Creating Type-Safe Maps in Java 8
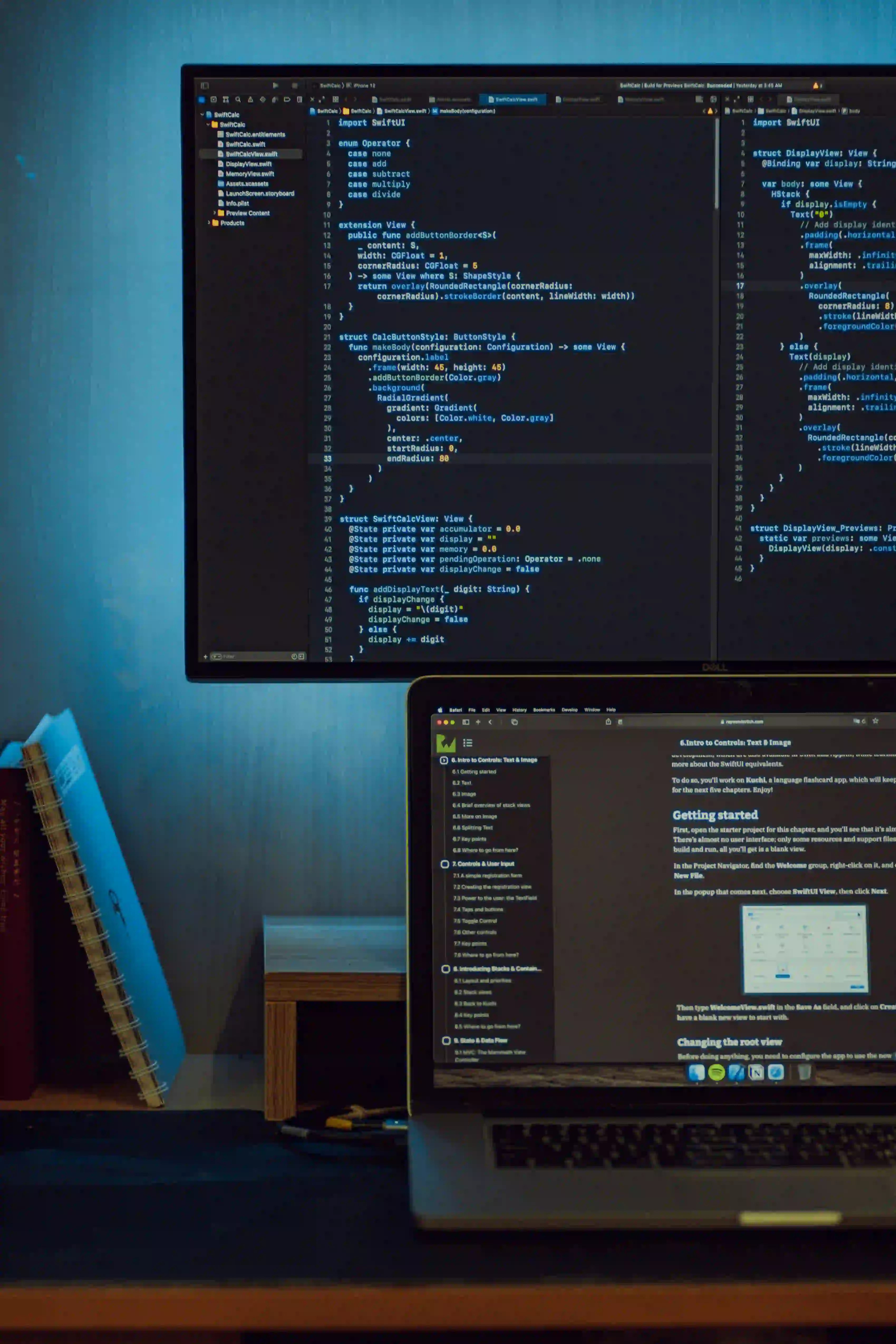
Creating Type-Safe Maps in Java 8
When working with maps in Java, it's common to come across scenarios where the type safety of the map becomes crucial. In Java 8, the introduction of lambda expressions and the stream API has made it easier to work with collections, including maps. However, ensuring type safety while working with maps still requires some effort.
In this article, we'll explore how to create type-safe maps in Java 8 to prevent runtime errors and provide a more robust and maintainable codebase.
The Challenge of Type Safety in Java Maps
One of the challenges of working with traditional maps in Java is the lack of type safety, especially when dealing with complex data structures. Consider the following code snippet:
Map myMap = new HashMap();
myMap.put("key", "value");
Integer retrievedValue = (Integer) myMap.get("key"); // Potential ClassCastException at runtime
In the above example, the code could result in a ClassCastException
at runtime because there's no compile-time guarantee that the value corresponding to the key "key" is an Integer
. This lack of type safety can lead to bugs that are difficult to track and resolve.
Introducing Type-Safe Maps with Generics
Java 5 introduced generics, which provides a way to create type-safe collections. By utilizing generics, we can ensure compile-time type safety for our maps.
Let's create a type-safe map using generics:
Map<String, Integer> myMap = new HashMap<>();
myMap.put("key", 123);
Integer retrievedValue = myMap.get("key"); // No casting required
In this improved version, the compiler ensures that only Integer
values can be retrieved for the key "key". This helps in catching type mismatches at compile time, thus preventing potential runtime errors.
Leveraging Immutable Maps and Type Inference
In Java 8, the Map.of()
and Map.ofEntries()
methods provide a convenient way to create immutable maps in a concise manner, leveraging the benefits of type inference.
Here's an example of creating an immutable type-safe map using Map.of()
:
Map<String, Integer> immutableMap = Map.of("key1", 123, "key2", 456);
The Map.of()
method infers the types, allowing us to create a compact and type-safe map without explicitly specifying the types in the declaration.
Creating Custom Type-Safe Map Implementations
In some cases, we may need to create custom type-safe map implementations to encapsulate specific business logic or to enforce constraints on the key-value pairs. Java provides the flexibility to achieve this by extending the AbstractMap
class or implementing the Map
interface.
Let's create a custom type-safe map that only allows a specific type of value:
public class StringToIntMap extends HashMap<String, Integer> {
@Override
public Integer put(String key, Integer value) {
// Additional validation logic if needed
return super.put(key, value);
}
}
In this example, StringToIntMap
extends HashMap
with the key as String
and the value as Integer
, providing an opportunity to add custom logic, such as validation checks, specific to our use case.
Leveraging Functional Programming for Type-Safe Maps
With the introduction of lambda expressions and the stream API in Java 8, functional programming has become more prevalent in Java development. We can leverage functional programming techniques to work with type-safe maps effectively.
Consider the following example where we use functional programming to process a type-safe map:
Map<String, Integer> myMap = Map.of("key1", 123, "key2", 456);
// Using forEach to iterate over the entries
myMap.forEach((key, value) -> System.out.println(key + " : " + value));
// Utilizing the stream API to perform operations
int sum = myMap.values().stream().mapToInt(Integer::intValue).sum();
In this example, we demonstrate the use of forEach
and the stream API to process the type-safe map. Leveraging functional programming not only simplifies the code but also ensures type safety throughout the operations.
Key Takeaways
Type safety is a crucial aspect of writing robust and maintainable Java code. By leveraging generics, immutable maps, custom map implementations, and functional programming techniques, we can create and work with type-safe maps effectively in Java 8.
Ensuring type safety not only prevents runtime errors but also makes the code more readable and easier to maintain. As Java continues to evolve, developers can expect further improvements and features that enhance type safety and functional programming capabilities.
In conclusion, the ability to create type-safe maps in Java 8 empowers developers to write safer and more reliable code, ultimately leading to more efficient and manageable applications.
By implementing type-safe maps in Java 8, developers can minimize runtime errors and maintain a more robust codebase. This method is essential for creating efficient applications without compromising safety.