Using Content Security Policy Nonce with Spring Security
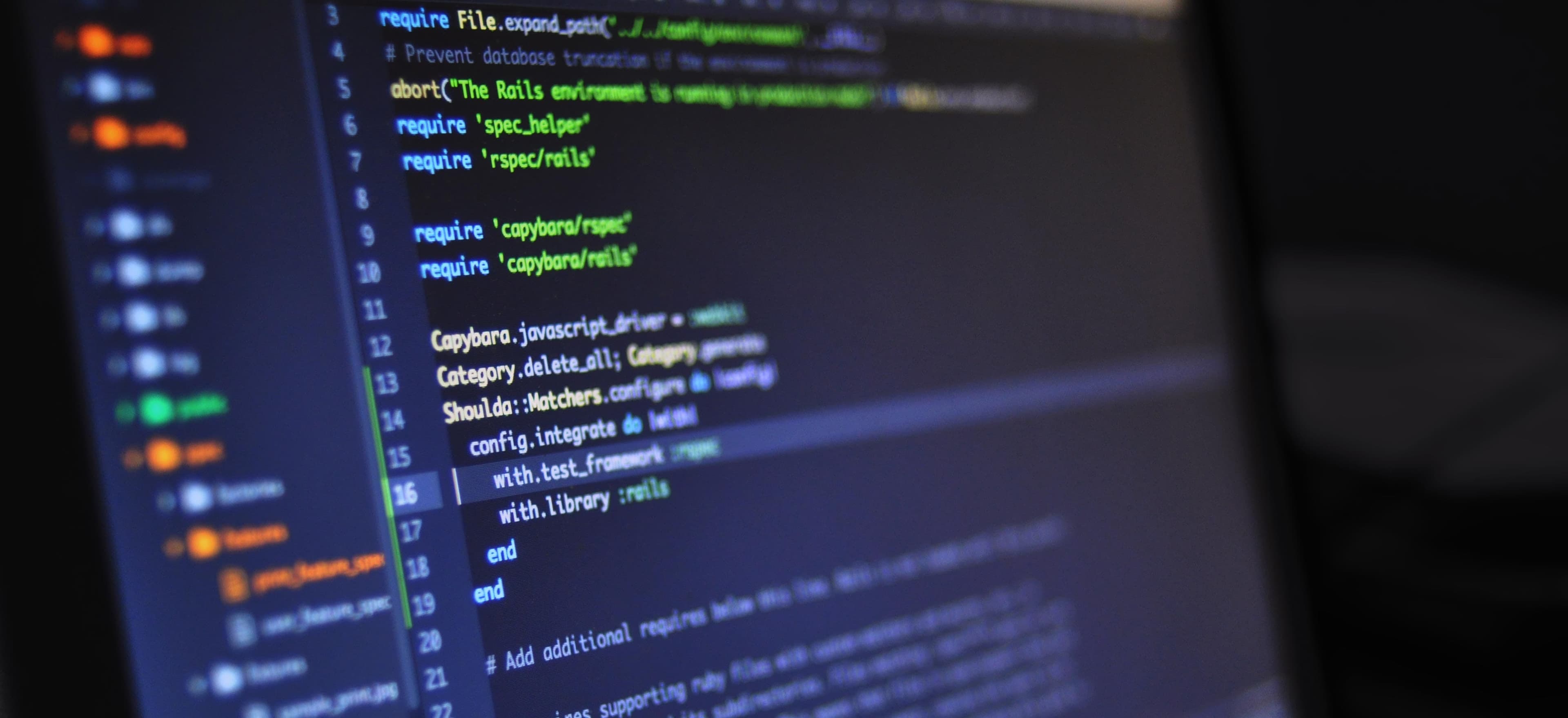
- Published on
Title: Enhancing Security with Content Security Policy Nonce in Spring Security
In today’s digital world, web application security is a critical consideration for any enterprise. As Java developers, we often rely on Spring Security to fortify our applications. However, one area that is sometimes overlooked is Content Security Policy (CSP). CSP is a vital layer of defense against cross-site scripting (XSS) attacks. In this blog post, we will delve into the concept of CSP nonce and how to integrate it with Spring Security to bolster the security posture of our Java web applications.
Understanding Content Security Policy (CSP) and Nonce
Content Security Policy (CSP) is a security standard that helps prevent various types of attacks, such as XSS. CSP works by allowing developers to define the sources from which certain types of content can be loaded on their websites. This includes scripts, stylesheets, fonts, and other resources.
One important feature of CSP is the nonce (number used once). A nonce is a random value generated on each page load and included in the CSP header. In conjunction with CSP, the nonce allows only scripts with a matching nonce to be executed, thereby thwarting XSS attacks.
Integrating CSP Nonce with Spring Security
When it comes to integrating CSP nonce with Spring Security, we need to make some adjustments to both the server-side and client-side code.
Server-Side Configuration
We start by configuring Spring Security to generate and include the CSP nonce in the response headers. To achieve this, let’s consider a simple Spring Security configuration:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// Other configurations
.and()
.headers()
.contentSecurityPolicy("script-src 'self' 'nonce-{nonce}'");
// Additional configurations
}
}
In the above configuration, we’ve added the contentSecurityPolicy
method to the headers section and specified script-src 'self' 'nonce-{nonce}'
. This includes the nonce
placeholder, which will be replaced with the actual nonce value.
Generating Nonce in the Controller
Next, we need to generate the nonce value and include it in the response headers. We can achieve this by creating a filter or interceptor to insert the nonce value into the CSP header. Here’s an example using a filter:
@Component
public class NonceFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
response.setHeader("Content-Security-Policy", "script-src 'self' 'nonce-" + generateNonce() + "'");
filterChain.doFilter(request, response);
}
private String generateNonce() {
// Add logic to generate a random nonce
return "random-nonce-value";
}
}
In this code, we create a NonceFilter
that adds the CSP header with the generated nonce value to every response.
Client-Side Usage
On the client side, when including scripts in the HTML, we need to add the nonce
attribute to the script tag, ensuring that it matches the nonce received in the CSP header. Here’s an example:
<script nonce="random-nonce-value" src="example.js"></script>
This script tag specifies the nonce value using the nonce
attribute, ensuring that it matches the value included in the CSP header from the server. This way, only scripts with matching nonces will be executed.
Closing Remarks
In conclusion, integrating Content Security Policy (CSP) nonce with Spring Security is a powerful strategy to enhance the security of Java web applications. By properly configuring Spring Security to include CSP nonce in response headers and ensuring client-side scripts match the nonce, developers can effectively mitigate the risk of XSS attacks.
Remember, security is an ongoing process, and staying informed about emerging threats and best practices is crucial for safeguarding our applications and the sensitive data they handle.
By incorporating CSP nonce with Spring Security, we reinforce our commitment to building robust and secure Java web applications.
Do you have experience with Content Security Policy or implementing security measures in Spring Security? Share your thoughts and experiences in the comments below!
For further reading, check out the following resources: