Optimizing Business Rules with Drools Executable Model
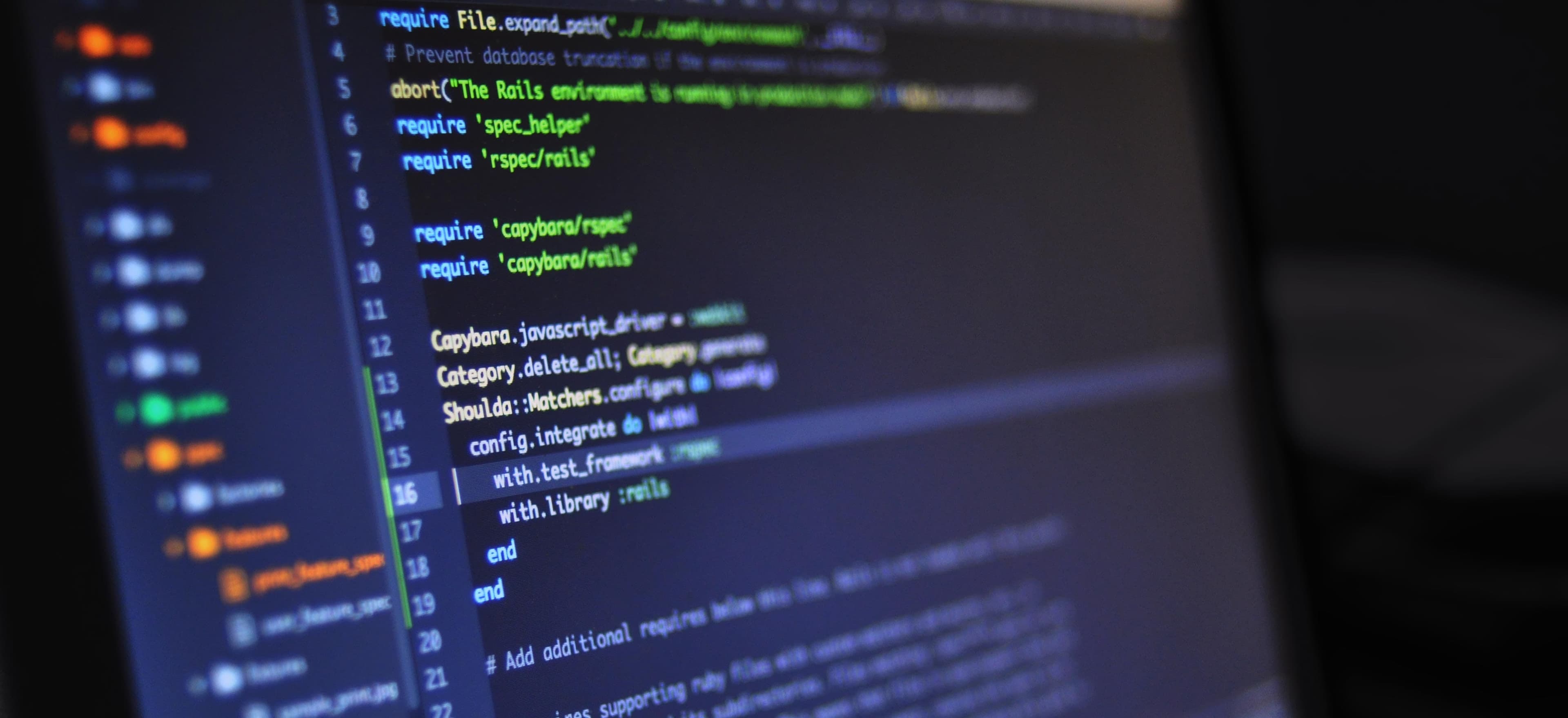
- Published on
Optimizing Business Rules with Drools Executable Model
In the realm of software development, incorporating business rules seamlessly into an application can be a challenging task. Traditional approaches often lead to rigid, difficult-to-maintain code that is tightly coupled with the application. However, with the emergence of rule engines like Drools, developers can now effectively externalize business rules from the application logic, enabling greater flexibility, maintainability, and agility.
In this article, we'll explore how to leverage the Drools executable model to optimize business rules within a Java application. We'll delve into the features and benefits of the Drools executable model and provide practical examples to demonstrate its usage and advantages.
Understanding the Drools Executable Model
The Drools executable model is a relatively new feature introduced in Drools 7. It represents a significant paradigm shift in the way business rules are defined, executed, and deployed. Unlike the traditional Drools rule engine, which parses rules at runtime and maintains a working memory alongside the application, the executable model allows rules to be compiled into a standalone, self-contained artifact that can be executed independently.
This new approach offers several noteworthy benefits, including improved performance, reduced memory footprint, and enhanced interoperability with other systems. By leveraging the executable model, developers can encapsulate business rules in a format that is optimized for efficient execution, making it an ideal choice for scenarios requiring high throughput and low latency.
Getting Started with Drools Executable Model
To begin harnessing the power of the Drools executable model, let's consider a simple use case where we need to validate customer orders based on a set of business rules. We'll walk through the process of defining, compiling, and executing these rules using the executable model.
Setting Up the Project
First, we need to create a new Maven project and add the necessary dependencies for using Drools. We can do this by including the following dependencies in the project's pom.xml
file:
<dependencies>
<dependency>
<groupId>org.drools</groupId>
<artifactId>drools-core</artifactId>
<version>7.59.0.Final</version>
</dependency>
<dependency>
<groupId>org.kie</groupId>
<artifactId>kie-api</artifactId>
<version>7.59.0.Final</version>
</dependency>
</dependencies>
Defining Business Rules
Next, let's define the business rules using the Drools rule language. For our example, we'll create a simple rule to validate customer orders based on their total amount.
package com.example.rules;
rule "Validate Order Total"
when
$order : Order(total > 100)
then
$order.setValid(true);
end
In this rule, we specify that if the total amount of the order is greater than 100, the order is considered valid.
Compiling Rules with the Executable Model
With the rules defined, we can now compile them into an executable model using the Drools compiler. The following Java code demonstrates this process:
import org.drools.modelcompiler.builder.KieBaseBuilder;
public class RuleCompiler {
public static void main(String[] args) {
KieBaseBuilder.createKieBaseFromModel("com.example.rules");
}
}
By invoking KieBaseBuilder.createKieBaseFromModel
, the rules defined in the specified package (com.example.rules
) will be compiled into an executable model.
Executing Business Rules
Once the rules have been compiled, we can proceed to execute them against customer orders within our application. The following snippet illustrates how to apply the compiled rules to validate a customer order:
import org.kie.api.KieServices;
import org.kie.api.runtime.KieContainer;
public class OrderValidator {
public boolean validateOrder(Order order) {
KieServices kieServices = KieServices.Factory.get();
KieContainer kieContainer = kieServices.newKieClasspathContainer();
KieSession kieSession = kieContainer.newKieSession();
kieSession.insert(order);
kieSession.fireAllRules();
kieSession.dispose();
return order.isValid();
}
}
In this code, we obtain an instance of KieServices
and create a KieContainer
representing the compiled executable model. We then instantiate a KieSession
to apply the rules to the customer order. After firing the rules, we dispose of the session and return the validation result.
Advantages of the Drools Executable Model
The Drools executable model offers several compelling advantages for optimizing business rules within a Java application:
-
Performance: By pre-compiling rules into an executable model, the overhead of runtime parsing and rule evaluation is significantly reduced, resulting in improved performance.
-
Memory Efficiency: The standalone nature of the executable model minimizes the memory footprint, particularly in comparison to traditional Drools applications that maintain a working memory alongside the application.
-
Interoperability: The executable model can be easily packaged and deployed as a standalone artifact, facilitating seamless integration with other systems and services.
-
Static Analysis: Due to the compilation process, the executable model allows for static analysis of rules, enabling early detection of potential issues and enhanced rule validation.
Key Takeaways
In this tutorial, we've explored the Drools executable model and its role in optimizing business rules within a Java application. By leveraging the executable model, developers can achieve significant performance improvements, reduced memory footprint, and enhanced interoperability when incorporating business rules into their applications.
The transition to the Drools executable model represents a fundamental evolution in the way business rules are handled, offering a modern approach that aligns with the demands of contemporary software development. As organizations continue to prioritize agility, efficiency, and maintainability, the executable model presents a compelling solution for effectively managing and executing business rules in a scalable and performant manner.
As you embark on your journey with the Drools executable model, consider exploring further resources, such as the official Drools documentation and the Drools user guide, to deepen your understanding and expand your capabilities in optimizing business rules within your Java applications. Embrace the power of the Drools executable model to unlock new possibilities for rule management and execution in your software solutions.