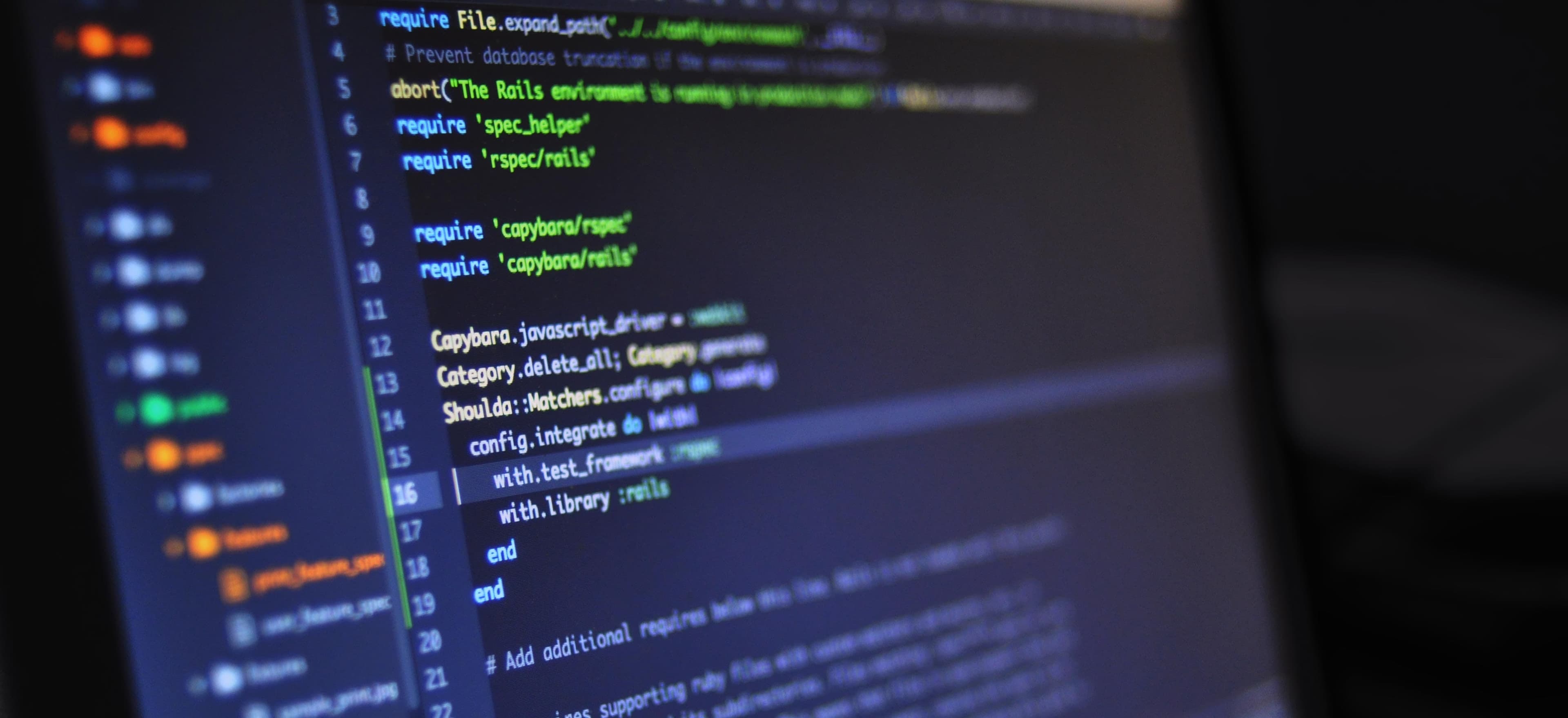
- Published on
Optimizing Dependency Injection with Spring Core Annotations
In the world of Java development, the Spring Framework has established itself as a powerful and versatile tool for building enterprise-grade applications. One of the key features that makes Spring such a popular choice among developers is its support for dependency injection. Dependency injection is a design pattern that allows for the creation of loosely coupled components within an application, making it more maintainable, testable, and scalable.
In this blog post, we will explore how to optimize dependency injection using Spring Core annotations. We will delve into the various annotations provided by the Spring Framework that can streamline the process of defining dependencies and wiring them together in a Java application.
Understanding Dependency Injection
Before we jump into the Spring annotations, let's take a quick refresher on what dependency injection is all about. In a nutshell, dependency injection is a technique where the dependencies of a class are provided from the outside. This approach allows for better separation of concerns and easier testing, as dependencies can be easily mocked or replaced.
In the context of Spring, dependency injection can be achieved through constructor injection, setter injection, or field injection. Each of these methods has its own use cases, and Spring provides annotations to facilitate each approach.
@Autowired for Constructor Injection
One of the most common ways to perform dependency injection in Spring is through constructor injection. The @Autowired
annotation can be used to mark a constructor to be autowired by Spring, meaning that Spring will automatically resolve and inject the dependencies.
Here's an example of using @Autowired
for constructor injection:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// ... other methods
}
In this example, the UserService
class has a constructor that takes a UserRepository
as a parameter. By annotating the constructor with @Autowired
, we are telling Spring to inject an instance of UserRepository
when creating an instance of UserService
.
Using constructor injection with @Autowired
can lead to better immutability and thread safety, as the dependencies are injected through the constructor and cannot be changed after the object is created.
@Autowired for Setter Injection
Another approach to dependency injection in Spring is through setter injection. Setter injection involves creating setter methods for the dependencies and annotating them with @Autowired
.
Let's see an example of setter injection using @Autowired
:
@Component
public class NotificationService {
private EmailService emailService;
@Autowired
public void setEmailService(EmailService emailService) {
this.emailService = emailService;
}
// ... other methods
}
In this example, the NotificationService
class has a setter method setEmailService
for injecting the EmailService
dependency. By annotating the setter method with @Autowired
, Spring will automatically inject the EmailService
when creating an instance of NotificationService
.
Setter injection is often used when a class has optional dependencies or when circular dependencies need to be resolved.
@Autowired for Field Injection
Field injection is the simplest form of dependency injection, where the dependencies are directly injected into the fields of a class. While this approach is straightforward, it can make testing more challenging and can lead to tight coupling between classes.
Here's an example of field injection using @Autowired
:
@Service
public class OrderService {
@Autowired
private PaymentService paymentService;
// ... other methods
}
In this example, the PaymentService
dependency is directly annotated with @Autowired
, allowing Spring to inject the PaymentService
when creating an instance of OrderService
.
While field injection may seem convenient, it's generally recommended to use constructor or setter injection over field injection to improve testability and maintainability.
@Qualifier for Dependency Resolution
When working with multiple implementations of an interface, you may encounter the need to disambiguate which bean should be injected. This is where the @Qualifier
annotation comes into play.
Consider the following example where we have multiple implementations of a PaymentGateway
interface:
@Component
@Qualifier("creditCard")
public class CreditCardPaymentGateway implements PaymentGateway {
// ... implementation
}
@Component
@Qualifier("paypal")
public class PayPalPaymentGateway implements PaymentGateway {
// ... implementation
}
@Service
public class PaymentService {
private final PaymentGateway paymentGateway;
@Autowired
public PaymentService(@Qualifier("paypal") PaymentGateway paymentGateway) {
this.paymentGateway = paymentGateway;
}
// ... other methods
}
In this example, the @Qualifier
annotation is used to specify which bean should be injected into the PaymentService
. By providing the qualifier value, we disambiguate between the multiple implementations of PaymentGateway
.
@Primary for Default Bean
In cases where there are multiple beans of the same type and there is a need to define a primary bean, the @Primary
annotation can be used.
Let's consider an example where we have multiple implementations of a MessageSender
interface:
@Component
@Primary
public class EmailMessageSender implements MessageSender {
// ... implementation
}
@Component
public class SmsMessageSender implements MessageSender {
// ... implementation
}
@Service
public class NotificationService {
private final MessageSender messageSender;
@Autowired
public NotificationService(MessageSender messageSender) {
this.messageSender = messageSender;
}
// ... other methods
}
In this example, the @Primary
annotation is used to designate EmailMessageSender
as the default bean to be injected when there are multiple candidates for autowiring.
Bringing It All Together
In this blog post, we have explored how to optimize dependency injection using Spring Core annotations. We have learned about various annotations such as @Autowired
for constructor, setter, and field injection, @Qualifier
for disambiguating beans, and @Primary
for defining a default bean.
By leveraging these annotations, we can streamline the process of defining and injecting dependencies in a Spring application, leading to cleaner, more maintainable code. It's important to keep in mind that while these annotations can greatly simplify the process of dependency injection, they should be used judiciously to maintain the clarity and structure of the application.
To dive deeper into Spring Core annotations and best practices for dependency injection, take a look at the official Spring Framework documentation.
Happy coding!