Best Practices for Null Checking in C#
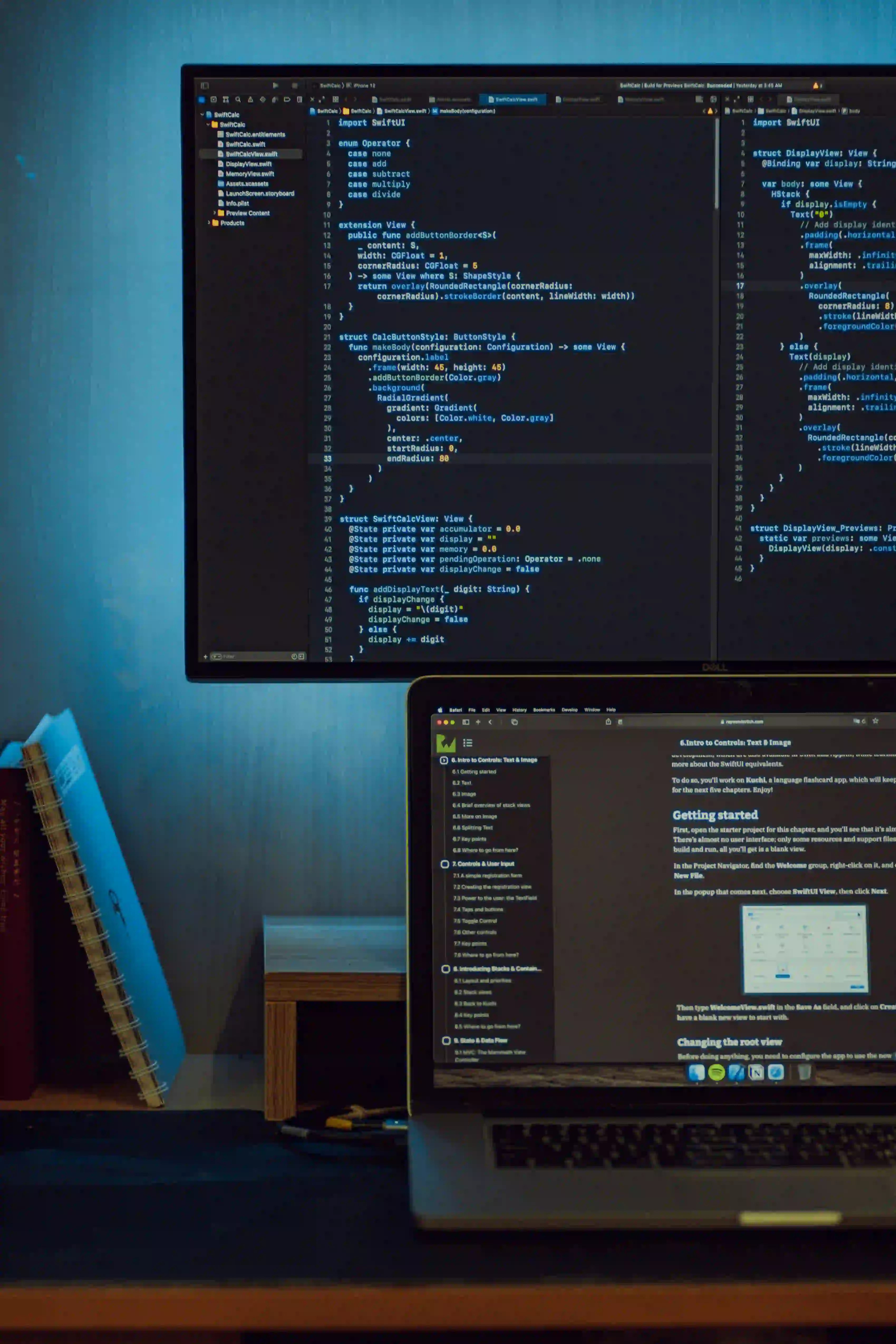
Best Practices for Null Checking in C#
Null reference errors are a common source of bugs and crashes in software development. In C#, null checking is a crucial aspect of writing robust and reliable code. Failing to check for null references can lead to NullReferenceException
which can cause your application to terminate unexpectedly. In this article, we will explore best practices for null checking in C# to help you write more resilient and maintainable code.
1. Use the Null-Conditional Operator
Introduced in C# 6.0, the null-conditional operator (?.
) allows you to safely access members and elements of an object without risking a null reference exception. It short-circuits the statement and returns null if any of the accessed members are null.
string name = person?.Address?.City;
By using the null-conditional operator, you can succinctly handle potential null references without writing verbose if statements.
2. Null Coalescing Operator for Default Values
When dealing with null values, the null coalescing operator (??
) can be used to provide a default value if the operand on the left-hand side is null.
int result = nullableIntValue ?? 0;
This ensures that even if the nullableIntValue
is null, the result
will have a default value of 0, thus preventing null reference exceptions.
3. Use the is
Keyword for Type Checking
When performing type checking against potential null references, use the is
keyword to ensure the object is not null before checking its type, as shown in the following example:
if (obj is MyClass myClassObj)
{
// Safely use myClassObj
}
By using the is
keyword, you combine null checking with the type check, ensuring that the object is not null before further processing.
4. Leverage Pattern Matching with switch
Pattern matching with the switch
statement can also be used for null checking and type casting simultaneously, leading to more concise and readable code.
switch (obj)
{
case MyClass myClassObj:
// Safely use myClassObj
break;
case null:
// Handle null case
break;
default:
// Handle other cases
break;
}
5. Avoid Nested Null Checks
Nested null checks can clutter your code and decrease its readability. Consider using the null-conditional operator or early returns to simplify nested null checking logic and improve code maintainability.
if (obj1 != null)
{
if (obj1.Property != null)
{
// Access obj1.Property
}
}
Refactor the nested null checks to utilize the null-conditional operator for a cleaner and more readable code.
6. Use Code Analysis Tools
Utilize code analysis tools such as Roslyn Analyzers or ReSharper to detect potential null reference issues in your codebase. These tools can provide insights and suggestions for improving null checking and handling in your C# code.
7. Document Expected Null Behavior
In cases where a method or property is expected to return null under certain conditions, document this behavior explicitly using XML comments. This helps other developers understand the expected null behavior and handle it accordingly.
/// <summary>
/// Retrieves the user by ID.
/// Returns null if the user is not found.
/// </summary>
public User GetUserById(int userId)
{
// Implementation
}
Explicitly documenting the expected null behavior enhances code readability and understanding for maintainers.
A Final Look
Null reference errors can introduce significant vulnerabilities to your code. By following these best practices for null checking in C#, you can enhance the robustness and reliability of your codebase. Leveraging language features such as the null-conditional and null coalescing operators, along with pattern matching and code analysis tools, can help you effectively prevent null reference exceptions and write more maintainable C# code.
Remember, null checking is not only about preventing exceptions, but also about writing clear and understandable code that makes the intent of handling null values explicit.
When in doubt, always prioritize defensive coding practices, and remember: "When in doubt, check for null!"
Utilize these best practices in your C# projects to minimize null reference issues and contribute to building a more stable and secure codebase.
This article on null checking in C# was brought to you by [YourCompany], where we are passionate about writing robust and reliable C# code.