Maximizing Performance with PL/Java Functions
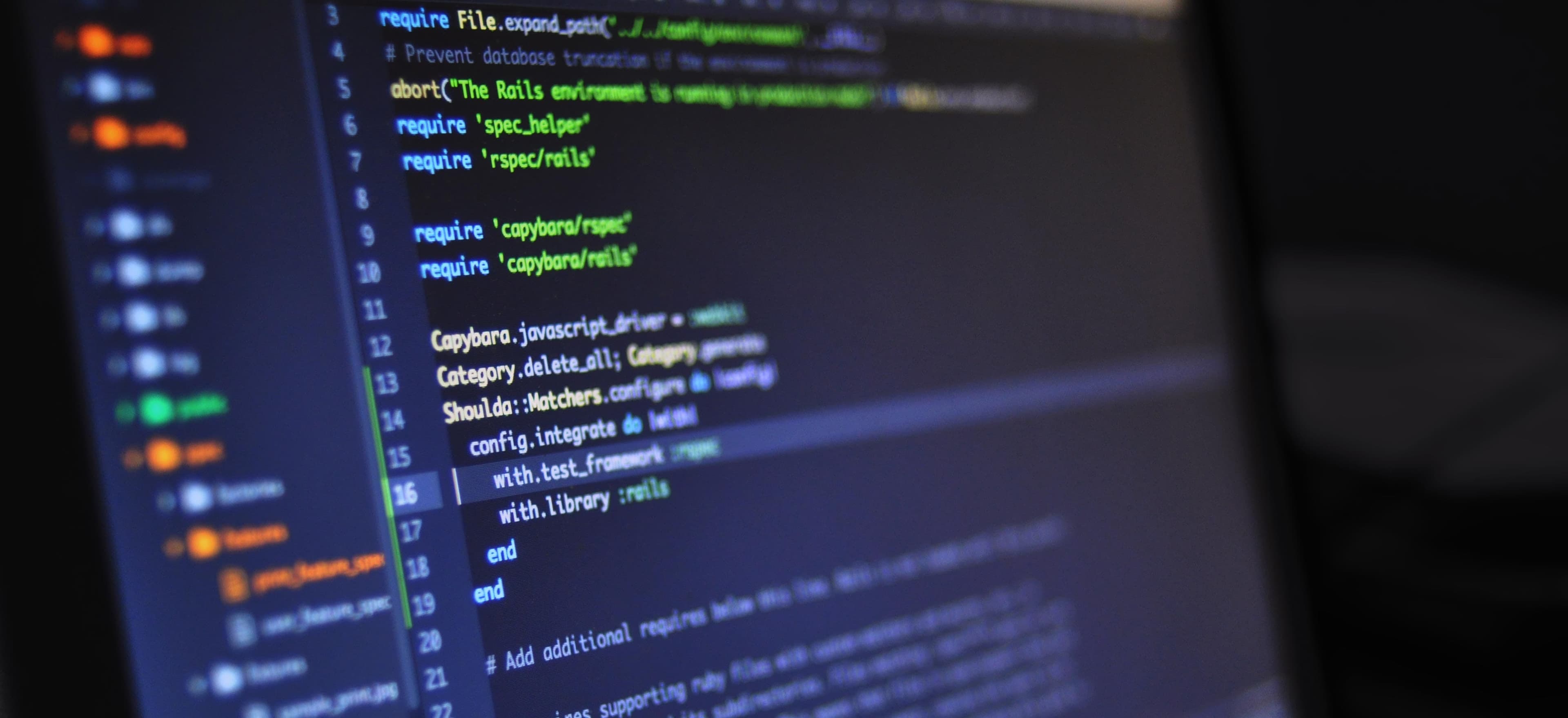
- Published on
Boosting Java Performance with PL/Java Functions
In the realm of software development, performance is a critical factor that can make or break an application. Java, with its robust features and widespread use, often finds itself at the forefront of performance optimization tactics. In this blog post, we will explore how PL/Java functions can be leveraged to maximize performance in Java applications.
Understanding PL/Java Functions
PL/Java is a procedural language extension for PostgreSQL that allows developers to write stored procedures, triggers, and functions in Java. By combining the power of Java with the flexibility of PostgreSQL, developers can create high-performance database functions that execute complex logic directly within the database server.
Benefits of PL/Java Functions
-
Performance: PL/Java functions can significantly boost performance by reducing data transfer between the database server and the application. By executing logic directly in the database, unnecessary data movement is eliminated, resulting in faster processing times.
-
Security: Using PL/Java functions can enhance security by encapsulating sensitive business logic within the database, reducing the risk of exposure to unauthorized access.
-
Code Reusability: By encapsulating frequently used logic within PL/Java functions, developers can promote code reusability across multiple database operations, leading to cleaner and more maintainable code.
Maximizing Performance with PL/Java Functions
Let's delve into some key strategies for maximizing performance with PL/Java functions.
Utilize Set-Based Operations
When writing PL/Java functions, favor set-based operations over iterative processing. Set-based operations, such as using SQL's JOIN
, GROUP BY
, and aggregate functions, are optimized within the database engine and can significantly enhance performance.
Example:
// Avoid using iterative processing like this
int total = 0;
for (int value : values) {
total += value;
}
// Instead, leverage set-based operations
SELECT SUM(value) FROM table;
In the above example, using a SUM
aggregate function within a SQL query can yield superior performance compared to manually summing values in a loop.
Optimize Data Access
Efficient data access is crucial for maximizing performance with PL/Java functions. Minimize unnecessary data retrieval and manipulation by employing efficient SQL queries, utilizing appropriate indexes, and considering the use of caching mechanisms.
Example:
// Inefficient data access
SELECT * FROM users WHERE age > 25;
// Optimize data access with appropriate indexing
CREATE INDEX idx_age ON users(age);
SELECT * FROM users WHERE age > 25;
In this example, creating an index on the age
column can greatly improve the performance of the query by facilitating faster data retrieval.
Leverage Connection Pooling
When interacting with external resources or services from PL/Java functions, consider utilizing connection pooling to manage database connections efficiently. Connection pooling minimizes the overhead of creating and destroying database connections, leading to improved performance and resource utilization.
Example (using HikariCP):
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost/mydb");
config.setUsername("username");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
By configuring a connection pool with HikariCP, PL/Java functions can efficiently manage database connections, enhancing overall performance.
Implement Proper Exception Handling
Exception handling is crucial for maintaining the robustness and reliability of PL/Java functions. Properly handle exceptions to prevent unexpected failures and ensure graceful error recovery.
Example:
try {
// Perform database operation
} catch (SQLException ex) {
// Handle exception
ex.printStackTrace();
}
In this example, catching and handling SQLException
ensures that any database-related exceptions are gracefully managed within the PL/Java function.
Utilize Inline SQL Execution
Opt for inline SQL execution within PL/Java functions to improve performance. Inline SQL execution allows queries to be directly embedded in Java code, eliminating the overhead of preparing and executing statements.
Example:
// Inline SQL execution
@Function
public static int getTotalUsers() throws SQLException {
try (Connection conn = DriverManager.getConnection(url, user, password)) {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT COUNT(*) FROM users");
if (rs.next()) {
return rs.getInt(1);
}
}
return 0;
}
In this example, the SQL query is directly executed within the Java function, avoiding the need for explicit statement preparation.
Lessons Learned
In conclusion, PL/Java functions offer a powerful mechanism for maximizing performance in Java applications, particularly when interacting with PostgreSQL. By employing set-based operations, optimizing data access, leveraging connection pooling, implementing proper exception handling, and utilizing inline SQL execution, developers can unlock the full potential of PL/Java functions to enhance performance and efficiency.
Optimizing performance with PL/Java functions is an essential consideration for Java developers seeking to build high-performing and scalable applications.
Now it's over to you - have you used PL/Java functions to maximize performance in your applications? Feel free to share your experiences and insights in the comments below.
Start harnessing the power of PL/Java functions today for a performance boost in your Java applications!