Managing Stale Data in Spring Data Repositories
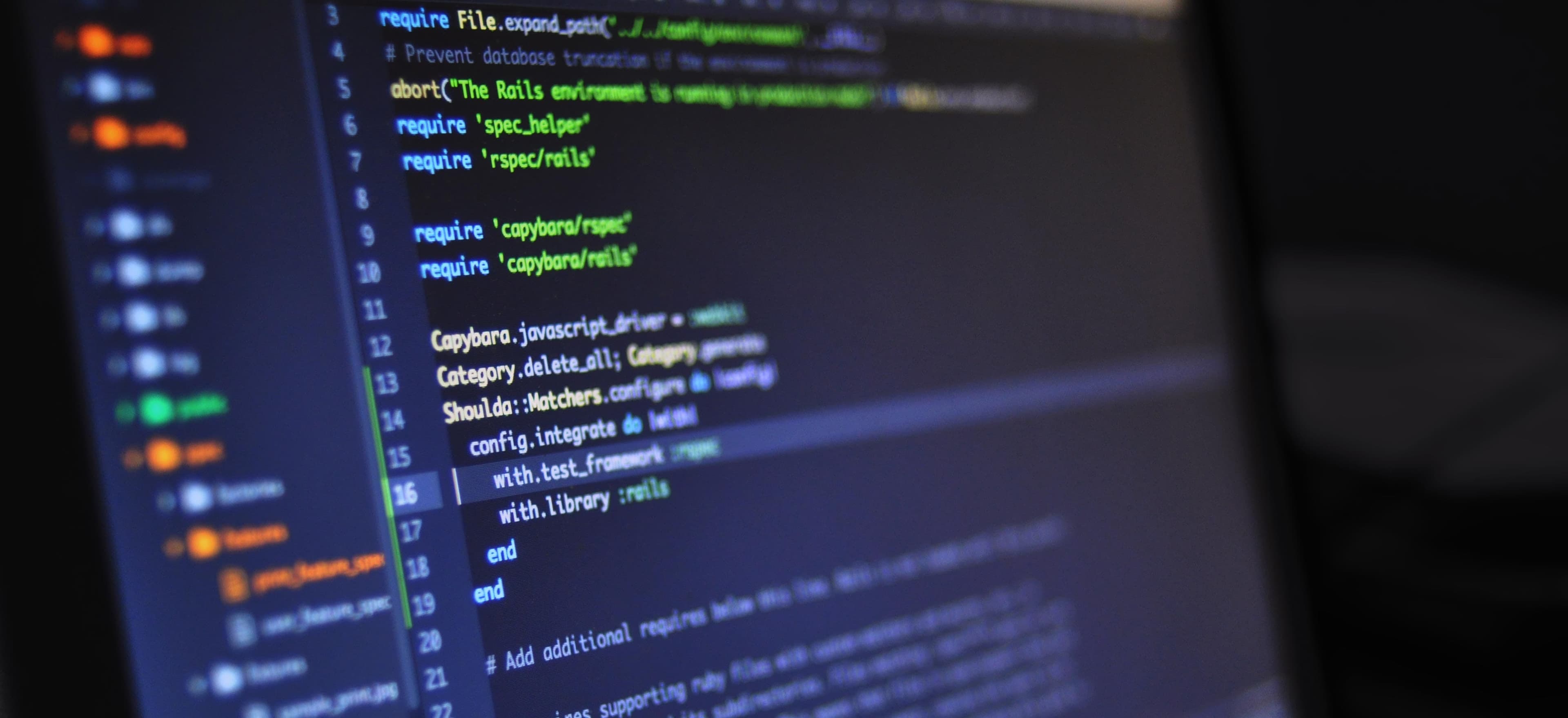
- Published on
Dealing with Stale Data in Spring Data Repositories
In a multi-user environment, it's common for data to become outdated due to concurrent transactions or caching. In such scenarios, managing stale data is crucial to ensure data consistency and integrity. This article explores how to handle stale data in Spring Data repositories using optimistic locking strategies.
Understanding Stale Data
Stale data refers to information that has become outdated and no longer reflects the current state of the system. This can occur when a user retrieves a piece of data, makes changes to it, and then tries to save the changes, only to realize that the data has been modified by another user or process in the meantime.
Optimistic Locking
Optimistic locking is a strategy used to handle concurrent updates to an entity. Instead of locking the resource during a transaction, optimistic locking assumes there won’t be contention for the resource and only checks for concurrent modifications at the time of update. If the data has been altered by another transaction, a conflict resolution mechanism can be applied to handle the situation.
Using @Version for Optimistic Locking
In Spring Data, optimistic locking is often implemented using the @Version
annotation. When an entity has a field annotated with @Version
, it indicates that this field will be used for optimistic locking. The field is typically of type long
or int
and is automatically incremented by the persistence provider upon each update.
Let's look at an example of using @Version
for optimistic locking in a Spring Data JPA entity:
import javax.persistence.Entity;
import javax.persistence.Version;
@Entity
public class Product {
// Other fields
@Version
private Long version;
// Getters and setters
}
In this example, the version
field is annotated with @Version
, indicating that it should be used for optimistic locking. Now, every time an update is made to a Product
entity, the version
field will be automatically incremented by the JPA provider. If another transaction attempts to update the same entity concurrently, a javax.persistence.OptimisticLockException
will be thrown, allowing you to handle the conflict gracefully.
Handling OptimisticLockException
When an OptimisticLockException
is thrown, you can catch it and perform conflict resolution. This might involve merging the changes, asking the user to review the conflicting updates, or any other custom resolution strategy that suits your application’s requirements.
try {
// Update the entity
} catch (OptimisticLockException ex) {
// Handle the conflict
}
Configuring Spring Data JPA for Optimistic Locking
To enable optimistic locking in Spring Data JPA repositories, you can do so by annotating your repository interface with @Lock
:
import org.springframework.data.jpa.repository.Lock;
import org.springframework.data.repository.CrudRepository;
@Lock(LockModeType.OPTIMISTIC)
public interface ProductRepository extends CrudRepository<Product, Long> {
// Repository methods
}
In this example, the ProductRepository
is annotated with @Lock(LockModeType.OPTIMISTIC)
, indicating that optimistic locking should be applied to all operations performed by this repository.
Using Optimistic Locking with Spring Data MongoDB
For Spring Data MongoDB, optimistic locking is supported through the @Version
annotation as well. When using MongoDB, the _class
field is used to handle optimistic locking, and it should be of type Long
or Integer
.
import org.springframework.data.annotation.Version;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class Product {
// Other fields
@Version
private Long version;
// Getters and setters
}
By annotating the version
field with @Version
, the MongoDB persistence provider will handle the optimistic locking automatically.
Closing the Chapter
Handling stale data is essential for maintaining data consistency in multi-user applications. Optimistic locking, when implemented correctly, provides a mechanism to detect and resolve concurrent modifications to the data. Spring Data repositories, whether for JPA or MongoDB, provide convenient ways to implement optimistic locking using the @Version
annotation and repository configuration.
By leveraging optimistic locking in your Spring Data repositories, you can ensure data integrity and provide a smooth user experience, even in highly concurrent environments.
To explore more about Spring Data and other Java topics, check out the Spring Data Official Documentation.