Implementing Dependency Injection in Java Spring
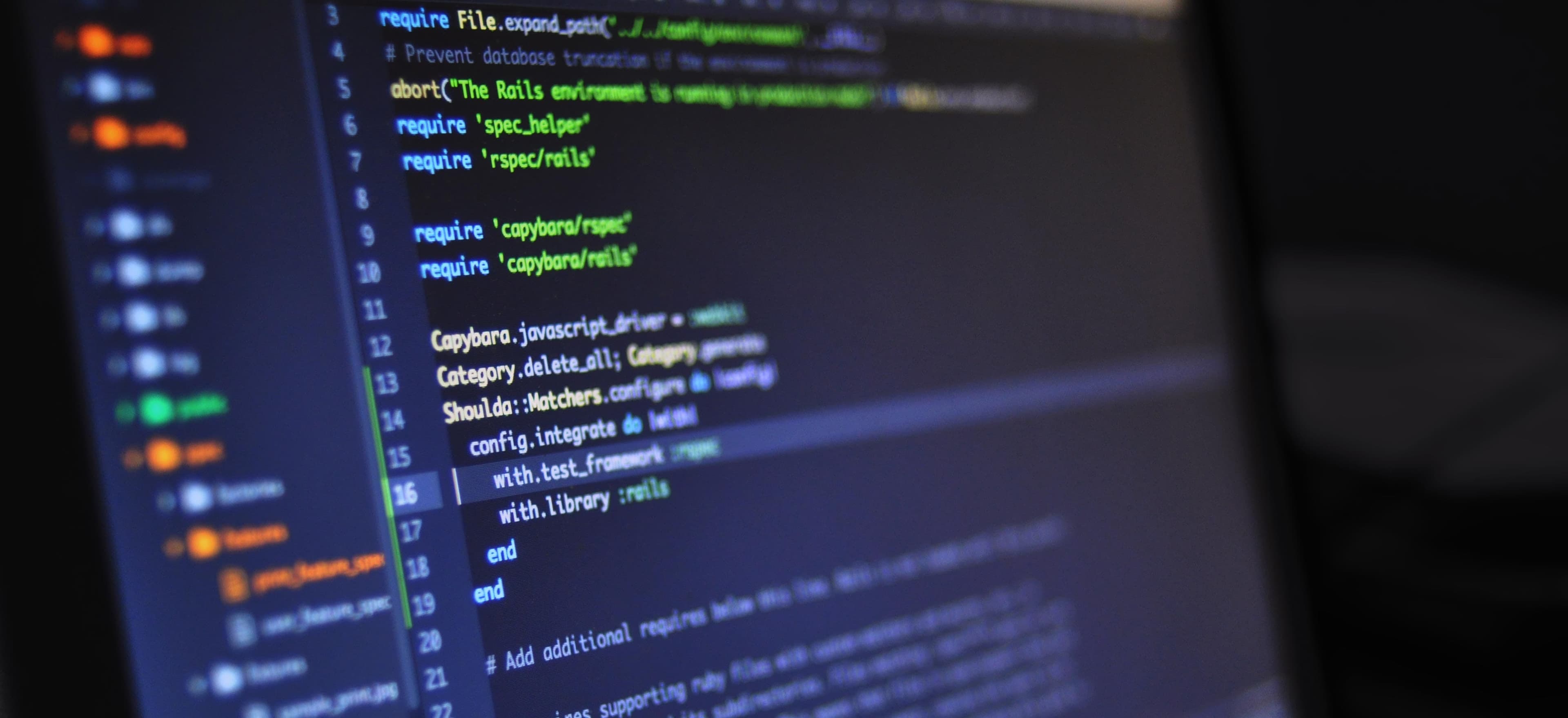
- Published on
Understanding Dependency Injection in Java Spring
In the world of Java development, Dependency Injection (DI) is a critical concept that plays a pivotal role in writing clean, maintainable, and testable code. In this article, we will delve into the significance of Dependency Injection and how it can be implemented in Java Spring.
What is Dependency Injection?
At its core, Dependency Injection is a design pattern that allows the removal of hard-coded dependencies in the code. Instead of creating instances of required dependencies within a class, these dependencies are provided from outside the class. This decoupling of dependencies enables flexibility, testability, and reusability of code.
Implementing Dependency Injection in Java Spring
Java Spring facilitates the implementation of Dependency Injection through its Inversion of Control (IoC) container. The IoC container manages the instantiation and lifecycle of application objects, also known as beans, and their dependencies. There are several ways to perform Dependency Injection in Java Spring, with the two primary methods being constructor-based injection and setter-based injection.
Constructor-Based Injection
Constructor-based injection involves injecting dependencies through the class constructor. Let’s consider the following example:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// UserService methods that utilize userRepository
}
In this example, the UserService
class has a constructor that takes a UserRepository
as a parameter. When an instance of UserService
is created, the UserRepository
dependency is injected into the class through the constructor.
Setter-Based Injection
Setter-based injection, on the other hand, involves providing setter methods for the dependencies to be injected. Here’s an example:
public class UserService {
private UserRepository userRepository;
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
// UserService methods that utilize userRepository
}
In this scenario, the UserService
class provides a setter method setUserRepository
to set the UserRepository
dependency.
Benefits of Dependency Injection in Java Spring
The use of Dependency Injection in Java Spring offers numerous benefits, including:
- Loose Coupling: Classes are not tightly coupled to their dependencies, allowing for easier modification and testing.
- Testability: Dependency Injection makes it simpler to write unit tests by providing the ability to easily mock or stub dependencies.
- Reusability: With Dependency Injection, components become more reusable as they are independent of their dependencies.
Autowiring in Java Spring
Autowiring is a convenient feature of Java Spring that automatically wires the dependencies in Spring beans. By using the @Autowired
annotation, Spring will automatically resolve and inject the matching beans into the properties of a class.
Here’s an example of how Autowiring can be used in Java Spring:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
// UserService methods that utilize userRepository
}
In this example, the @Autowired
annotation eliminates the need for explicit setter methods or constructors to inject the UserRepository
dependency into the UserService
class.
Qualifiers in Autowiring
In scenarios where there are multiple beans of the same type, the use of qualifiers becomes necessary to specify which bean should be injected. Let’s consider an example:
@Service
public class UserService {
@Autowired
@Qualifier("h2UserRepository")
private UserRepository userRepository;
// UserService methods that utilize userRepository
}
In this case, the @Qualifier
annotation is used to specify the desired bean by its name.
In Conclusion, Here is What Matters
In conclusion, Dependency Injection is a foundational concept for writing maintainable and testable code, and Java Spring provides a powerful framework for implementing it. By leveraging the IoC container and the capabilities of Autowiring, Spring simplifies the process of managing dependencies and promotes the development of clean, decoupled code.
To summarize, Dependency Injection in Java Spring:
- Promotes loose coupling
- Enhances testability
- Facilitates reusability
- Streamlines dependency management through the IoC container and Autowiring
By mastering Dependency Injection in Java Spring, developers can elevate the quality and maintainability of their applications while embracing best practices in software design.
For a deeper dive into the topic of Dependency Injection in Java Spring, check out Spring Framework Documentation for comprehensive insights.
Start harnessing the power of Dependency Injection in Java Spring to revolutionize your code architecture and propel your development journey to new heights.