Optimizing Student Project Queries with JPA Criteria
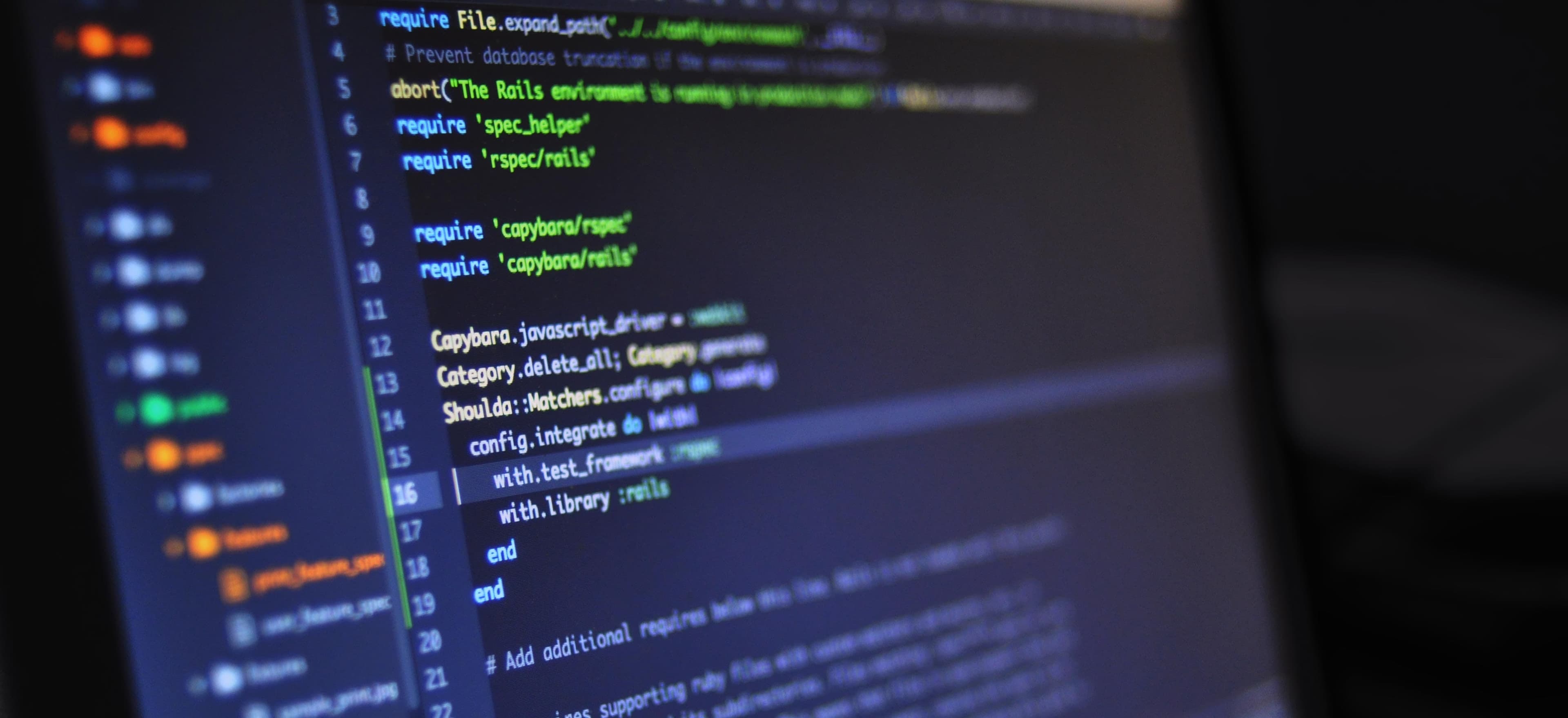
- Published on
Optimizing Student Project Queries with JPA Criteria
When working with a large database and complex queries, it's essential to optimize the queries to ensure efficient performance. In this article, we'll explore how to optimize queries for student projects using JPA Criteria in Java.
What is JPA Criteria?
JPA (Java Persistence API) Criteria is a standardized API for building dynamic queries in Java. It allows developers to create queries programmatically, which can be advantageous for building complex or dynamic queries at runtime. Using JPA Criteria can lead to more maintainable and readable code, as well as improved performance.
Examining the Problem
Let's consider a scenario where we have a database of students and their projects. We want to retrieve all the projects for a particular student, but we also want to filter the projects based on specific criteria, such as the project's status or completion date.
In a traditional approach, we might use JPQL (Java Persistence Query Language) to write the query. However, as the complexity of the query increases, maintaining and modifying the JPQL string becomes cumbersome. This is where JPA Criteria can come to the rescue.
Using JPA Criteria to Optimize Queries
We'll now delve into using JPA Criteria to optimize the queries for retrieving student projects based on various criteria. We'll showcase how JPA Criteria can offer a more flexible and manageable solution.
Setting Up the Entity Relationships
First, let's consider the entity relationships. We have Student
and Project
entities, where a student can have multiple projects. The Project
entity contains fields such as status
and completionDate
, which will be used for filtering projects.
Writing a Basic JPA Criteria Query
We can start by writing a basic JPA Criteria query to retrieve all projects for a specific student. The following code snippet demonstrates how to achieve this:
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Project> query = cb.createQuery(Project.class);
Root<Student> studentRoot = query.from(Student.class);
Join<Student, Project> projectJoin = studentRoot.join("projects");
query.select(projectJoin).where(cb.equal(studentRoot.get("id"), studentId));
List<Project> projects = entityManager.createQuery(query).getResultList();
In this code, we use the CriteriaBuilder
to create a criteria query for the Project
entity. We then join the Student
and Project
entities and specify the condition for the specific student's projects. Finally, we execute the query and retrieve the results.
Adding Conditions for Filtering Projects
Now, let's enhance the query to include conditions for filtering projects based on specific criteria, such as the project's status and completion date. We'll add predicates to the query to filter the projects based on these conditions.
List<Predicate> predicates = new ArrayList<>();
predicates.add(cb.equal(studentRoot.get("id"), studentId));
// Add condition to filter projects by status
if (status != null) {
predicates.add(cb.equal(projectJoin.get("status"), status));
}
// Add condition to filter projects by completion date
if (completionDate != null) {
predicates.add(cb.greaterThanOrEqualTo(projectJoin.get("completionDate"), completionDate));
}
query.select(projectJoin).where(predicates.toArray(new Predicate[0]));
List<Project> filteredProjects = entityManager.createQuery(query).getResultList();
In this enhanced code, we create a list of predicates to capture the conditions for filtering projects. We add predicates based on the status and completion date, and then apply these predicates to the query using the where
method.
Benefits of Using JPA Criteria for Optimization
Using JPA Criteria for optimizing queries offers several benefits:
-
Dynamic Query Building: JPA Criteria allows for the dynamic construction of queries based on runtime conditions, which leads to more flexible and adaptable query logic.
-
Type Safety: Since JPA Criteria queries are built using Java types and expressions, they are type-safe and less error-prone compared to JPQL strings.
-
Maintainability: JPA Criteria queries are more maintainable due to their programmatic nature, making it easier to modify and extend query logic.
In Conclusion, Here is What Matters
In this article, we explored the use of JPA Criteria to optimize queries for retrieving student projects based on various criteria. We demonstrated how JPA Criteria offers a more flexible and maintainable approach to building dynamic queries in Java, ultimately leading to improved query performance.
By leveraging JPA Criteria, developers can streamline the process of building and optimizing complex queries, resulting in more efficient interactions with the database.
For more in-depth understanding of JPA Criteria and its capabilities, refer to the official Java EE Documentation.
Optimizing queries with JPA Criteria is a powerful technique that can significantly enhance the performance of database interactions in Java applications.