Maximizing Java Garbage Collection Efficiency
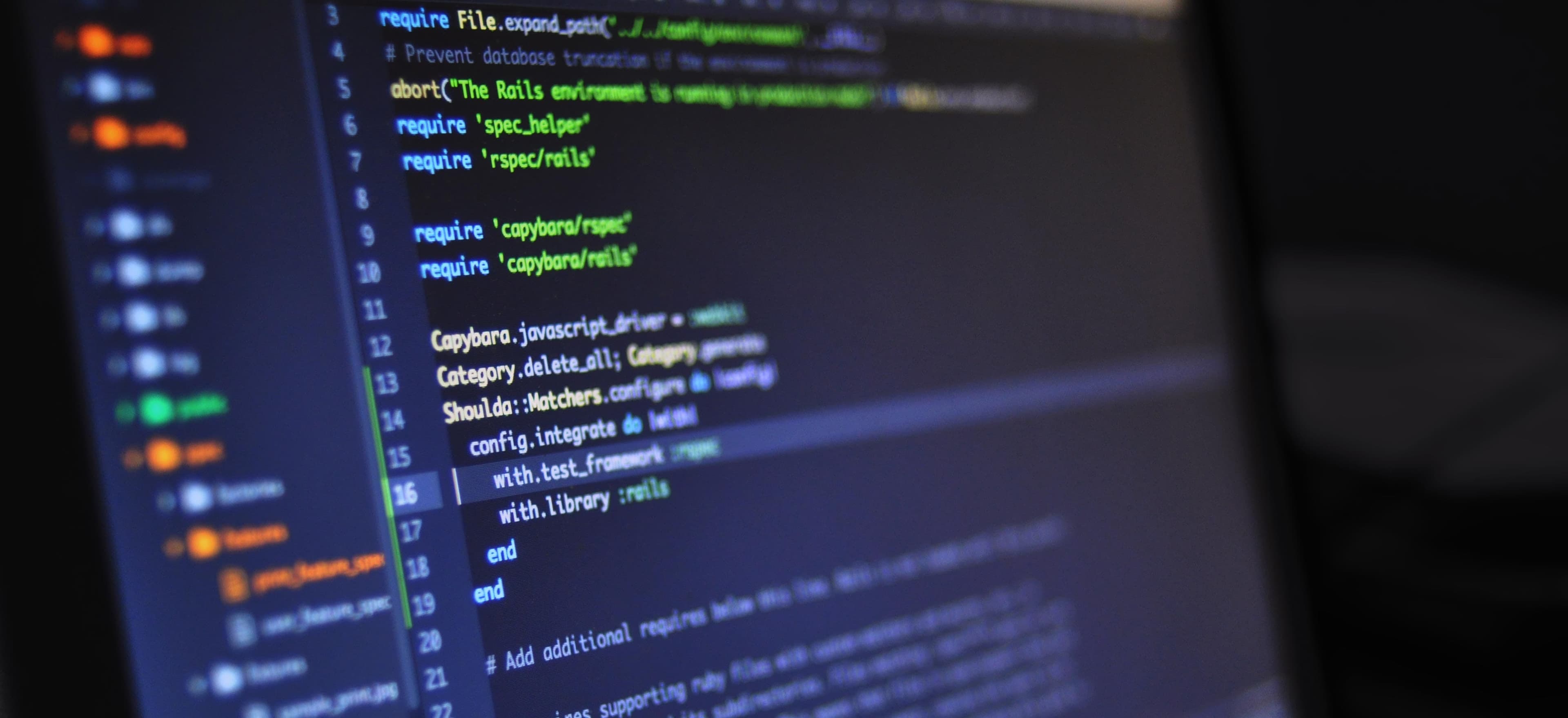
- Published on
In the world of Java development, efficient memory management is crucial for achieving optimal application performance. One of the key components of memory management in Java is garbage collection. Garbage collection plays a critical role in reclaiming memory occupied by objects that are no longer in use, preventing memory leaks and improving overall system stability. However, inefficient garbage collection can lead to performance issues such as increased latency and decreased throughput. In this blog post, we will explore strategies for maximizing garbage collection efficiency in Java.
Understanding Garbage Collection in Java
Before delving into optimization techniques, it is essential to have a solid understanding of how garbage collection works in Java. When a Java application creates objects, they are stored in the heap memory. Over time, some of these objects become unreachable or no longer in use. The garbage collector identifies these unreferenced objects and reclaims the memory they occupy, making it available for future allocations.
Java's garbage collector operates as part of the Java Virtual Machine (JVM) and comes in different flavors, such as the Serial Collector, Parallel Collector, CMS Collector, G1 Collector, and more. Each collector has its own strengths and weaknesses, and the choice of collector depends on factors like the size of the heap, the number of available CPU cores, and the application's memory allocation patterns. Understanding the characteristics of different garbage collectors is crucial when aiming to maximize garbage collection efficiency.
Minimizing Object Creation
One effective strategy for optimizing garbage collection is to minimize the creation of short-lived objects. Since short-lived objects tend to clutter the memory and trigger frequent garbage collection cycles, reducing their creation can have a significant impact on overall garbage collection efficiency.
Consider the following code snippet:
List<String> strings = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
strings.add("String " + i);
}
In this example, a large number of String objects are being created within the loop, contributing to increased memory consumption and potential garbage collection overhead. To minimize object creation, we can utilize the StringBuilder
class for string concatenation within the loop:
List<String> strings = new ArrayList<>();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
strings.add(sb.append("String ").append(i).toString());
sb.setLength(0);
}
By using StringBuilder
, we avoid unnecessary creation of intermediate String objects, thus reducing the burden on the garbage collector. This simple change can lead to improved garbage collection efficiency and overall application performance.
Tuning Garbage Collection Settings
Another crucial aspect of maximizing garbage collection efficiency is tuning the garbage collection settings to align with the specific requirements of the application. The Java Virtual Machine provides a range of command-line options that allow fine-tuning of garbage collection behavior, heap size, and other memory-related parameters.
For instance, the -Xms
and -Xmx
options control the initial and maximum heap size, while the -XX:NewSize
and -XX:MaxNewSize
options govern the size of the young generation space. By carefully adjusting these settings based on the application's memory usage patterns, developers can minimize excessive garbage collection pauses and improve memory management efficiency.
Utilizing Concurrent Mark Sweep (CMS) Collector
In scenarios where low-latency garbage collection is paramount, the Concurrent Mark Sweep (CMS) collector can be a valuable choice. The CMS collector is designed to minimize pause times by performing most of its work concurrently with the application threads.
To enable the CMS collector, the following command-line options can be used:
-XX:+UseConcMarkSweepGC -XX:+UseParNewGC
It's important to note that while the CMS collector excels in reducing pause times, it may not be as efficient in reclaiming space and compacting the heap compared to other collectors like the G1 collector. Careful consideration of the specific requirements and characteristics of the application is essential when selecting the appropriate garbage collection strategy.
Employing the G1 Garbage Collector
For modern Java applications running on Java 7 and later, the Garbage-First (G1) collector presents a compelling choice for achieving balanced performance and predictable garbage collection behavior. The G1 collector is designed to deliver high throughput while keeping pause times relatively low and predictable.
To enable the G1 collector, the following command-line option can be utilized:
-XX:+UseG1GC
The G1 collector organizes the heap into regions and uses a mix of parallel, concurrent, and parallel phases to achieve efficient garbage collection with minimal pause times. By leveraging G1, developers can benefit from improved garbage collection efficiency, especially in scenarios where low-latency and predictable pause times are critical requirements.
Implementing Object Pooling
Object pooling is a technique that involves reusing objects instead of creating new ones, thereby reducing the frequency of object allocation and subsequent garbage collection. In situations where objects are frequently created and discarded, object pooling can be a valuable optimization strategy.
One popular library for implementing object pooling in Java is Apache Commons Pool, which provides a generic object pooling framework. By reusing pooled objects, the application can minimize the impact of object creation on garbage collection, leading to improved memory management efficiency.
Monitoring Garbage Collection Performance
In the quest to maximize garbage collection efficiency, monitoring and analyzing garbage collection performance is essential. Tools such as VisualVM, Java Mission Control, and GCViewer can provide valuable insights into garbage collection behavior, heap utilization, and overall memory management.
By analyzing garbage collection logs, heap dumps, and utilization statistics, developers can identify potential bottlenecks, memory leaks, and areas for optimization. This data-driven approach empowers developers to make informed decisions when fine-tuning garbage collection settings and optimizing memory usage in Java applications.
The Last Word
In conclusion, achieving optimal garbage collection efficiency in Java requires a combination of understanding garbage collection fundamentals, implementing best practices for memory management, and leveraging the right garbage collection strategies based on the specific requirements of the application. By minimizing object creation, tuning garbage collection settings, selecting appropriate garbage collection algorithms, and employing object pooling, developers can maximize the efficiency of memory management and enhance the performance of Java applications.
In the ever-evolving landscape of Java development, the quest for efficient garbage collection remains a critical aspect of delivering high-performing and scalable applications. Continuous exploration of best practices, advanced optimization techniques, and utilization of modern garbage collection algorithms empowers developers to push the boundaries of memory management efficiency in Java.
By constantly refining garbage collection strategies and embracing innovative approaches, Java developers can unlock the full potential of memory management, thereby elevating the performance and resilience of their applications in today's demanding computing environments.