The Pitfalls of Overusing Common Methods in Java
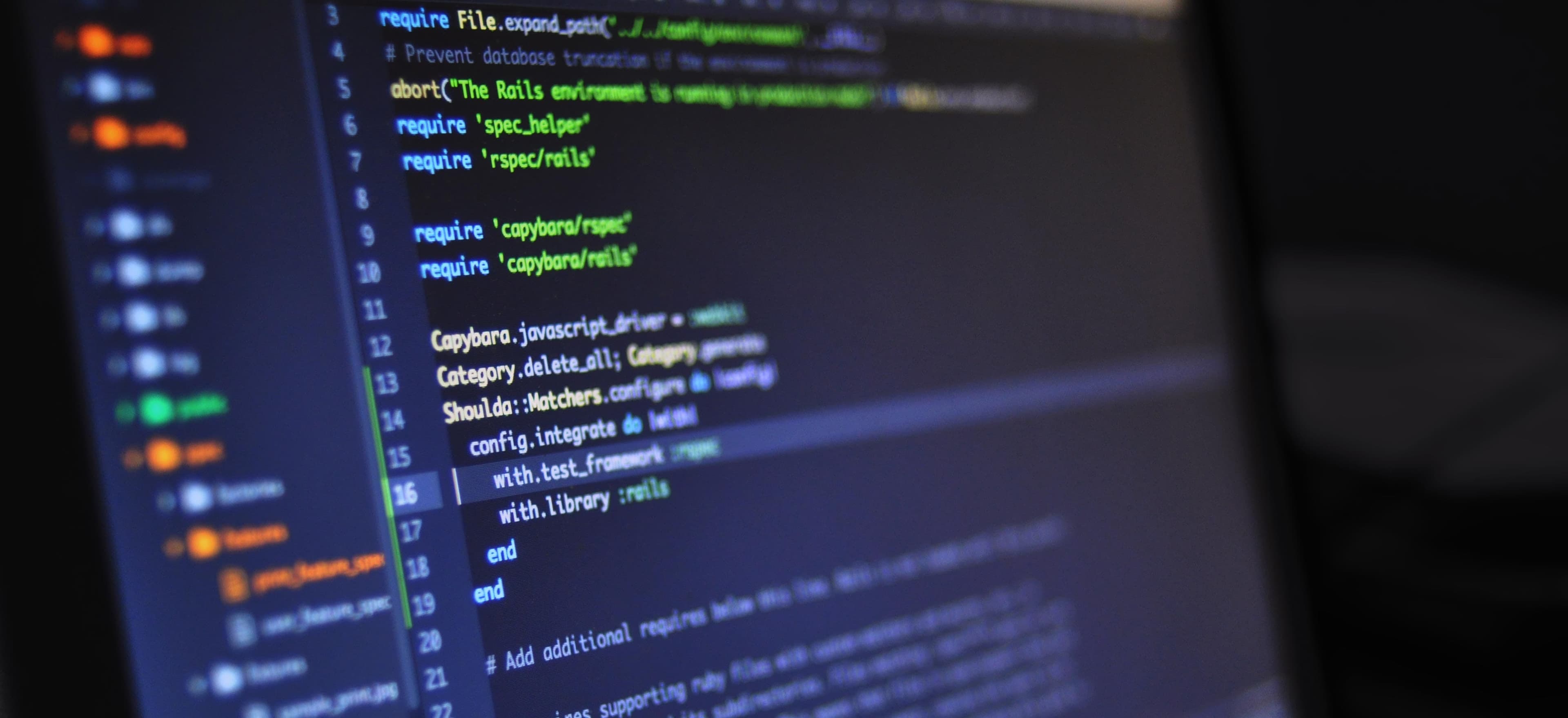
- Published on
The Pitfalls of Overusing Common Methods in Java
When working with Java, it's natural to reach for commonly used methods that have been around for years. However, overusing these methods can lead to a range of issues that can impact the efficiency and maintainability of your code. In this blog post, we'll explore some of the pitfalls of overusing common methods in Java and discuss alternative approaches for a more robust codebase.
The Dangers of Overreliance on Common Methods
Java provides developers with a wide array of built-in methods that cover many common use cases, such as sorting arrays, manipulating strings, and managing collections. While these methods can be incredibly useful, overreliance on them can lead to several pitfalls, including:
1. Loss of Performance Optimization
Common methods are designed to be generally applicable and cater to a wide range of scenarios. As a result, they might not be the most performant solution for a specific use case. Overusing common methods without considering the context of your application can lead to suboptimal performance.
2. Reduced Code Readability and Maintainability
When common methods are used extensively throughout your codebase, it can make the code less readable and harder to maintain. Overuse of these methods might lead to less intuitive code that is difficult for other developers to understand and modify.
3. Limited Flexibility
Common methods have a predefined set of behaviors, and using them excessively can limit the flexibility of your code. This can make it challenging to adapt to changing requirements or optimize for specific edge cases.
Example of Overusing a Common Method: Arrays.sort()
Let's consider the Arrays.sort()
method, which is commonly used for sorting arrays in Java. While this method is convenient and efficient for general sorting needs, overusing it without understanding its underlying implementation can lead to issues.
int[] numbers = {5, 2, 8, 1, 9};
Arrays.sort(numbers);
At first glance, using Arrays.sort()
seems like a straightforward solution for sorting an array of integers. However, if the array is frequently modified and re-sorted, using a different approach such as a data structure with built-in sorting capabilities, like a priority queue, might offer better performance.
How to Avoid Overreliance on Common Methods
To mitigate the pitfalls of overusing common methods in Java, consider the following strategies:
1. Understand the Underlying Implementation
Before using a common method, take the time to understand how it works under the hood. This knowledge will enable you to make informed decisions about when to use the method and when an alternative approach might be more suitable.
2. Evaluate Performance Implications
Consider the performance implications of using common methods in your specific use case. In some scenarios, a custom solution tailored to your application's needs might outperform a generic method.
3. Embrace Custom Implementations When Appropriate
Don't hesitate to create custom implementations tailored to your specific requirements. Custom implementations can offer better control, improved performance, and increased flexibility compared to blindly relying on common methods.
Lessons Learned
While common methods in Java provide convenient solutions for many programming tasks, overusing them without consideration of their implications can lead to suboptimal code. By understanding the pitfalls of overreliance on common methods and embracing alternative approaches, you can develop a more efficient and maintainable Java codebase.
To further enhance your understanding of Java best practices and coding techniques, consider exploring Java's official documentation and engaging with the vibrant Java community through platforms like Stack Overflow.
Remember, the key to writing robust Java code lies in striking a balance between leveraging common methods and implementing custom solutions tailored to your specific requirements.