Preventing Java Code Injection Attacks
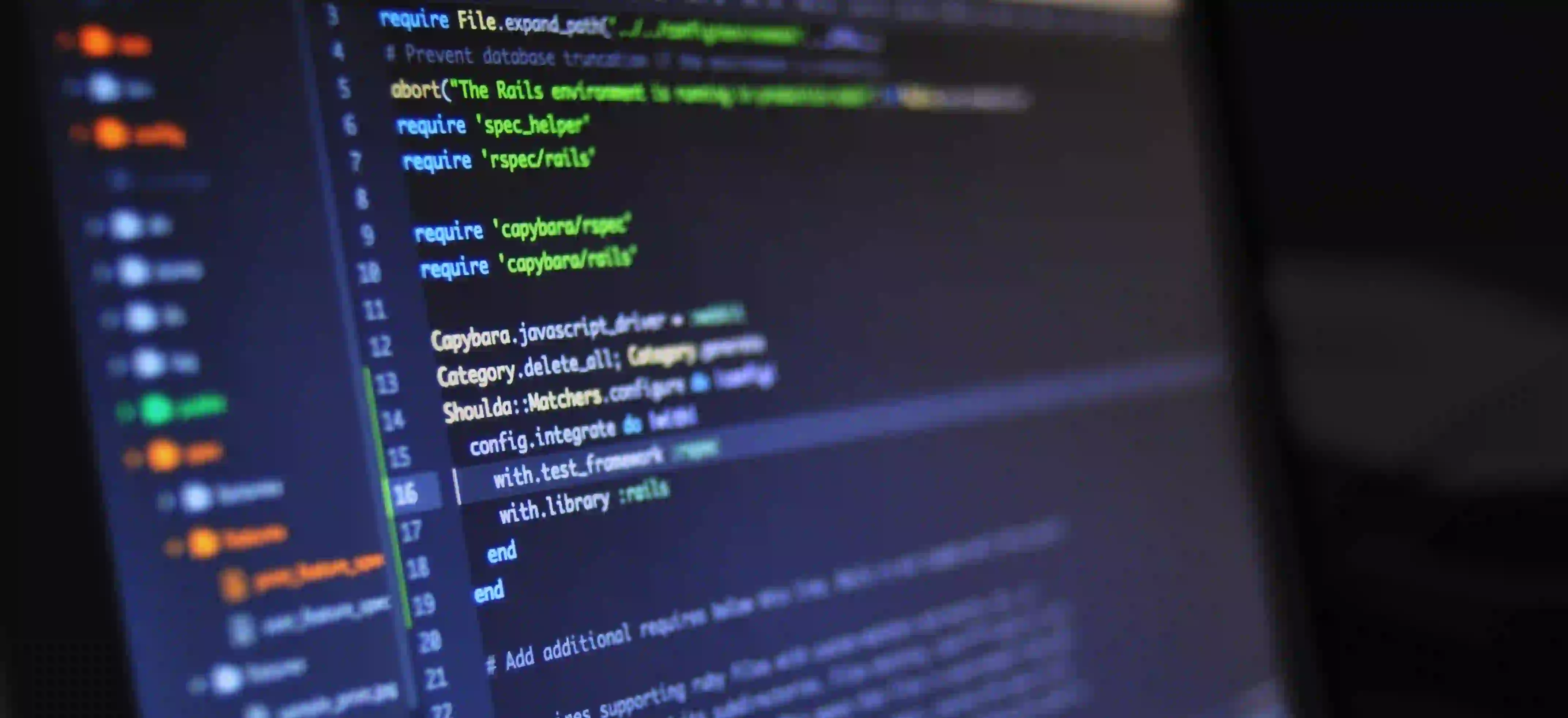
Preventing Java Code Injection Attacks
In the world of web development, security is paramount. One common security threat that developers face is code injection attacks. These attacks occur when untrusted data is sent to an interpreter as part of a command or query. In the case of Java applications, code injection attacks can have severe consequences, including unauthorized access, data theft, and system compromise.
In this article, we'll explore what Java code injection attacks are, common types of code injection attacks, and best practices for preventing them in your Java applications.
Understanding Java Code Injection Attacks
Java code injection attacks occur when untrusted input is used to manipulate the behavior of the Java application. This can happen in various ways, including:
-
SQL Injection: Attackers inject malicious SQL code into input fields, which can manipulate the backend SQL queries and potentially access or modify the database.
-
Command Injection: Attackers inject malicious commands into input fields that are used to execute system commands, potentially leading to unauthorized access or system compromise.
-
Object Injection: Attackers manipulate the serialized Java objects to execute arbitrary code on the server, leading to remote code execution.
Common Prevention Techniques
1. Input Validation and Sanitization
The first line of defense against code injection attacks is input validation and sanitization. Always validate and sanitize any input received from users or external systems before using it in your Java application. This can include using whitelists, blacklists, or input validation libraries like Apache Commons Validator to ensure that the input adheres to expected data formats and ranges.
2. Prepared Statements and Parameterized Queries
When dealing with databases, it's crucial to use prepared statements and parameterized queries instead of concatenating user input into SQL queries. This helps prevent SQL injection attacks by ensuring that user input is treated as data rather than executable SQL code.
Here's an example of using a prepared statement with JDBC:
String username = request.getParameter("username");
String password = request.getParameter("password");
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, username);
statement.setString(2, password);
ResultSet result = statement.executeQuery();
In this example, user input is passed as parameters to the prepared statement rather than being directly concatenated into the SQL query.
3. Output Escaping
When displaying user-supplied data in web pages, it's essential to escape the output to prevent cross-site scripting (XSS) attacks. Use libraries like OWASP's ESAPI to escape user input before rendering it in HTML pages to avoid injecting malicious scripts.
Preventing Object Injection
Java is susceptible to object injection attacks when deserializing objects. It's crucial to validate and sanitize any serialized objects to prevent attackers from injecting malicious code into your application.
1. Use Safe Serialization Mechanisms
Prefer using safe serialization libraries like Google's Protocol Buffers or JSON over Java's default serialization mechanism, which can be vulnerable to object injection attacks. These libraries provide more control over the serialization process and are less prone to security vulnerabilities.
2. Implement Serialization Filters
If you must use Java's default serialization mechanism, implement serialization filters to control and validate the deserialization process. Ensure that only trusted classes are allowed to be deserialized and restrict the deserialization of sensitive classes.
Here's an example of implementing a serialization filter using ObjectInputFilter
:
ObjectInputFilter filter = ObjectInputFilter.Config.createFilter("com.example.TrustedClass", ObjectInputFilter.Config.Action.ALLOW);
ObjectInputStream input = new ObjectInputStream(new FileInputStream("data.bin"));
input.setObjectInputFilter(filter);
Object obj = input.readObject();
In this example, only the com.example.TrustedClass
is allowed to be deserialized, providing an additional layer of security against object injection attacks.
3. Monitor and Update Libraries
Regularly monitor for security updates and patches for the libraries and frameworks used in your Java application. Vulnerabilities in serialization libraries can expose your application to object injection attacks, so staying up to date with security patches is crucial.
The Closing Argument
Code injection attacks pose a significant threat to Java applications, but with proper preventive measures, they can be mitigated. By implementing input validation, using parameterized queries, escaping output, and taking precautions against object injection, you can significantly reduce the risk of code injection attacks in your Java applications.
Remember, security is an ongoing process, so it's essential to stay updated on the latest security best practices and vulnerabilities to keep your Java applications secure. By following these best practices, you can build robust and secure Java applications that are resilient to code injection attacks.
For further reading, you can explore the OWASP (Open Web Application Security Project) guide on Injection Attacks and the Java documentation on Input Validation. These resources provide additional insights into preventing code injection attacks and enhancing the security of your Java applications.