Enhancing Code Readability with Dependency Injection
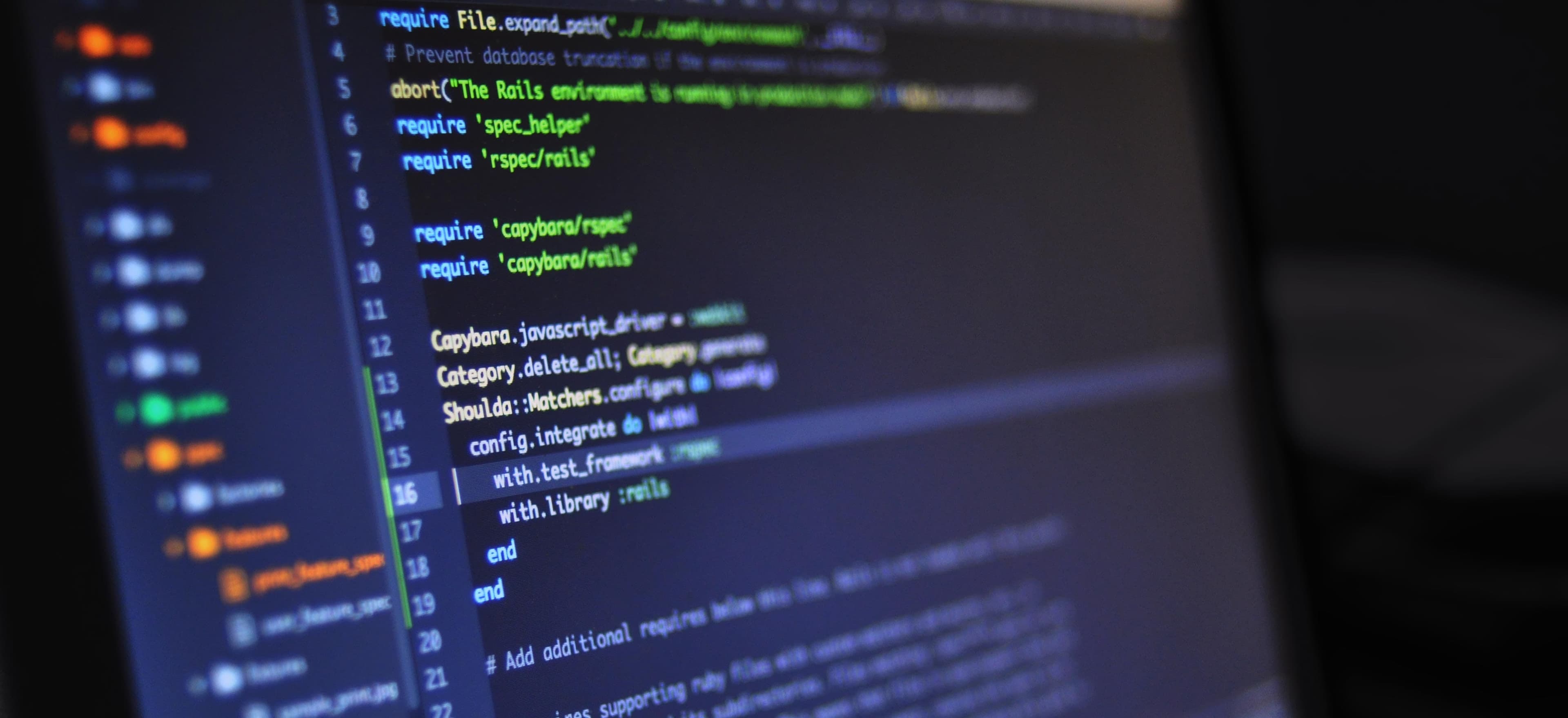
- Published on
Enhancing Code Readability with Dependency Injection
In the world of Java development, writing clean, maintainable, and readable code is essential for long-term success. One of the key principles in achieving this is the use of dependency injection. By leveraging dependency injection, developers can improve the readability and maintainability of their code while also promoting modularity and testability.
What is Dependency Injection?
Dependency injection is a design pattern that allows the removal of hard-coded dependencies in an application by providing those dependencies externally. This external provision of dependencies decouples the dependent code from the actual implementation, making it easier to manage and test.
In the context of Java, dependency injection is commonly implemented through frameworks such as Spring and Google Guice. These frameworks provide the necessary infrastructure to manage and inject dependencies into Java objects.
Why is Dependency Injection Important for Readable Code?
1. Decoupling Dependencies
When dependencies are hard-coded within a class, it becomes tightly coupled to those dependencies. This not only makes the class less flexible but also decreases its readability. By using dependency injection, the dependencies are provided from the outside, leading to looser coupling and more readable code.
2. Improved Modularity
Dependency injection promotes modularity by allowing components to be developed and tested independently. This, in turn, enhances readability as each component can be focused on without the need to understand the intricacies of its dependencies.
3. Easier Testing
With dependency injection, it becomes easier to test individual components in isolation. By providing mock or stub implementations of dependencies, unit testing is streamlined, leading to more readable and understandable test cases.
How to Use Dependency Injection in Java
Let's take a look at how dependency injection can be implemented in Java using the Spring Framework.
Step 1: Define a Service Interface
public interface UserService {
void addUser(User user);
List<User> getUsers();
}
Step 2: Implement the Service Interface
@Service
public class UserServiceImpl implements UserService {
private UserRepository userRepository;
@Autowired
public UserServiceImpl(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Implement methods
}
In this example, the UserServiceImpl
class depends on the UserRepository
interface. By using the @Autowired
annotation, we are telling the Spring framework to inject an instance of UserRepository
when creating an instance of UserServiceImpl
.
Step 3: Configuration
@Configuration
@ComponentScan(basePackages = "com.example.services")
public class AppConfig {
// Configuration
}
In the configuration class, we define the base package for component scanning using the @ComponentScan
annotation. This tells Spring where to look for components that require dependency injection.
Step 4: Utilize the Service
@RestController
public class UserController {
private UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
// Use userService methods
}
In the UserController
, we inject the UserService
using the @Autowired
annotation, allowing us to utilize the UserService
without explicitly creating an instance of UserServiceImpl
.
By following these steps, we have implemented dependency injection using the Spring framework, which enhances the readability of our code by explicitly defining and injecting dependencies.
Best Practices for Dependency Injection
While leveraging dependency injection, it’s important to adhere to best practices to maximize the benefits of this design pattern.
1. Constructor Injection vs. Setter Injection
Constructor Injection: This involves injecting dependencies through the class constructor. It ensures that the required dependencies are provided when an instance is created, resulting in a clear and immutable object state.
Setter Injection: This involves injecting dependencies through setter methods. While it provides flexibility, it can lead to a partially initialized object state and should be used judiciously.
It’s best to favor constructor injection for mandatory dependencies and setter injection for optional dependencies.
2. Interface-Based Programming
Programming to interfaces rather than concrete implementations is a fundamental best practice that aligns with dependency injection. By depending on interfaces, the code becomes more flexible, maintainable, and readable.
3. Single Responsibility Principle
Adhering to the single responsibility principle while designing components and their dependencies leads to cleaner and more readable code. Each component should have a clear and singular purpose, making it easier to understand and maintain.
My Closing Thoughts on the Matter
Dependency injection plays a significant role in enhancing code readability by promoting decoupling, modularity, and testability. By leveraging frameworks like Spring and adhering to best practices, Java developers can consistently write more maintainable and readable code. This results in improved collaboration, easier maintenance, and overall higher quality software.
By incorporating dependency injection into your Java projects, you pave the way for code that is not only readable and maintainable but also scalable and adaptable to changing requirements.