Customizing AuthenticationProvider for Grails App Authentication
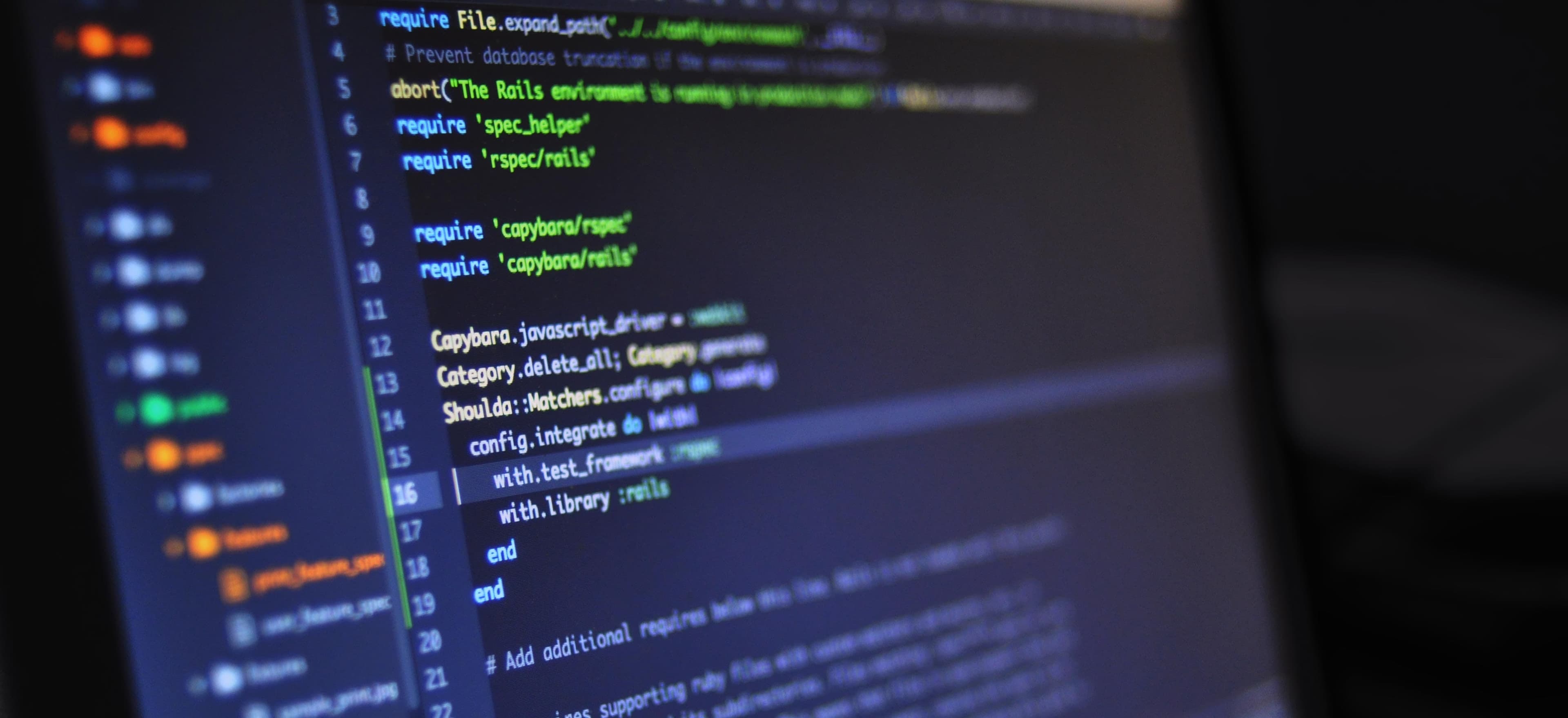
- Published on
Customizing AuthenticationProvider for Grails App Authentication
Authentication is a crucial part of any web application, especially when it comes to securing user data and resources. Grails, a powerful web development framework based on the Groovy language, offers various ways to handle authentication for your application. One common approach is to customize the AuthenticationProvider
for Grails app authentication. In this blog post, we'll explore the process of customizing the AuthenticationProvider
in a Grails application to provide a more flexible and tailored authentication mechanism.
Understanding AuthenticationProvider
In Grails, the AuthenticationProvider
interface is a key component of the security framework. It is responsible for authenticating users based on their credentials, such as username and password. By default, Grails uses the DaoAuthenticationProvider
, which relies on a data access object (DAO) to retrieve user details and perform authentication. However, in many cases, you may need to customize the authentication process to integrate with external systems, use alternative authentication methods, or apply specific business rules.
Creating a Custom AuthenticationProvider
To create a custom AuthenticationProvider
in a Grails application, you can follow these steps:
Step 1: Define the Custom AuthenticationProvider
Create a new Groovy class that implements the AuthenticationProvider
interface. This class will contain the logic for authenticating users based on your custom requirements. Let's create a simple example of a custom AuthenticationProvider
:
// File: CustomAuthenticationProvider.groovy
import org.springframework.security.authentication.AuthenticationProvider
import org.springframework.security.core.Authentication
import org.springframework.security.core.AuthenticationException
import org.springframework.security.core.userdetails.UserDetails
import org.springframework.security.core.userdetails.UsernameNotFoundException
import org.springframework.stereotype.Component
@Component
class CustomAuthenticationProvider implements AuthenticationProvider {
@Override
boolean supports(Class<?> authentication) {
// Define the authentication mechanism supported by this provider
return UsernamePasswordAuthenticationToken.isAssignableFrom(authentication)
}
@Override
Authentication authenticate(Authentication authentication) throws AuthenticationException {
// Implement the authentication logic based on your requirements
String username = authentication.name
String password = authentication.credentials.toString()
// Perform custom authentication logic, such as calling an external service, checking against a different data source, etc.
// Replace this logic with your specific authentication requirements
// ...
// If authentication is successful, return a fully populated Authentication object
// Otherwise, throw AuthenticationException
}
}
In this example, we've created a CustomAuthenticationProvider
class that implements the supports
method to specify the authentication mechanism it supports and the authenticate
method to define the authentication logic.
Step 2: Configure the Custom AuthenticationProvider
Once the custom AuthenticationProvider
class is defined, you need to configure it in the Grails application's security settings. This can be achieved by modifying the grails-app/conf/spring/resources.groovy
file to include the bean definition for the custom AuthenticationProvider
:
// File: resources.groovy
beans = {
customAuthenticationProvider(CustomAuthenticationProvider)
}
By defining the bean for the custom AuthenticationProvider
, you are instructing the Grails application to use this provider for authentication.
Step 3: Integration and Testing
After creating and configuring the custom AuthenticationProvider
, it's crucial to integrate it with the rest of the application's security setup, such as user role management and access control. Additionally, thorough testing of the custom authentication logic is essential to ensure its reliability and security.
Benefits of Custom AuthenticationProvider
Customizing the AuthenticationProvider
in a Grails application offers several benefits, including:
- Flexibility: You can tailor the authentication process to meet specific business requirements or integrate with external systems and APIs.
- Enhanced Security: By implementing custom authentication logic, you can implement additional security measures beyond the standard username and password verification.
- Scalability: Custom authentication providers can easily accommodate future enhancements and integrations as the application evolves.
Key Takeaways
In this blog post, we've delved into the process of customizing the AuthenticationProvider
for Grails app authentication. By creating a custom AuthenticationProvider
class, configuring it in the application, and integrating it with the existing security setup, you can ensure a more tailored and flexible authentication mechanism for your Grails application. Customizing the AuthenticationProvider
offers the flexibility, security, and scalability required for modern web application authentication.
Would you like to delve deeper into Grails application security or explore advanced authentication strategies? Drop your thoughts in the comments below and let's continue the conversation!