Streamlining Data Access Between Salesforce and Android
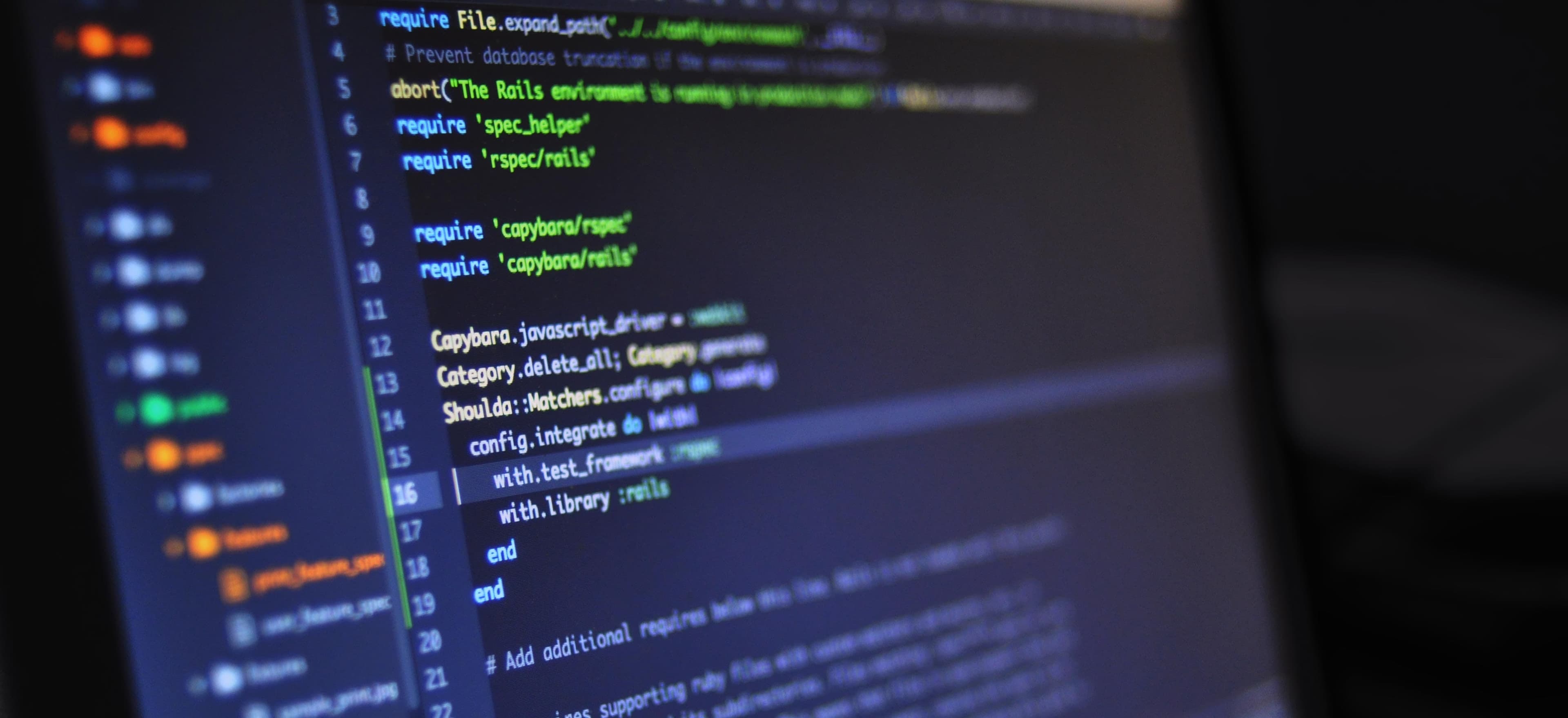
- Published on
Simplifying Data Access: Using Java for Salesforce and Android Integration
In today's fast-paced business environment, seamless data access and integration between different platforms are crucial. Salesforce is a powerful CRM platform widely used for managing customer data, while Android remains the dominant mobile operating system. Integrating these two platforms enables businesses to access and update customer data on the go, leading to improved productivity and customer satisfaction.
Java, being a versatile and widely adopted programming language, plays a pivotal role in bridging the gap between Salesforce and Android. In this article, we will explore how Java can streamline data access between Salesforce and Android, enabling businesses to leverage the full potential of these platforms.
Integration Approaches
Before delving into Java-specific implementation, it's essential to understand the possible approaches for integrating Salesforce with an Android app.
Salesforce Mobile SDK
Salesforce provides a Mobile SDK that allows developers to build custom mobile apps that interact with Salesforce data. This SDK handles the complexities of authentication, data access, and offline capabilities. However, while the SDK simplifies development to some extent, it may not always align with the specific requirements of an Android app.
Custom Integration using REST API
Another approach involves directly integrating with Salesforce using its REST API. This approach provides more flexibility as it allows developers to tailor the integration according to the precise needs of the Android app. However, it requires handling authentication, request building, and response parsing manually.
Leveraging Java for Integration
Java, with its robust libraries and frameworks, serves as an effective tool for integrating Salesforce with Android. Let's explore how Java can be utilized for seamless data access between these platforms.
Using Salesforce REST API with Java
Java's HttpClient library can be employed to interact with the Salesforce REST API. This allows for making HTTP requests to Salesforce endpoints for querying, creating, updating, or deleting records.
// Example of making a GET request to retrieve Salesforce data
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet request = new HttpGet("https://your-instance.salesforce.com/services/data/vXX.X/query?q=SELECT+Id,Name+FROM+Account");
request.addHeader("Authorization", "Bearer your_access_token");
try (CloseableHttpResponse response = httpClient.execute(request)) {
// Handle response
HttpEntity entity = response.getEntity();
if (entity != null) {
String result = EntityUtils.toString(entity);
// Process the JSON response
}
} catch (IOException e) {
// Handle exception
} finally {
try {
httpClient.close();
} catch (IOException e) {
// Handle exception
}
}
In the above code snippet, we demonstrate how Java's HttpClient can be used to fetch data from Salesforce. By leveraging Java's capabilities, we can seamlessly retrieve and process Salesforce data within an Android app.
Managing Authentication
Authentication is a critical aspect of integrating with Salesforce. Java provides robust support for handling authentication, including OAuth 2.0, which is the standard authentication mechanism for Salesforce APIs.
// Example of authenticating with Salesforce using OAuth 2.0
HttpPost request = new HttpPost("https://login.salesforce.com/services/oauth2/token");
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("grant_type", "password"));
params.add(new BasicNameValuePair("client_id", "your_client_id"));
params.add(new BasicNameValuePair("client_secret", "your_client_secret"));
params.add(new BasicNameValuePair("username", "your_username"));
params.add(new BasicNameValuePair("password", "your_password"));
request.setEntity(new UrlEncodedFormEntity(params));
try (CloseableHttpResponse response = httpClient.execute(request)) {
// Handle authentication response
HttpEntity entity = response.getEntity();
if (entity != null) {
String result = EntityUtils.toString(entity);
// Extract access token for subsequent requests
}
} catch (IOException e) {
// Handle exception
}
With Java, developers can seamlessly manage Salesforce authentication, allowing Android apps to securely access Salesforce data.
Data Mapping and Serialization
Java offers robust libraries like Gson for mapping JSON responses from Salesforce to Java objects. This simplifies the process of handling and processing data within an Android app.
// Example of mapping Salesforce JSON response to Java object using Gson
Gson gson = new Gson();
YourCustomObject[] objects = gson.fromJson(jsonResponse, YourCustomObject[].class);
By leveraging Java's capabilities for data mapping and serialization, developers can efficiently work with Salesforce data within the Android app, ensuring seamless integration and optimal performance.
Best Practices and Considerations
When using Java to integrate Salesforce with an Android app, certain best practices and considerations should be kept in mind:
Error Handling
Proper error handling is crucial to ensure robustness in the integration. Java's exception handling mechanisms should be used to gracefully manage errors that may arise during data access and communication with Salesforce.
Asynchronous Operations
Given the potentially long-running nature of network operations when interacting with Salesforce, leveraging Java's multithreading and asynchronous programming features can enhance the responsiveness of the Android app.
Security
Security is paramount when integrating with Salesforce, especially when dealing with sensitive customer data. Java provides strong security features that should be carefully implemented to safeguard data during transit and at rest.
Performance Optimization
Efficient data retrieval and processing are essential for a seamless user experience. Leveraging Java's performance optimization techniques can ensure that the integration remains snappy and responsive.
Key Takeaways
Java serves as a powerful ally in streamlining data access between Salesforce and Android. Its rich set of libraries, robust support for network operations, and seamless integration capabilities make it an ideal choice for bridging the gap between these two platforms.
Integrating Salesforce with an Android app using Java empowers businesses to harness the full potential of their customer data, enabling enhanced productivity and a superior user experience.
By understanding the best practices and considerations outlined in this article, developers can leverage Java effectively to create robust, efficient, and secure integrations, ultimately driving business success through streamlined data access.
In conclusion, Java remains a top contender for powering seamless Salesforce and Android integration, unlocking a world of possibilities for businesses and developers alike.
Remember to stay updated with the latest developments in Java, Salesforce, and Android to continuously optimize and refine the integration for maximum efficiency and effectiveness.