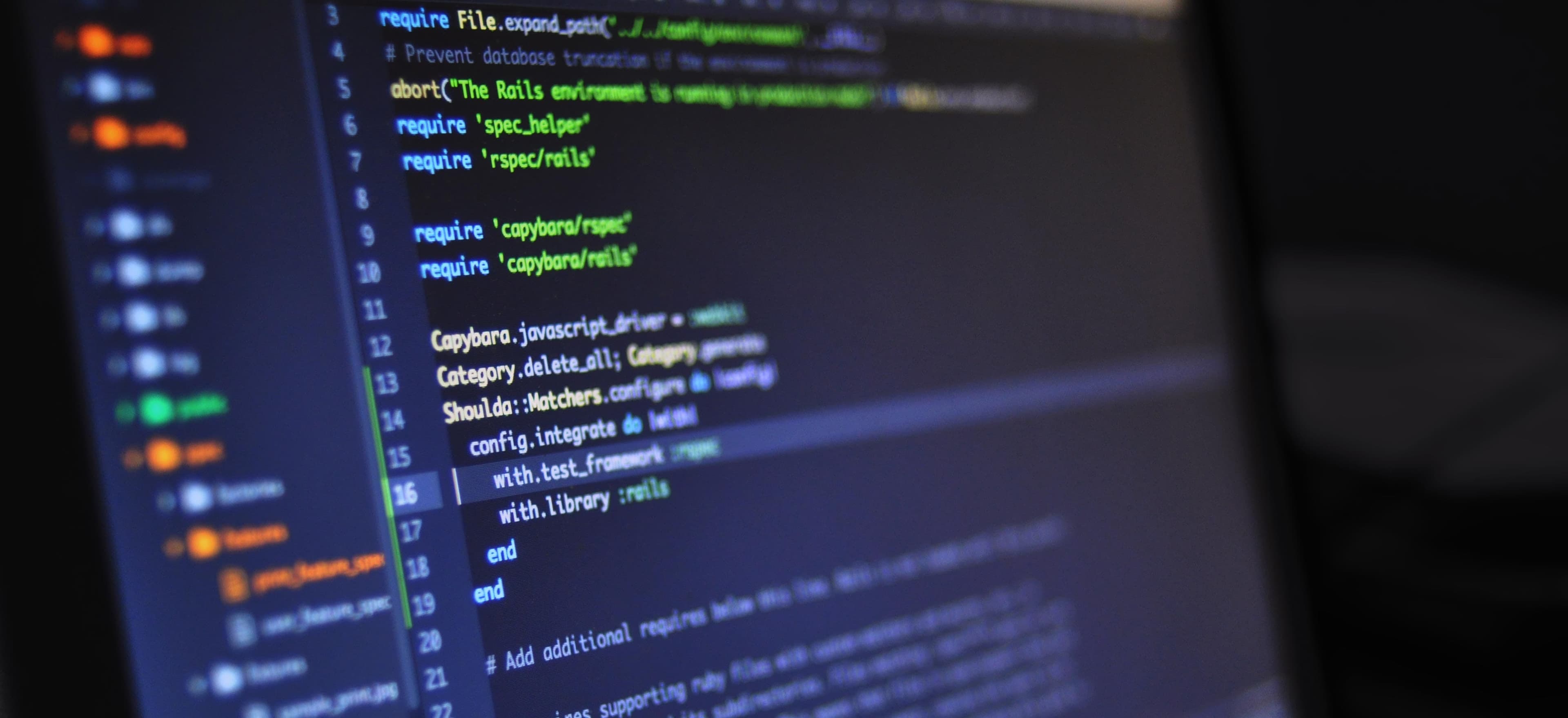
- Published on
Understanding Covariant and Contravariant Subtyping in Java
When working with Java generics, it’s essential to grasp the concepts of covariant and contravariant subtyping. These concepts are fundamental for writing flexible and type-safe code that can handle different types of data. In this article, we'll delve into the details of covariant and contravariant subtyping, understand their significance, and see how they can be leveraged in Java programming.
What is Covariant and Contravariant Subtyping?
Covariant and contravariant subtyping are concepts that come into play when dealing with subtyping relationships between parameterized types. In the context of Java generics, covariant and contravariant subtyping refer to the relationship between parameterized types with wildcard and the corresponding subtypes.
Covariant Subtyping
Covariant subtyping establishes a relationship where a parameterized type with a wildcard (?) can be replaced with its subtype. In other words, if A
is a subtype of B
, then List<A>
is considered a subtype of List<B>
. This relationship preserves the direction of subtyping, which means data can flow in the same direction as the subtyping relationship.
Contravariant Subtyping
Contravariant subtyping, on the other hand, involves a relationship where a parameterized type with a wildcard (?) can be replaced with its supertype. This means that if A
is a subtype of B
, then Consumer<B>
is considered a subtype of Consumer<A>
. Contrary to covariant subtyping, contravariant subtyping allows data to flow in the opposite direction of the subtyping relationship.
Importance of Covariant and Contravariant Subtyping
Understanding covariant and contravariant subtyping is crucial for designing APIs and writing flexible and reusable code. By leveraging these concepts, you can ensure that your code can handle various types without sacrificing type-safety. This is particularly useful when working with collections, functional interfaces, and other generic types where dealing with different subtypes is a common scenario.
Moreover, covariant and contravariant subtyping play a significant role in ensuring that the Liskov substitution principle is upheld. This principle states that objects of a superclass should be replaceable with objects of its subclass without affecting the functionality and correctness of the program. Covariant and contravariant subtyping allow for a more nuanced and accurate representation of these relationships within the type system.
Using Covariant and Contravariant Subtyping in Java
Now that we understand the significance of covariant and contravariant subtyping, let’s explore how we can leverage these concepts in Java programming.
Covariant Subtyping in Java Generics
In Java, covariant subtyping is directly supported when working with arrays. Arrays in Java exhibit covariant behavior, which means that if A
is a subtype of B
, then A[]
is considered a subtype of B[]
. Let's look at an example to illustrate this behavior:
public class CovariantSubtypingExample {
public static void main(String[] args) {
String[] strings = new String[]{"Hello", "World"};
Object[] objects = strings; // Covariant assignment
objects[1] = 123; // Compiles but throws ArrayStoreException at runtime
}
}
In the above code snippet, the strings
array, which is an array of String
, is assigned to the objects
array, which is an array of Object
. This is allowed due to covariant subtyping. However, as seen in the subsequent line, attempting to store an integer in the objects
array results in an ArrayStoreException
at runtime, highlighting the importance of understanding array covariance and its potential pitfalls.
Covariant Wildcard in Generics
When it comes to Java generics, wildcard types enable covariant subtyping. The wildcard <?>
allows for flexibility in working with unknown types while maintaining type safety. Let's consider the use of a wildcard in a printAll
method which prints all elements in a list:
public class CovariantWildcardExample {
public static void printAll(List<?> list) {
for (Object o : list) {
System.out.println(o);
}
}
public static void main(String[] args) {
List<String> strings = Arrays.asList("Java", "is", "great");
printAll(strings); // Covariant argument
}
}
In this example, the printAll
method accepts a List
with a wildcard <?>
type, making it possible to pass a List<String>
as an argument. This showcases covariant subtyping and allows the printAll
method to work with different types of List
s, while preserving type safety.
Contravariant Subtyping in Functional Interfaces
Contravariant subtyping is often observed in the context of functional interfaces wherein the input parameter of a method accommodates contravariant behavior. The Consumer
functional interface from the java.util.function
package can serve as an example. Let's take a look at a contravariant use case:
public class ContravariantFunctionalInterfaceExample {
public static void processValue(Consumer<? super Integer> consumer, int value) {
consumer.accept(value); // Contravariant usage
}
public static void main(String[] args) {
Consumer<Number> numberConsumer = n -> System.out.println("Consuming number: " + n);
processValue(numberConsumer, 10); // Contravariant argument
}
}
In this example, the processValue
method accepts a Consumer
with a wildcard ? super Integer
type. Despite the numberConsumer
being of type Consumer<Number>
, it can be passed as an argument to processValue
due to contravariant subtyping. This showcases how contravariant subtyping allows for more flexibility in accepting different subtypes without compromising type safety.
Lessons Learned
In conclusion, understanding covariant and contravariant subtyping is crucial for writing robust and flexible Java code, particularly when working with generics and inheritance. These concepts enable you to design APIs that can handle a wide range of data types while ensuring type safety and adherence to the Liskov substitution principle.
By mastering these concepts, you can make your code more adaptable and reusable, ultimately leading to more maintainable and resilient software. So next time you find yourself in a scenario where you need to handle different types or accommodate various subtypes, remember to leverage covariant and contravariant subtyping to streamline your design and ensure the integrity of your code. Happy coding!
These concepts can significantly improve Java programming practices. If you are interested in diving deeper into Java generics, particularly its type variance, consider reading through Oracle's official documentation.
Checkout our other articles