Configuring Apache Storm's KafkaBolt in Flux
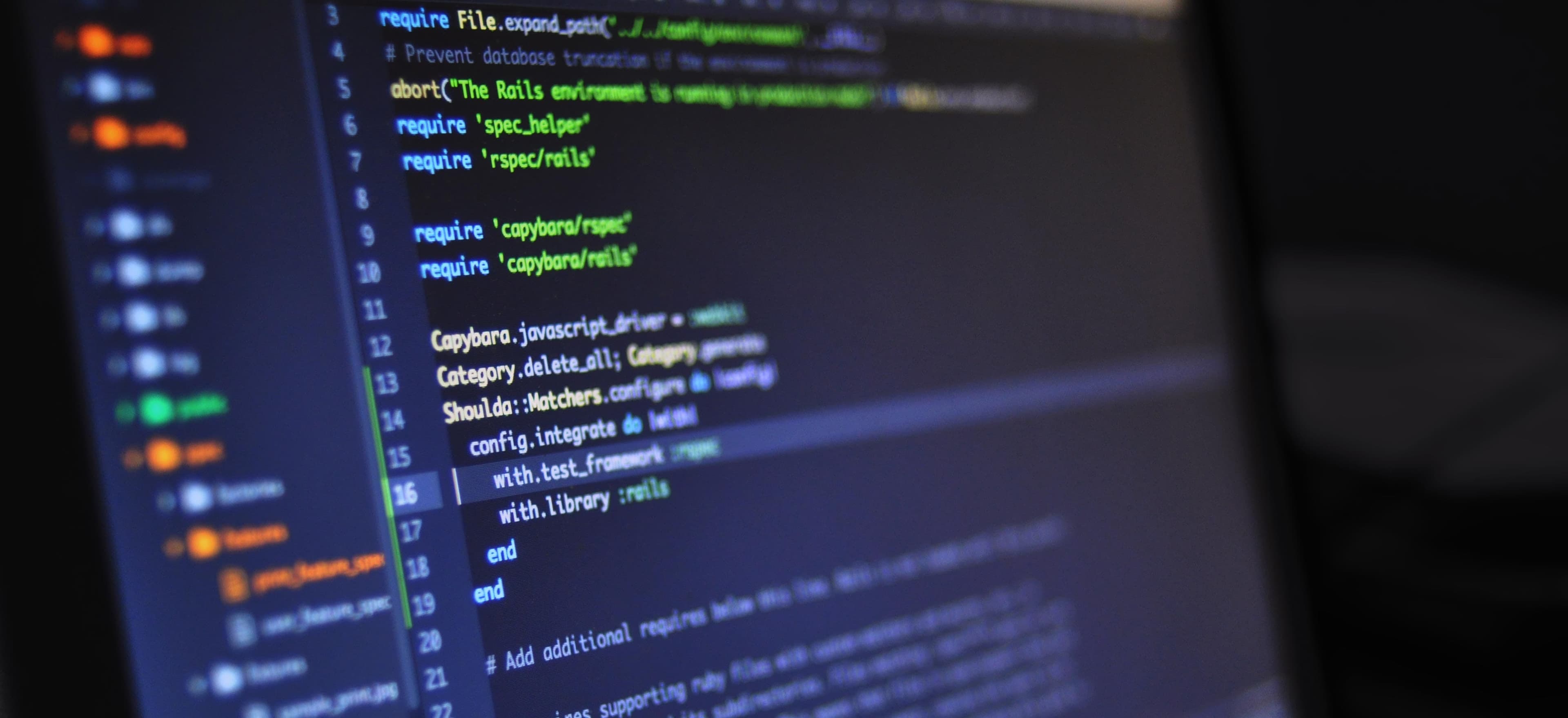
- Published on
Configuring Apache Storm's KafkaBolt in Flux
Apache Storm is a distributed real-time computation system. The KafkaBolt in Apache Storm allows you to write tuples to Kafka topics. Flux is a configuration-driven framework for specifying and wiring together topologies.
In this article, we'll walk through the configuration of the KafkaBolt in Apache Storm using Flux. We'll cover the necessary steps and provide code snippets for a clear understanding of the process.
Step 1: Add the KafkaBolt to your Flux Topology
To get started, you'll need to include the KafkaBolt component in your Flux topology. Here's an example of how to define the KafkaBolt component within the bolts section of your Flux file:
bolts:
- id: "kafka-bolt"
className: "org.apache.storm.kafka.bolt.KafkaBolt"
constructorArgs:
- ref: "kafka-bolt-properties"
In this snippet, we're defining a bolt with the id "kafka-bolt" and specifying its className as "org.apache.storm.kafka.bolt.KafkaBolt". We're also passing constructor arguments, specifically a reference to a KafkaBolt properties configuration.
Step 2: Configure the KafkaBolt Properties
Next, you'll need to define the KafkaBolt properties in the components section of your Flux file. Here's an example of how to configure the properties:
components:
- id: "kafka-bolt-properties"
className: "org.apache.storm.kafka.bolt.KafkaBolt$KafkaBoltConfig"
constructorArgs:
- "localhost:9092" # brokerList
- "your-topic" # topic
configMethods:
- name: "withTupleToKafkaMapper"
args:
- # Here you would specify your implementation of TupleToKafkaMapper
In this snippet, we're creating a component with the id "kafka-bolt-properties" and specifying its className as "org.apache.storm.kafka.bolt.KafkaBolt$KafkaBoltConfig". We're providing constructor arguments for the brokerList and topic, and we also have the option to configure a custom TupleToKafkaMapper.
Step 3: Define the TupleToKafkaMapper
The TupleToKafkaMapper interface allows you to specify how the tuples should be mapped to Kafka messages. You can customize this mapping based on your specific use case. Here's an example of how you might implement a TupleToKafkaMapper:
public class MyTupleToKafkaMapper implements TupleToKafkaMapper<String, String> {
@Override
public ProducerRecord<String, String> fromTuple(Tuple tuple) {
// Extract values from the tuple and create a ProducerRecord
String key = tuple.getStringByField("key");
String value = tuple.getStringByField("value");
return new ProducerRecord<>("your-topic", key, value);
}
}
In this example, we're creating a custom implementation of TupleToKafkaMapper that extracts key and value fields from the tuple and uses them to create a ProducerRecord for the specified topic.
Step 4: Register the TupleToKafkaMapper in the KafkaBolt properties
Finally, you'll need to register your custom TupleToKafkaMapper within the KafkaBolt properties configuration. Modify the Flux component configuration for the KafkaBolt properties to include your custom TupleToKafkaMapper:
configMethods:
- name: "withTupleToKafkaMapper"
args:
- # Specify the reference to your implementation of TupleToKafkaMapper
By specifying the reference to your custom TupleToKafkaMapper implementation here, you're instructing the KafkaBolt to use your custom mapping logic when writing tuples to Kafka.
To Wrap Things Up
In this article, we've outlined the process of configuring Apache Storm's KafkaBolt in a Flux topology. By following these steps, you can set up the KafkaBolt to write tuples to Kafka topics based on your specific requirements. Understanding and effectively configuring the KafkaBolt within a Flux topology is crucial for integrating Apache Storm with Kafka, and this guide can serve as a valuable reference to streamline the process.
For more information on Apache Storm and Flux, you can refer to the official documentation:
- Apache Storm Documentation
- Flux Documentation