Optimizing Data Processing with Java 9 Streams
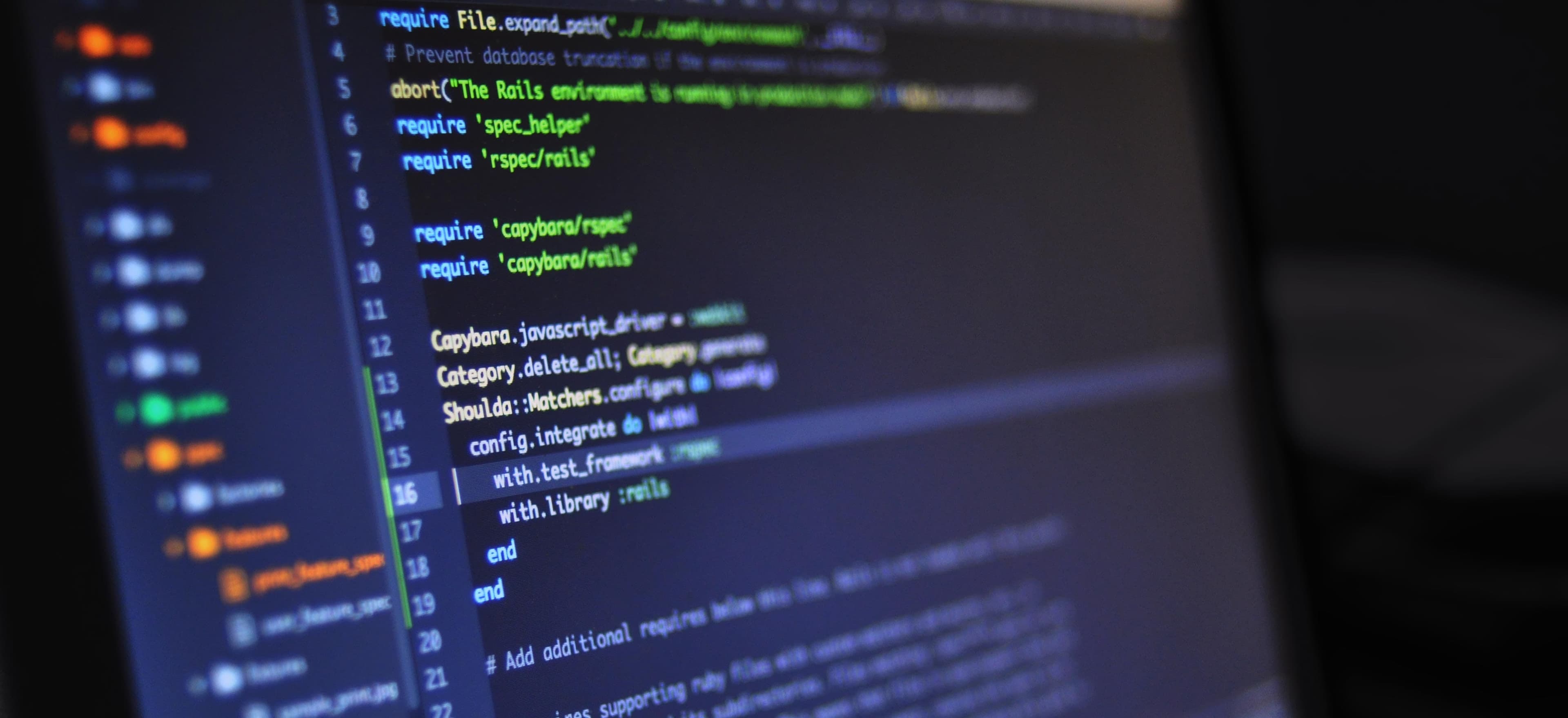
- Published on
Optimizing Data Processing with Java 9 Streams
When it comes to data processing in Java, efficiency and readability are crucial. With the introduction of Java 8, the Streams API revolutionized the way we handle collections and perform operations on them. Java 9 further enhanced this capability with additional features and optimizations.
In this article, we'll dive into the world of Java 9 Streams, exploring how they can significantly optimize data processing tasks. We'll discuss the benefits of using Streams, explore the new features introduced in Java 9, and provide practical examples to demonstrate their usage for data processing.
Understanding the Power of Streams
Java Streams provide a declarative and functional approach to processing collections. They enable developers to express complex data processing operations concisely and clearly. By leveraging the power of Streams, developers can write clean, readable code while benefiting from efficient and optimized data processing.
One of the key advantages of Streams is their ability to facilitate parallel processing. With parallel Streams, the processing of elements in a collection can be automatically distributed across multiple threads, harnessing the full power of multi-core processors and improving performance for large datasets.
What's New in Java 9 Streams?
Java 9 introduced several enhancements to the Streams API, empowering developers with additional features to streamline data processing tasks. Some of the notable additions include:
Stream::takeWhile and Stream::dropWhile
The takeWhile
and dropWhile
methods allow developers to take or drop elements from a Stream based on a condition. This provides more flexibility and control when processing elements in a Stream, enabling efficient filtering operations.
Optional::stream
The Optional::stream
method allows easy transformation of Optional
objects into Streams. This simplifies the integration of Optional values into Stream-based processing, making the code more expressive and concise.
Stream::iterate
The Stream::iterate
method now supports a predicate to define when the iteration should stop. This enhancement allows for more dynamic and flexible stream generation, catering to a wider range of use cases.
Practical Examples
Let's explore some practical examples to demonstrate the power of Java 9 Streams in optimizing data processing tasks.
Example 1: Filtering Data with takeWhile and dropWhile
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> result1 = numbers.stream()
.takeWhile(n -> n < 5)
.collect(Collectors.toList());
// Output: [1, 2, 3, 4]
List<Integer> result2 = numbers.stream()
.dropWhile(n -> n < 5)
.collect(Collectors.toList());
// Output: [5, 6, 7, 8, 9, 10]
In this example, the takeWhile
method is used to take elements from the stream while the condition (n < 5
) is met. Conversely, the dropWhile
method drops elements from the stream until the condition is no longer satisfied.
Example 2: Converting Optional to Stream
Optional<String> value = Optional.of("Hello, World!");
List<String> result = value.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
// Output: ["HELLO, WORLD!"]
In this example, the Optional::stream
method is used to transform the Optional
value into a Stream, enabling seamless integration with Stream operations such as mapping and collection.
Example 3: Generating a Dynamic Stream with iterate
Stream.iterate(1, n -> n < 100, n -> n * 2)
.forEach(System.out::println);
// Output: 1 2 4 8 16 32 64
In this example, the Stream::iterate
method is used to generate a dynamic stream of integers, applying a predicate to determine when the iteration should stop and a function to define the next element in the sequence.
The Last Word
Java 9 Streams offer a powerful way to optimize data processing tasks, providing enhanced capabilities and performance improvements over traditional collection processing methods. By leveraging the new features introduced in Java 9, developers can write more expressive and efficient code for handling collections and stream-based operations.
Incorporating Java 9 Streams into your projects can lead to improved readability, better performance, and increased productivity. So, next time you find yourself working with collections in Java, consider harnessing the power of Streams to optimize your data processing tasks.
To delve deeper into Java 9 Streams and their capabilities, check out the official Java 9 documentation and explore additional resources for advanced Stream usage.
Remember, mastering Java 9 Streams can unlock a whole new level of efficiency and elegance in your data processing workflows. Happy coding!