Simplifying OIDC Integration in Spring Security 5.0
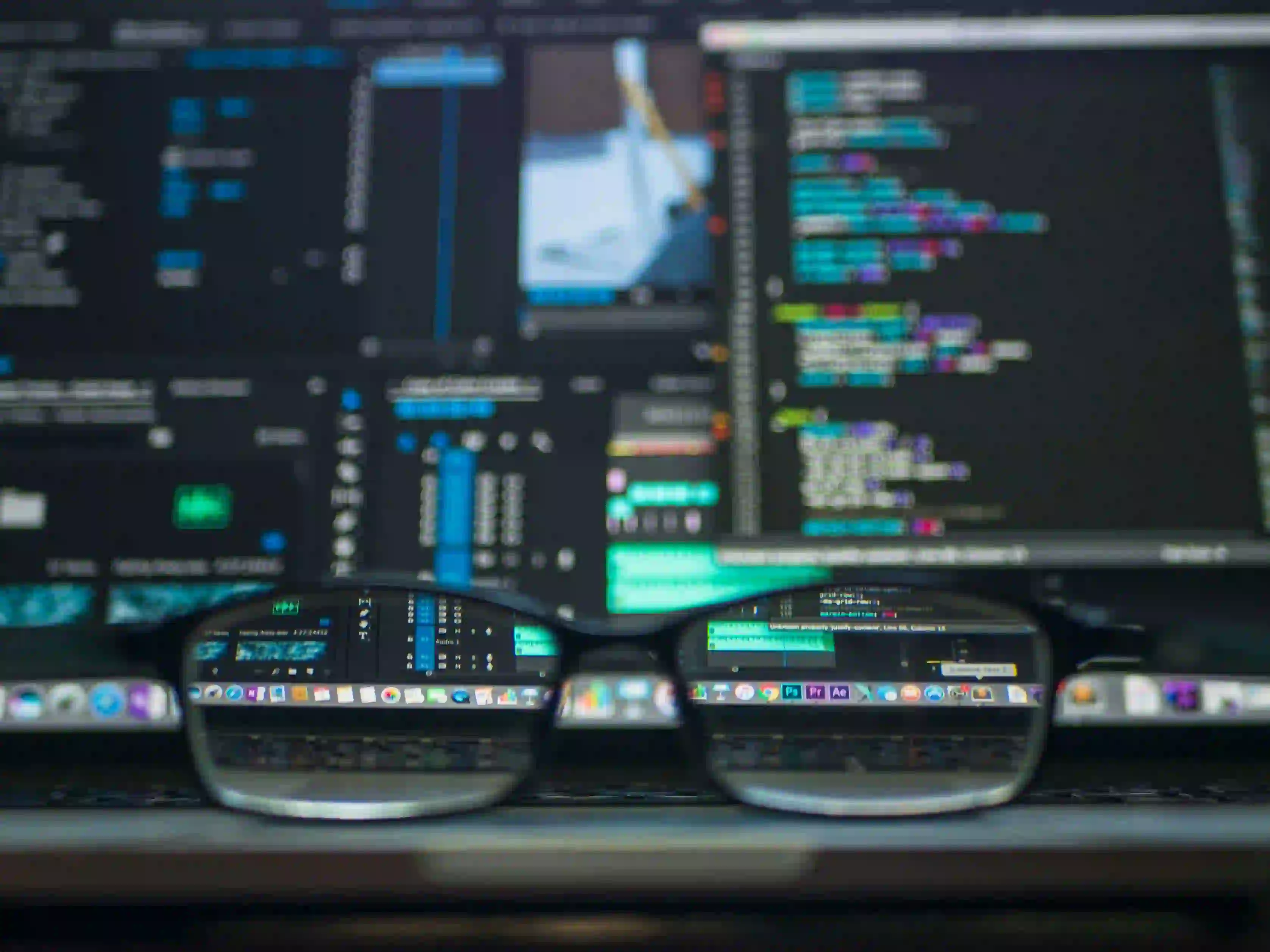
Simplifying OIDC Integration in Spring Security 5.0
Spring Security is a powerful and customizable authentication and access control framework for Java applications. With the release of Spring Security 5.0, integrating OpenID Connect (OIDC) into your Spring application has become even simpler. In this blog post, we will explore the seamless OIDC integration in Spring Security 5.0 and understand how it makes the process easier for developers.
What is OpenID Connect (OIDC)?
OpenID Connect is an authentication layer built on top of the OAuth 2.0 protocol. It allows clients to verify the identity of the end-user based on the authentication performed by an authorization server, as well as to obtain basic profile information about the end-user in an interoperable and REST-like manner. OIDC is widely used for single sign-on (SSO) and is the preferred choice for identity management in modern applications.
OIDC Integration in Spring Security 5.0
With the introduction of the spring-security-oauth2-client
module, Spring Security 5.0 now provides built-in support for OIDC, making it easier for developers to secure their applications using OIDC providers such as Google, Okta, or Keycloak.
1. Configuring OIDC Client in Spring Security
To integrate OIDC authentication into a Spring application, you first need to configure an OIDC client registration. This can be done in the application properties file or programmatically as follows:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2Login(oauth2 -> oauth2
.clientRegistrationRepository(clientRegistrationRepository())
);
}
private ClientRegistrationRepository clientRegistrationRepository() {
return new InMemoryClientRegistrationRepository(this.googleClientRegistration());
}
private ClientRegistration googleClientRegistration() {
return ClientRegistration.withRegistrationId("google")
.clientId("google-client-id")
.clientSecret("google-client-secret")
.clientName("Google")
.redirectUriTemplate("{baseUrl}/{action}/oauth2/code/{registrationId}")
.authorizationGrantType(AuthorizationGrantType.AUTHORIZATION_CODE)
.userInfoUri("https://www.googleapis.com/oauth2/v3/userinfo")
.userNameAttributeName(IdTokenClaimNames.SUB)
.clientName("Google")
.build();
}
}
In the above code snippet, we configure an OIDC client for Google using ClientRegistration
and ClientRegistrationRepository
.
2. Handling OIDC Authentication and User Information
After configuring the OIDC client, Spring Security handles the entire OIDC authentication flow seamlessly. When a user tries to access a protected resource and is not authenticated, they are redirected to the OIDC identity provider's login page. Once authenticated, the user's profile information, including claims from the ID token, is retrieved and made available within the application. This information can be accessed as shown below:
@RestController
public class UserController {
@GetMapping("/user/profile")
public String getUserProfile(OAuth2AuthenticationToken token) {
OAuth2User user = token.getPrincipal();
return "Hello, " + user.getAttribute("name");
}
}
In this code snippet, we retrieve the user's name from the OIDC user information using OAuth2AuthenticationToken
.
3. Securing Endpoints with OIDC
Securing endpoints based on user roles and permissions is an essential part of application security. With OIDC integration in Spring Security, you can easily restrict access to endpoints based on the user's OIDC attributes and claims:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests(authorize -> authorize
.antMatchers("/admin/**").hasAuthority("ROLE_ADMIN")
.anyRequest().authenticated()
)
.oauth2Login()
.oauth2Login().userInfoEndpoint().userService(new CustomOAuth2UserService());
}
}
In this example, we restrict access to the /admin
endpoints based on the user's role using the hasAuthority
method.
Closing the Chapter
In conclusion, Spring Security 5.0 has made OpenID Connect integration seamless and straightforward. With built-in support for OIDC clients, handling OIDC authentication flows, and securing endpoints based on OIDC attributes, Spring Security simplifies the process of integrating OIDC into Java applications. Developers can now focus on building secure and robust applications without getting bogged down by the complexities of OIDC integration.
If you're interested in exploring OIDC integration further, you can refer to the official Spring Security documentation.
In conclusion, OIDC integration in Spring Security 5.0 is a game-changer for Java developers. With a few configurations and minimal code changes, you can have a robust OIDC authentication mechanism in your Spring applications. Embrace the simplicity, enhance the security, and elevate the user experience with OIDC integration in Spring Security 5.0.