Maximizing Flexibility in Structural Design with Composite Patterns
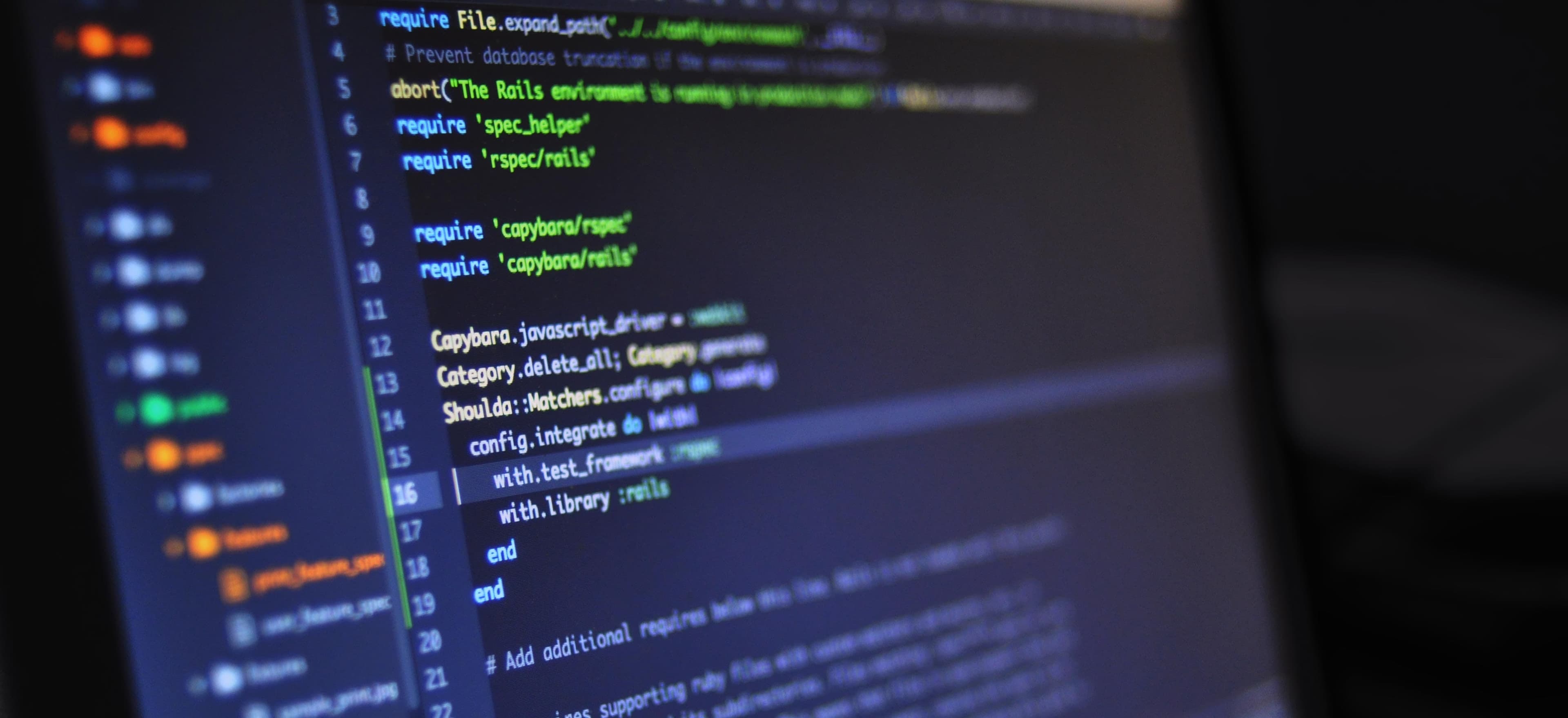
- Published on
Maximizing Flexibility in Structural Design with Composite Patterns
In Java software development, mastering design patterns is essential for harnessing the full potential of the language. Among the various design patterns, the Composite pattern stands as a fundamental tool for creating flexible and maintainable object-oriented designs.
What is the Composite Pattern?
The Composite pattern is a structural design pattern that allows you to compose objects into tree structures to represent part-whole hierarchies. This pattern lets clients treat individual objects and compositions of objects uniformly.
By using the Composite pattern, you can create hierarchical structures from individual objects and treat both the whole and the parts uniformly, making the client code simpler and more flexible.
When to Use the Composite Pattern
The Composite pattern is particularly useful when dealing with hierarchies, such as representing a file system, graphical user interface elements, or organization structures.
In Java, the Composite pattern is especially powerful when dealing with complex GUI components or managing hierarchical data structures such as trees.
Implementing the Composite Pattern
Let's dive into an example to illustrate the implementation of the Composite pattern in Java.
Suppose we want to model a simple directory structure using the Composite pattern.
First, let's define a common component interface FileSystemItem
:
public interface FileSystemItem {
void printName();
}
Next, we'll create File
and Directory
classes implementing the FileSystemItem
interface:
public class File implements FileSystemItem {
private String name;
public File(String name) {
this.name = name;
}
public void printName() {
System.out.println("File: " + name);
}
}
public class Directory implements FileSystemItem {
private String name;
private List<FileSystemItem> children = new ArrayList<>();
public Directory(String name) {
this.name = name;
}
public void add(FileSystemItem item) {
children.add(item);
}
public void printName() {
System.out.println("Directory: " + name);
for (FileSystemItem item : children) {
item.printName();
}
}
}
In this example, the File
and Directory
classes represent the leaf and composite components, respectively. The Directory
class can contain other FileSystemItem
s, allowing us to create a hierarchical structure.
Now, let's use these classes to create a sample directory structure:
FileSystemItem file1 = new File("file1.txt");
FileSystemItem file2 = new File("file2.txt");
FileSystemItem dir1 = new Directory("dir1");
dir1.add(file1);
dir1.add(file2);
FileSystemItem dir2 = new Directory("dir2");
dir2.add(dir1);
dir2.printName();
When running this code, the output will display the entire directory structure, demonstrating the power of the Composite pattern.
Benefits of the Composite Pattern
- Simplified Client Code: Clients can treat individual objects and compositions uniformly, simplifying client code that deals with complex hierarchies.
- Flexible and Scalable Design: The Composite pattern allows for easy addition of new component types and changes to the structure without affecting client code.
- Encapsulation of Complexity: The pattern encapsulates the complexities of the hierarchy within the components, promoting a clearer and more maintainable design.
Key Takeaways
The Composite pattern is a valuable tool in Java software development for creating flexible and maintainable object-oriented designs, especially when dealing with hierarchical structures.
By understanding and applying the Composite pattern, developers can simplify client code, maintain flexibility in design, and encapsulate complexities within the components, resulting in more robust and extensible software systems.
To delve deeper into design patterns, check out the official Design Patterns documentation provided by Oracle.
Mastering design patterns like the Composite pattern equips Java developers with a powerful arsenal for building elegant and maintainable software solutions.
Checkout our other articles