Efficient Strategies: Maximizing Productivity and Minimizing Workload
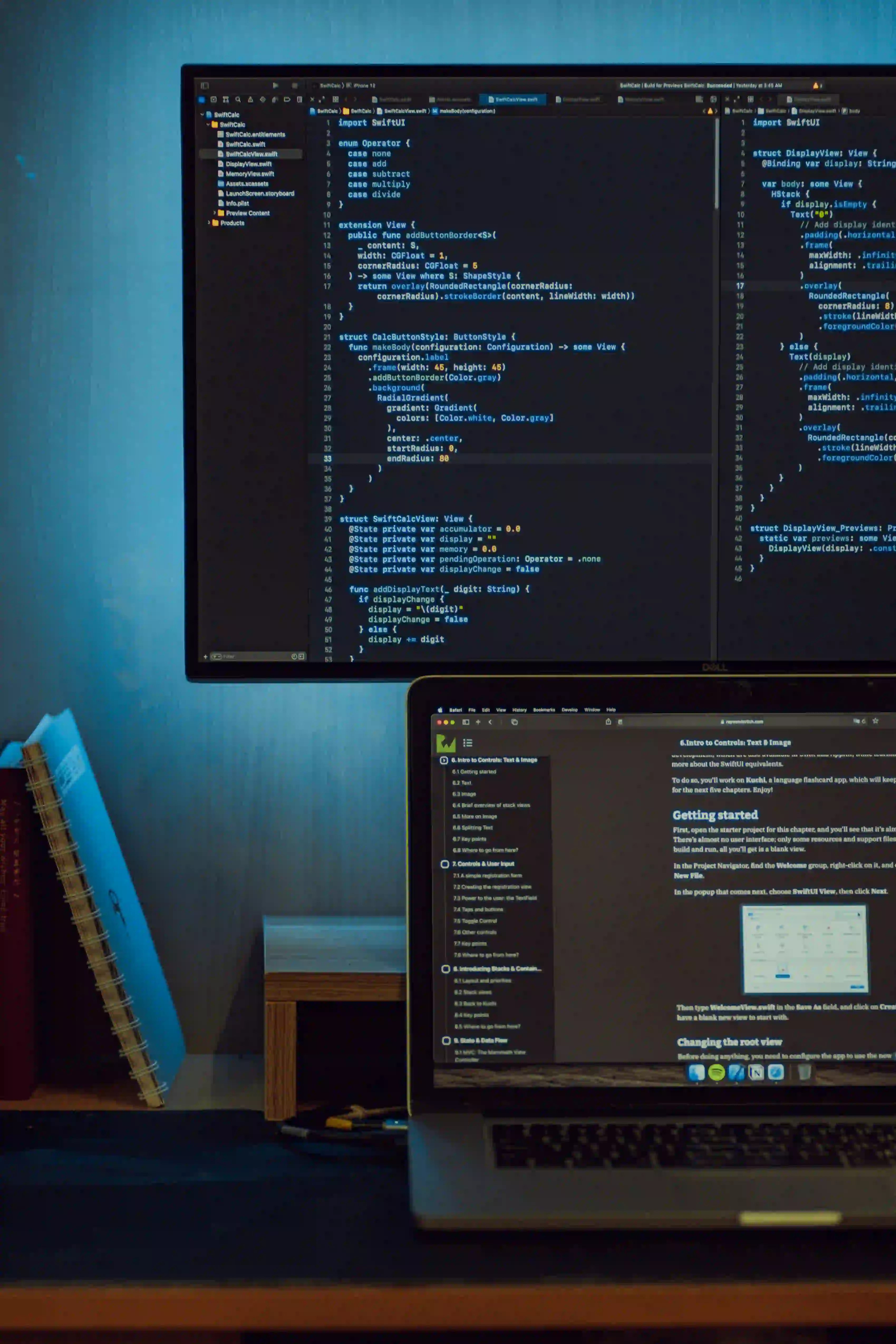
Maximizing Productivity in Java: Efficient Strategies
As a Java developer, maximizing productivity and minimizing workload are crucial for achieving optimal results. This can be achieved through the adoption of efficient strategies that streamline development processes while ensuring high-quality code. In this blog post, we will explore some actionable techniques and best practices in Java development to boost productivity and reduce unnecessary workload.
Utilize Modern Java Features
Java continues to evolve with each new release, introducing modern features that enhance developer productivity. Leveraging these features not only simplifies code but also improves readability and maintainability. For instance, the introduction of the var
keyword in Java 10 reduces boilerplate code when declaring variables. Consider the following example:
// Traditional approach
Map<String, List<String>> traditionalMap = new HashMap<>();
// Modern approach with var
var modernMap = new HashMap<String, List<String>>();
Using var
not only reduces the verbosity of the code but also allows for easier maintenance and refactoring. Embracing modern Java features empowers developers to write cleaner and more concise code, ultimately saving time and effort.
Leverage Java Libraries and Frameworks
Java offers a rich ecosystem of libraries and frameworks that address various development requirements. Instead of reinventing the wheel, utilizing these resources can significantly reduce development time and effort. For example, for web development, frameworks like Spring Boot provide a robust foundation and numerous out-of-the-box features, enabling rapid application development with minimal configuration.
// Example of a Spring Boot REST Controller
@RestController
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/products")
public List<Product> getAllProducts() {
return productService.getAllProducts();
}
// Additional controller methods
}
By leveraging such frameworks, developers can focus on implementing business logic rather than dealing with low-level technical details, thereby boosting productivity and minimizing unnecessary workload.
Automated Testing and Continuous Integration
Automated testing is indispensable for ensuring the reliability and quality of software. By writing comprehensive unit tests and integrating them into a continuous integration pipeline, developers can catch bugs early and prevent regressions. Tools like JUnit and Mockito are pivotal in creating robust test suites and mock objects for efficient unit testing.
// Example of a JUnit test for a simple method
public class StringUtilTest {
@Test
public void testStringLength() {
String str = "Hello, World!";
assertEquals(13, StringUtil.getLength(str));
}
// Additional test cases
}
Integrating automated testing with continuous integration systems such as Jenkins or CircleCI streamlines the development workflow by automatically running tests upon code changes. This facilitates early detection of issues, ensuring that the codebase remains stable and reducing the need for manual intervention.
Embrace Functional Programming Paradigms
Java supports functional programming paradigms through features like lambdas, streams, and optional types introduced in Java 8 and subsequent versions. Embracing these paradigms enables developers to write more expressive and concise code, particularly for operations involving collections and data transformations.
// Example of using streams for data manipulation
List<String> products = Arrays.asList("Laptop", "Phone", "Tablet");
List<String> filteredProducts = products.stream()
.filter(product -> product.startsWith("L"))
.collect(Collectors.toList());
By leveraging functional programming constructs, developers can enhance code readability and maintainability, ultimately reducing the cognitive load and workload associated with complex data processing tasks.
Prioritize Code Refactoring and Maintenance
Maintaining a clean and organized codebase is essential for long-term productivity. Regular code refactoring and maintenance activities prevent technical debt from accumulating and ensure that the code remains comprehensible and maintainable.
Adopting tools such as SonarQube can assist in analyzing code quality and identifying areas for improvement. Additionally, adhering to well-established design patterns and best practices, such as SOLID principles, fosters a codebase that is easier to extend and modify.
// Example of refactored code using Extract Method refactoring
public class OrderProcessor {
public void processOrder(Order order) {
validateOrder(order);
// Additional processing logic
}
private void validateOrder(Order order) {
// Validation logic
}
}
By prioritizing code refactoring and maintenance, developers can mitigate potential future issues and minimize the effort required for understanding and modifying the code.
Closing the Chapter
In conclusion, maximizing productivity and minimizing workload in Java development necessitates the adoption of efficient strategies and best practices. By leveraging modern Java features, embracing libraries and frameworks, incorporating automated testing, embracing functional programming paradigms, and prioritizing code refactoring and maintenance, developers can streamline their workflow and deliver high-quality software with reduced effort.
Java's versatility and vast ecosystem provide developers with an array of tools and techniques to enhance productivity and minimize unnecessary workload. By continuously refining development practices and staying abreast of advancements in the Java ecosystem, developers can establish a sustainable framework for efficient and effective software development.
Embracing these strategies not only benefits individual developers but also contributes to the overall success of projects and organizations, fostering a culture of excellence and innovation in Java development.
By implementing these efficient strategies, Java developers can elevate their productivity and ensure the delivery of high-quality software. If you're interested in diving deeper into Java optimization, check out Oracle's official documentation for comprehensive insights into the latest features and best practices.